Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial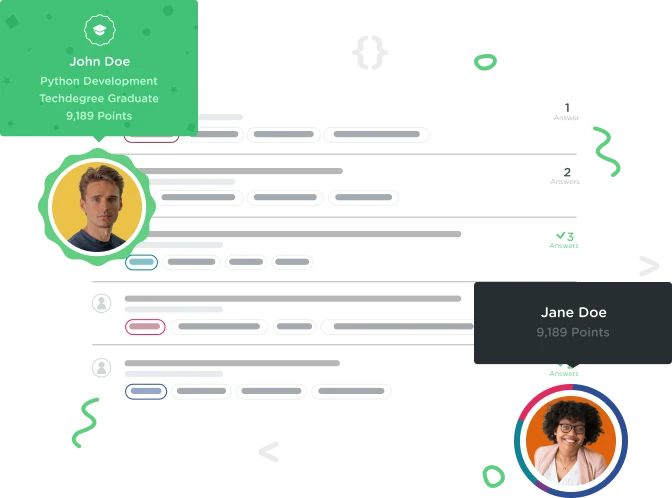

sanjeshwari Nand
Full Stack JavaScript Techdegree Student 4,673 Pointsmy name/input value keeps disappearing after i edit, please help
// write a function that //creates a list item element (name) and add to the DOM then takes the text we want the list item to contain and returns that list item back to us //wrap the text created inside the li in a span tag so that the text becomes an html element, currently it's just a text element and we cannot do anything with it
function createName (text) {
const name = document.createElement('LI');
const span = document.createElement('span');
span.textContent = text;
name.appendChild(span);
const label = document.createElement('LABEL');
label.textContent = 'confirmed';
const checkBox = document.createElement('INPUT');
checkBox.type = 'checkbox';
label.appendChild(checkBox);
name.appendChild(label);
const editBtn = document.createElement('button');
editBtn.textContent = 'edit';
editBtn.className = 'edit'
name.appendChild(editBtn);
const removeBtn = document.createElement('button');
removeBtn.className = 'remove';
removeBtn.textContent = 'remove';
name.appendChild(removeBtn);
return name;
};
//listen for the click handler submit button //get the value of the text in the input box //create li and add the value to the Ul via the li
//set the click handler on the form instead of the submit button. The form has a submit 'event' that listens to clicks on the form so the form can be submitted multiple ways ie //via clicking on the submit button or ANYWHERE on the form.
const form = document.getElementById('registrar'); const ulList = document.getElementById('invitedList');
const names = form.querySelector('input');
form.addEventListener('submit', (e) => {
e.preventDefault();
const text = names.value;
names.value = '';
const name = createName(text);
ulList.appendChild(name)
});
// add a handler to the checkbox, when the checkbox is checked add a class of responded (already in CSS) //instead of add the event handler to each checkbox when clicked(this causes bulk and is not preffered) we should use event bubbling (the act of an event bubbling up the DOM tree setting off any and all events until it reaches the root element, bubbling will continue up weather the next element has an event handler attached or not and will simply keep going till it reaches any event handlers.The parent node, in this case the Ul is the best place to attach the event handler (the click event) for the checkbox because the event on the Ul will listen out for the right event ie click on checkbox then it will find the source of the event and will perform the callback function on that source.So it doesn't matter where the click takes place, only the right target will be handled due to the event object target property.
ulList.addEventListener('change', (e) => {
if(e.target.checked) {
e.target.parentNode.parentNode.className = 'responded';
}else{
e.target.parentNode.parentNode.className = ''
}
});
// add a remove button to each invitee to be able to remove li item from the Ul
ulList.addEventListener('click', (e) => {
//the click event can be triggered by clicking on any element so we must emphasise the target element that should trigger the click event
//Edit list items once saved
//create edit button, add event handler that removes the text element (name) and adds an input element // save button that has event handler, that removes the input element and adds the text element
const li = e.target.parentNode;
const ul = li.parentNode;
if (e.target.className === 'remove') {
ul.removeChild(li);
} else if (e.target.className === 'edit') {
const span = li.firstElementChild;
const newInput = document.createElement('input');
newInput.type = 'text';
newInput.value = span.textContent;
li.insertBefore(newInput, span);
li.removeChild(span);
e.target.textContent = 'save';
}
});
2 Answers
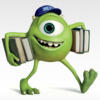
Tobias Edwards
14,458 PointsAt the moment, once you click edit
and enter the new name, clicking save
afterwards acts the same as clicking the edit
button again. Why? Let's break it down:
-
edit
button with class nameedit
is clicked. This event bubbles up toulList
and is caught by your event handler. - The button clicked has a class name of
edit
, and so the appropriate code is executed:- Inserting
newInput
and changing thetextContent
of the edit button tosave
only. Only the text value of this button is changed, so its class is STILLedit
.
- Inserting
- Entering a new name into
newInput
and then clickingsave
(actually the 'edit' button) will trigger step 1 again.
On clicking save
, the reason the new input field is cleared is because before you were setting its value to span.textContent
which was removed: li.removeChild(span)
.

sanjeshwari Nand
Full Stack JavaScript Techdegree Student 4,673 PointsThank you Tobias! that made sense! i was able to fix it!