Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial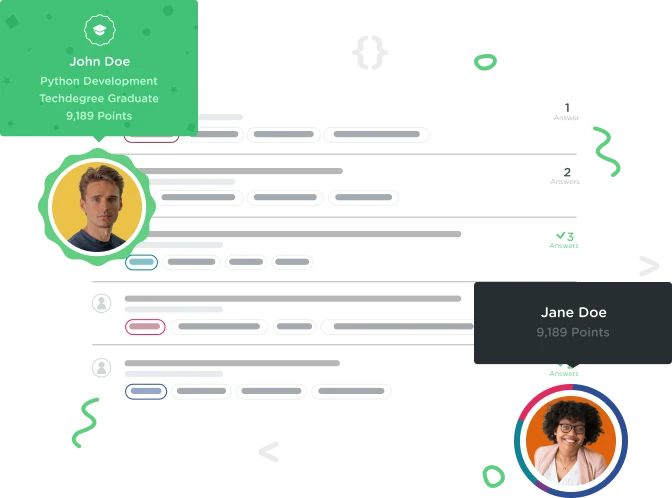

dfeem
4,660 PointsMy list doesn't function appropriately. I've watched video over and over, but can't figure out where I messed up?
The following is what I ran in the REPL, but it doesn't function as it's supposed to. It functions a little, but not how it does in video. Please give any insight on what I did wrong?
import os
shopping_list = []
def clear_screen(): os.system(cls if os.name == "nt" else "clear")
def show_help(): clear_screen() print("This list is for you to list whatever:") print("""
- Enter 'DONE' to stop adding items.
- Enter 'HELP' for help using this app.
- Enter 'SHOW' to show items in list. """)
def add_to_list(item): show_list() if len(shopping_list): position = input("Where should I add {}?\n" "Press 'ENTER' to add to end of the list\n" "> ".format(item)) else: position = 0
try:
position = abs(int(position))
except ValueError:
position = None
if position is not None:
shopping_list.insert(position-1, item)
else:
shopping_list.append(new_item)
def show_list(): clear_screen()
print("* Here's your list:")
index = 1
for item in shopping_list:
print("{}. {}".format(index, item))
index += 1
print("-"*10)
show_help()
while True: new_item = input("> ")
if new_item.upper() == 'DONE' or new_item.upper() == 'QUIT':
break
elif new_item.upper() == 'HELP':
show_help()
continue
elif new_item.upper == 'SHOW':
show_list()
continue
else:
add_to_list(new_item)
show_list()

dfeem
4,660 PointsSure, The, 'DONE', 'SHOW' and 'HELP' prompts don't work as they do in video. To the best of my knowledge, all the functionality code that I wrote is identical to the code in video.
Thanks!

nico teuben
2,509 PointsCan you post the entire file ?

dfeem
4,660 PointsYep, I sure can. I did so with my initial question, but for some reason it came out weirdly.
import os
shopping_list = []
def clear_screen(): os.system(cls if os.name == "nt" else "clear")
def show_help(): clear_screen() print("This list is for you to list whatever:") print("""
- Enter 'DONE' to stop adding items.
- Enter 'HELP' for help using this app.
- Enter 'SHOW' to show items in list. """)
def add_to_list(item): show_list() if len(shopping_list): position = input("Where should I add {}?\n" "Press 'ENTER' to add to end of the list\n" "> ".format(item)) else: position = 0
try:
position = abs(int(position))
except ValueError:
position = None
if position is not None:
shopping_list.insert(position-1, item)
else:
shopping_list.append(new_item)
def show_list(): clear_screen()
print("* Here's your list:")
index = 1
for item in shopping_list:
print("{}. {}".format(index, item))
index += 1
print("-"*10)
show_help()
while True: new_item = input("> ")
if new_item.upper() == 'DONE' or new_item.upper() == 'QUIT':
break
elif new_item.upper() == 'HELP':
show_help()
continue
elif new_item.upper == 'SHOW':
show_list()
continue
else:
add_to_list(new_item)
show_list()

dfeem
4,660 PointsIt came out the same way. I don't know why.
4 Answers
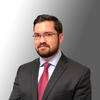
Brennan White
11,646 PointsYou can reference the Markdown Cheatsheet to see why what you are posting is coming out weird. You use three backpacks to start and end code sections.
I reformatted your code and tested it out. Looks like you were running a clear_screen() in show_help(). Take that out and everything works.
import os
shopping_list = []
def clear_screen():
os.system("cls" if os.name == "nt" else "clear")
def show_help():
#clear_screen()
print("This list is for you to list whatever:")
print("""
Enter 'DONE' to stop adding items.
Enter 'HELP' for help using this app.
Enter 'SHOW' to show items in list. """)
def add_to_list(item):
show_list()
if len(shopping_list):
position = input("Where should I add {}?\n" "Press 'ENTER' to add to end of the list\n" "> ".format(item))
else:
position = 0
try:
position = abs(int(position))
except ValueError:
position = None
if position is not None:
shopping_list.insert(position-1, item)
else:
shopping_list.append(new_item)
def show_list():
clear_screen()
print("* Here's your list:")
index = 1
for item in shopping_list:
print("{}. {}".format(index, item))
index += 1
print("-"*10)
show_help()
while True:
new_item = input("> ")
if (new_item.upper() == 'DONE') or (new_item.upper() == 'QUIT'):
break
elif new_item.upper() == 'HELP':
show_help()
continue
elif new_item.upper == 'SHOW':
show_list()
continue
else:
add_to_list(new_item)
show_list()

dfeem
4,660 Pointstry: position = abs(int(position)) except ValueError: position = None if position is not None: shopping_list.insert(position-1, item) else: shopping_list.append(new_item)
I found the problem. I forgot to put 'show_list()' right here. Once I did, everything worked well.
show_list()

dfeem
4,660 PointsI took that out 'clear screen' out, but it still doesn't function correctly. I'll mess around with it some more.
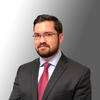
Brennan White
11,646 PointsDid you use the code that I posted? It seemed to be working fine to me.

Lawrence Maripfonde
8,102 PointsYeah you are right @Brennan, I guess need to rectify that line of code. Thank you very much.

Lawrence Maripfonde
8,102 PointsThe error is on indexing. Try this line of code instead of insert(position-1, item)
if position is not None:
shopping_list.insert(-1, item)
else:
shopping_list.append(new_item)
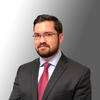
Brennan White
11,646 PointsYour approach will place the item at the end rather than in a desired position. You are merely appending two different ways.

dfeem
4,660 Pointsif position is not None: shopping_list.insert(position-1, item) else: shopping_list.append(new_item)
Brennan White
11,646 PointsBrennan White
11,646 PointsHey can you be more specific about what is not working properly?