Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial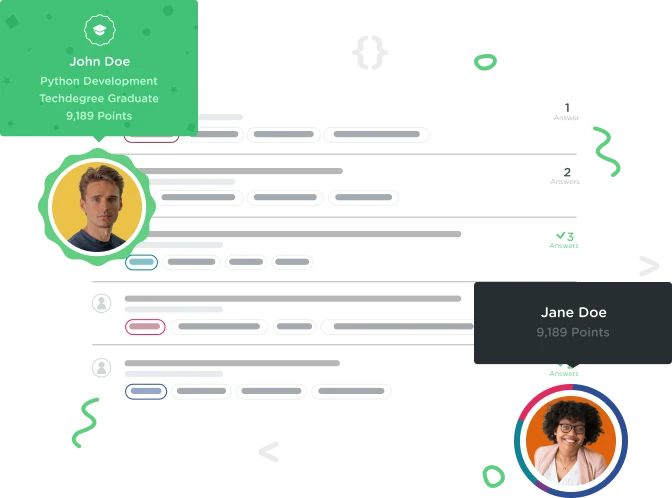

Jose Sanchez
7,849 PointsMy next and stop button not working, Song selections stay pink even after hitting next and stop.
Here is all my JavaScript code, its most likely just a typo
// sets properties for the songs
function Song(title, artist, duration) {
this.title = title;
this.artist = artist;
this.duration = duration;
// this is to monitor whether the song is on or off
this.isPlaying = false;
}
Song.prototype.play = function() {
// this will start playing the song
this.isPlaying = true;
};
Song.prototype.stop = function() {
// this will make the song stop playing
this.isPLaying = false;
};
Song.prototype.toHTML = function() {
//create the HTML that will be displayed
var htmlString = '<li';
if(this.isPlaying){
htmlString += ' class="current"'
}
htmlString += '>';
htmlString += this.title;
htmlString += ' - ';
htmlString += this.artist;
htmlString += '<span class="duration">';
htmlString += this.duration;
htmlString += '</span></li>'
return htmlString;
};
function Playlist() {
// create an empty array of songs
this.songs = [];
this.nowPlayingIndex = 0;
}
// push songs into empty songs array []
Playlist.prototype.add = function(song) {
this.songs.push(song);
};
Playlist.prototype.play = function() {
// access current song by using nowPlayingIndex
var currentSong = this.songs[this.nowPlayingIndex];
currentSong.play();
};
Playlist.prototype.stop = function(){
// access current song by using nowPlayingIndex
var currentSong = this.songs[this.nowPlayingIndex];
currentSong.stop();
};
Playlist.prototype.next = function(){
// stops current song that is playing
this.stop();
// increment the index to move the playlist to the next song
this.nowPlayingIndex++;
// ensure that nowPlayingIndex count doesnt go over the the amount of songs availble
if(this.nowPlayingIndex === this.songs.length){
this.nowPlayingIndex = 0;
}
this.play();
};
Playlist.prototype.renderInElement = function(list) {
list.innerHTML = "";
for(var i = 0; i < this.songs.length; i++){
// .innerHTML allows us to insert it into the HTML
list.innerHTML += this.songs[i].toHTML();
}
};
var playlist = new Playlist();
var allTheWay = new Song("All the way", "Time flies ", "2:54");
var iChooseYou = new Song("I choose you", "Time flies ", "3:43");
playlist.add(allTheWay);
playlist.add(iChooseYou);
// Getting ordered HTML list
var playlistElement = document.getElementById("playlist");
// pass playlistElement into renderInElement
playlist.renderInElement(playlistElement);
// implement a click element on buttons
var playButton = document.getElementById("play");
playButton.onclick = function(){
playlist.play();
// since playlist.play() changes the state of the objects, we need to refresh the user interface by passing in the renderInElement
playlist.renderInElement(playlistElement);
}
var nextButton = document.getElementById("next");
nextButton.onclick = function(){
playlist.next();
playlist.renderInElement(playlistElement);
}
var stopButton = document.getElementById("stop");
stopButton.onclick = function(){
playlist.stop();
playlist.renderInElement(playlistElement);
}
1 Answer

jared eiseman
29,023 PointsSong.prototype.stop = function() {
// this will make the song stop playing
this.isPLaying = false;
};
You have a typo in the "this.isPlaying = false" the L is capitalized.
Jose Sanchez
7,849 PointsJose Sanchez
7,849 PointsI swear I looked at that line at least 10 time but not once did I see that... Thank you for the help !
jared eiseman
29,023 Pointsjared eiseman
29,023 PointsNo problem, glad to help. :)