Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial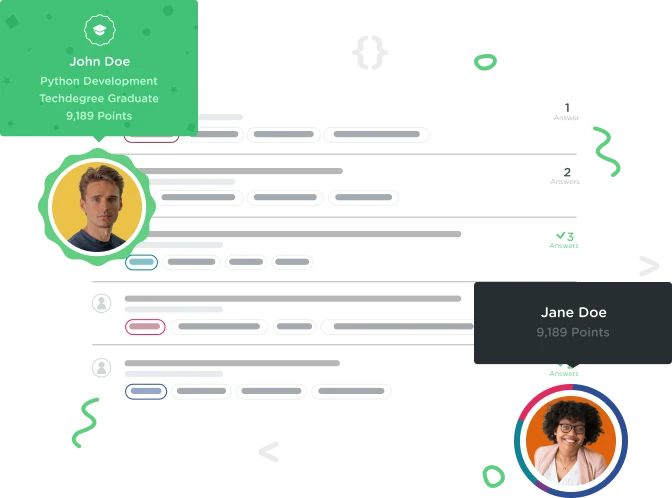

DAVID WILSON
1,243 Pointsmy loop doesn't break
hello, this game works fine except when i enter the right number it doesn't break and print you won.
import random goal_num = random.randint(1,10) allowed_guesses = 5 used_numbers = [] def show_help(): print("\nguess a number from 1 to 10") print("\nyou can have 5 tries") print("\nenter EXIT to exit") show_help() while len(used_numbers) < 5: a = input("what's your number ? ") if a == goal_num: print("you won") break elif a != goal_num: used_numbers.append(a) print("try again") continue else: print("you lost, number was {}".format(goal_num))
2 Answers

Keerthi Suria Kumar Arumugam
4,585 PointsAll inputs in Python are strings. Convert it to a number before you compare with goal_num.
And your else: block will never run. So, consider putting it somewhere else so that the user knows he has run out of turns
import random
goal_num = random.randint(1,10)
print(goal_num)
allowed_guesses = 5
used_numbers = []
def show_help():
print("\nguess a number from 1 to 10")
print("\nyou can have 5 tries")
print("\nenter EXIT to exit")
show_help()
while len(used_numbers) < 5:
a = int(input("what's your number ? "))
if a == goal_num:
print("you won")
break
elif a != goal_num:
used_numbers.append(a)
print("try again")
continue
else:
print("you lost, number was {}".format(goal_num))

Frederick Pearce
10,677 PointsAgree with Keerthi's answer, you need to convert the result from input into an integer for comparison with goal_num.
As for the proper place to print "you won", one could use an infinite while loop and put the print within another "if, elif" block that is embedded in the "elif a ~= goal_num" block, as shown below. It's also a good idea to use a variable reference where possible, instead of coding in hard numbers that actually depend on that variable (e.g. use "allowed_guesses", as "5" is only right when allowed_guesses = 5, etc.)
import random
goal_num = random.randint(1,10)
print(goal_num)
allowed_guesses = 5
used_numbers = []
def show_help():
print("\nguess a number from 1 to 10")
print("\nyou can have 5 tries")
print("\nenter EXIT to exit")
show_help()
while True:
a = int(input("what's your number ? "))
if a == goal_num:
print("you won")
break
elif a != goal_num:
if len(used_numbers) == allowed_guesses - 1:
# This was your last guess, so no need to store the number?
# Instead, print "you lost", and break
print("you lost, number was {}".format(goal_num))
break
else:
# otherwise append guess and keep going
used_numbers.append(a)
print("try again")
continue
Frederick Pearce
10,677 PointsFrederick Pearce
10,677 PointsKeerthi, can you tell me how you get such nice formatting for the code portion of your replies? Thanks!
Keerthi Suria Kumar Arumugam
4,585 PointsKeerthi Suria Kumar Arumugam
4,585 PointsHi Fred,
From your answer below, I guess you have already enclosed your code in two sets of three backticks(`).
Include the word Python after the first set of backticks so that the formatter knows what kind of code you are adding
Frederick Pearce
10,677 PointsFrederick Pearce
10,677 PointsThank you for the help, Keerthi!