Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial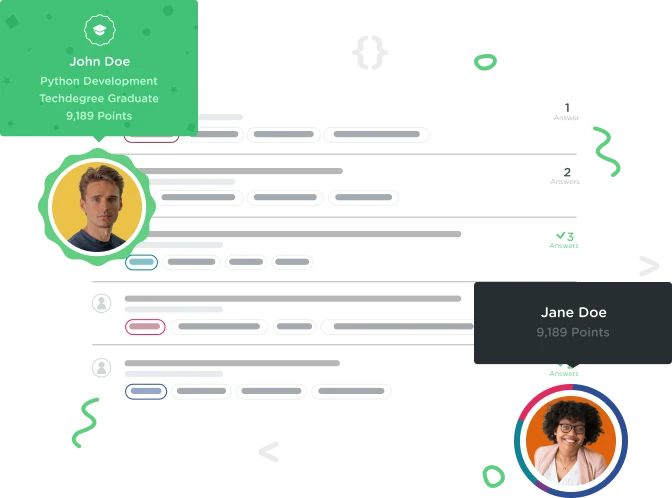

Thomas Moore
5,301 PointsMy own code not working
I made my own code for this challenge and it works until it comes to printing out to the page. I think it has something to do with my html variable, but I'm not sure.
var quiz = [
['How many stars are on the American Flag?' , 50],
['How many capitals per state?' , 1],
['How many legs does a dog have?' , 4]
];
var right= 0;
var correct = [];
var wrong = [];
var answer;
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
function buildList(arr) {
var listHTML = '<ol>';
for (i = 0; i < arr.length; i +=1) {
listHTML + '<li>' +arr[i] + '</li>';
}
listHTML +='</ol>';
return listHTML;
}
for (i=0; i<quiz.length; i+=1) {
answer = parseInt(prompt(quiz[i][0]));
if (answer === quiz[i][1] ) {
right += 1;
correct += quiz[i][0];
} else {
wrong += quiz[i][0];
}
}
html = 'Congratulations you got ' + right + ' question(s) right!';
html += '<h2>You got these questions correct:</h2>';
html += buildList(correct);
html += '<h2>You got these questions wrong:</h2>';
html += buildList(wrong);
print(html);
console.log(correct);
console.log(wrong);
The questions answered correct and the questions answered wrong do print out to the console log, but don't print out to the order list. I know that I will need to format the list better, because as of now it's only going to be one list item. Still I imagine they should print the the correct and wrong variables, even if it's a bunch of strings mashed together?
Thanks in advance.
2 Answers
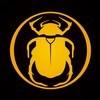
rydavim
18,814 PointsI found two small problems, but the rest looks good. Nice job!
function buildList(arr) {
var listHTML = '<ol>';
for (i = 0; i < arr.length; i +=1) {
listHTML += '<li>' + arr[i] + '</li>'; // you were missing an equals sign here
}
listHTML +='</ol>';
return listHTML;
}
for (i=0; i<quiz.length; i+=1) {
answer = parseInt(prompt(quiz[i][0]));
if (answer === quiz[i][1] ) {
right += 1;
correct.push(quiz[i][0]); // you can't concatenate to add to an array, use push
} else {
wrong.push(quiz[i][0]); // you can't concatenate to add to an array, use push
}
}
Those two changes got it working for me. :)

Hayes McCardell II
643 PointsAre you using the Chrome Debugging tools? (F12)
What does the console log say when you run it?
Thomas Moore
5,301 PointsThomas Moore
5,301 PointsBah an equals sign! Should have caught it. Thanks a lot!