Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial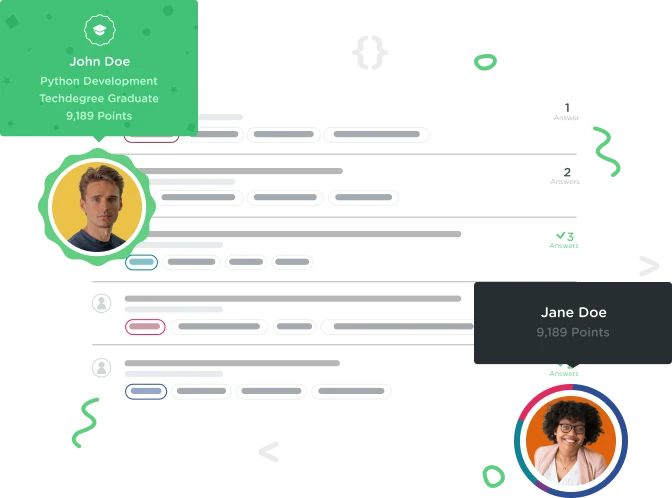

Terrell Stewart
Courses Plus Student 4,228 PointsMy logout button wont appear once user is authenticated
Within my layout.html I have a if statement that checks to see if the user is logged in, If so the logout button will appear and the register and login buttons will not show. Also if the user is not logged in the login and register button will show. My problem here is that the statement isn't picking up is the user is authenticated and just moving straight to the else statement why is this happening?
from flask import (Flask,g,render_template,flash,redirect,url_for,abort)
from flask.ext.bcrypt import check_password_hash
from flask.ext.login import (LoginManager,login_user,logout_user,
current_user,login_required)
import models
import forms
DEBUG = True
PORT = 8000
HOST = '0.0.0.0'
app = Flask(__name__)
app.secret_key = 'rfhrfuwn3ofi39-fh'
login_manager = LoginManager()
login_manager.init_app(app)
login_manager.login_view = 'login'
@login_manager.user_loader
def load_user(user_id):
try:
models.User.get(models.User.id==user_id)
except models.DoesNotExist:
return None
@app.before_request
def before_request():
g.db = models.DATABASE
g.db.connect()
g.user = current_user
@app.after_request
def after_request(response):
g.db.close()
return response
@app.route('/register',methods=('GET','POST'))
def register():
form = forms.Registration()
if form.validate_on_submit():
flash('You just registered!!!',"success")
models.User.create_user(
email = form.email.data,
password = form.password.data
)
return redirect(url_for('index'))
return render_template('register.html',form=form)
@app.route('/login', methods=('GET', 'POST'))
def login():
form = forms.LoginForm()
if form.validate_on_submit():
try:
user = models.User.select().where(
models.User.email**form.email.data
).get()
if check_password_hash(user.password, form.password.data):
login_user(user)
flash("You're now logged in!")
return redirect(url_for('index'))
else:
flash("Email or password is invalid")
except models.DoesNotExist:
flash("Email or password is invalid")
return render_template('login.html', form=form)
@app.route('/logout')
@login_required
def logout():
logout_user()
flash("You've been logged out!","success")
return redirect(url_for('index'))
@app.route('/')
def index():
tacos = models.Taco.select()
return render_template('index.html',tacos=tacos)
if __name__== '__main__':
models.initialize()
app.run(debug=DEBUG,port=PORT,host=HOST)
<!doctype html> <html> <head> <title>Tacocat</title> <link rel="stylesheet" href="/static/css/normalize.css"> <link rel="stylesheet" href="/static/css/skeleton.css"> <link rel="stylesheet" href="/static/css/tacocat.css"> </head> <body> {% with messages=get_flashed_messages() %} {% if messages %} <div class="messages"> {% for message in messages %} <div class="message"> {{ message }} </div> {% endfor %} </div> {% endif %} {% endwith %}
<div class="container">
<div class="row">
<div class="u-full-width">
<nav class="menu">
{% if current_user.is_authenticated() %}
<a href="{{ url_for('logout') }}" class="icon-power" title="Log out">Logout</a>
{% else %}
<a href="{{ url_for('login') }}" class="icon-power" title="Log in">Login</a>
<a href="{{ url_for('register') }}" class="icon-profile" title="Register">Register</a>
{% endif %}
<!-- menu goes here -->
</nav>
{% block content %}{% endblock %}
</div>
</div>
<footer>
<p>An MVP web app made with Flask on <a href="http://teamtreehouse.com">Treehouse</a>.</p>
</footer>
</div>
</body> </html>
1 Answer
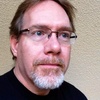
Chris Freeman
Treehouse Moderator 68,457 PointsHey Terrell, depending on your environment, the parens after is_authenticated()
may or may not be needed. It was different between my local testing and workspaces. Try removing the parens in your template usage.
This guidance is mentioned at the bottom of a Teacher's Note but it's easy to overlook.
Add a comment if this doesn't work.