Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial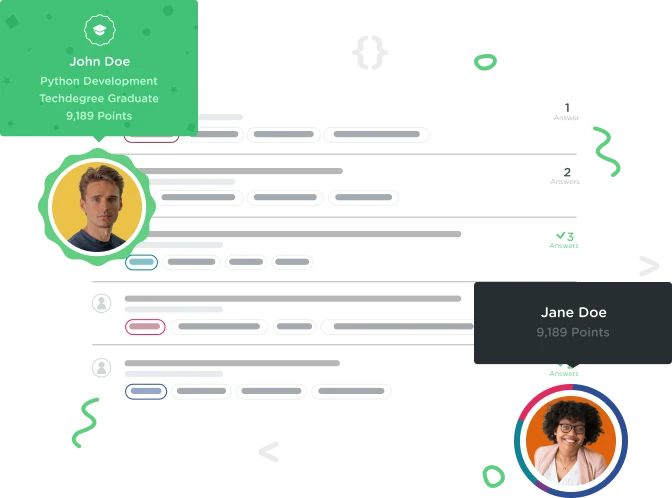
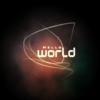
Kristian Terziev
28,449 PointsMy logic seems to be a bit off regarding the byArtist method. Would appreciate some help.
Hi :) So first off, here's the method:
private Map<String, List<Song>> byArtist() {
Map<String, List<Song>> byArtist = new HashMap<>();
for (Song song : mSongs) {
List<Song> artistSongs = byArtist.get(song.getArtist());
if (artistSongs == null) {
artistSongs = new ArrayList<>();
byArtist.put(song.getArtist(), artistSongs);
}
artistSongs.add(song);
}
return byArtist;
}
So the thing I can't quite understand is how we add the songs in the map. Here's how my logic works:
Let's say we have 2 songs in our mSongs list:
- MJ - They Don't Care About Us
- MJ - Beat It
So we enter the method and a NEW map is created. (meaning it's all "null" inside it, right?). So the map looks like this:
null -> null
We take "They Don't Care About Us" and enter the scope of the foreach. We assign a new list (artistSongs) with whatever is the value for key MJ in the map (that would be null). Meaning we enter the "if" scope. Here we assign that list (currently null) to a brand new ArrayList (again null? (correct me if I'm wrong)). Then we put inside the map key "MJ" and value "null". So the map looks like this:
MJ -> null;
Finally we add the song to the list(so it has only one element) Now we take "Beat It". We assign it to whatever is the value for key MJ (currently that would be null). Therefore, we enter the if again. The list is assigned to a new one (meaning it's back to "null") and in the map, once again, is being put MJ -> null. Then "Beat It" is being add to the list and we exit the method by returning the map which is MJ -> null. So we basically have no songs in the map.
I know my logic is off somewhere but I just can't find that place. I went through the method at least 10 times with different songs by different artists and I still got the same result. Would appreciate some clarification on the whole process. Thank you :)
EDIT: Craig Dennis, I would love some input
4 Answers

Craig Dennis
Treehouse TeacherHi Kristian!
I was on PTO, sorry about the delay.
It's easiest if you think about these object references. And yes it is really just pointers when it all boils down.
So artistsSongs gets initialized with either an existing list or null
if it doesn't exist. If it doesn't exist, we make a new one, and put it in the map so that the next time through it will be there. Because the list is just an object reference, we either have the existing one or the new one, and we add our new song to it.
Sounds like you got it ;)
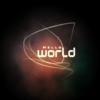
Kristian Terziev
28,449 PointsAll right. After doing a little research I was enlightened in the concept of pointers from C++ so now I understand why the code works. If anyone is facing the same issue and needs help, feel free to ask. I'm not sure I'll explain it perfectly but I will do my best

James Reynolds
3,325 PointsI was struggling with this as well until I remembered pointers from C. I am kind of curious though as to what would happen if this object was created out of scope, such as a private member variable. What stops the data in reference from being over-written?

Thomas Williams
9,447 PointsJust trying to get to grips with this so would appreciate if someone could clarify or rectify my current understanding of lines 6-9... as i understand it...an ArrayList gets created called artistSongs, we then tie this empty list to a specific key/artist with the byArtist.put() method, then finally we add a song to that list. On the next time we run through the loop with a different artist, we create another ArrayList also called artistSongs but because we then put it into the Map using a different key/artist it can be called up separately from the first 'artistSongs' ArrayList we created before, hence, for the artistsSongs.add(song) method it is adding the song only to the artistSongs ArrayList that has been called up/created in the preceding 'for each' loop as opposed to there being just one ArrayList called artistSongs that either keeps getting bigger or overwritten?
Luis Flores
10,499 PointsLuis Flores
10,499 PointsThis helped me understand it!! Thanks Kristian Terziev