Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial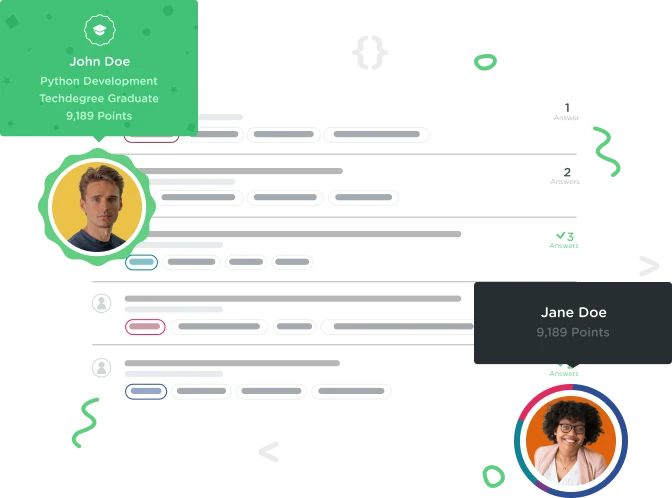

David Scanu
20,160 PointsMy own Facebook Events to Wordpress Plugin
I am trying to write my own wordpress plugin that would import public events into my wordpress site.
My plugin will :
register a custom post type "events" and add meta boxes (custom fields) to it
connect to the Graph API and get an object or array containing all the events posted by a public facebook page. [https://developers.facebook.com/docs/graph-api/reference/v2.2/page/events]
use that object/array to populate my custom posts "Events" with all the informations : name, start time, description, event cover, etc...
Here is the PHP code i use to connect to the Graph API ad return an array containing my "events" :
<?php
$myplugin_app_id = 'YOUR APP ID HERE';
$myplugin_secret = 'YOUR APP SECRET HERE';
$myplugin_page_id = 'PUBLIC PAGE ID YOU WANT TO GET EVENTS FROM';
// Skip these two lines if you're using Composer
// define('FACEBOOK_SDK_V4_SRC_DIR', '/facebook-php-sdk-v4/src/Facebook/');
try {
require __DIR__ . '/facebook-php-sdk-v4/autoload.php';
} catch (Exception $e) {
exit('Require failed! Error: '.$e);
}
use Facebook\FacebookSession;
use Facebook\FacebookRequest;
use Facebook\GraphUser;
use Facebook\FacebookRequestException;
use Facebook\GraphObject;
FacebookSession::setDefaultApplication( $myplugin_app_id, $myplugin_secret);
// If you're making app-level requests:
$session = FacebookSession::newAppSession();
// If you already have a valid access token:
// $session = new FacebookSession( $pp_app_token );
// To validate the session:
try {
$session->validate();
} catch (FacebookRequestException $ex) {
// Session not valid, Graph API returned an exception with the reason.
echo $ex->getMessage();
} catch (\Exception $ex) {
// Graph API returned info, but it may mismatch the current app or have expired.
echo $ex->getMessage();
}
/* PHP SDK v4.0.0 */
/* make the API call */
$request = new FacebookRequest(
$session,
'GET',
// get all events of a Page, ?fields= let you choose which fields you want the Graph to return
'/' . $myplugin_page_id . '/events?fields=id,name,location,description,start_time'
);
$response = $request->execute();
$graphObject = $response->getGraphObject()->asArray();
/* handle the result */
$objectData = $graphObject['data']; // returns an indexed array without key
?>
This code fully works and you can use it to make your own Graph API connections. $objectData is an indexed array without key. $objectData[0] is an "event", so the name of the event will be $objectData[0]->{'name'};
The next part is where I am having difficulties :
How do I trigger WP to create a post for each event in the array ?
How do I populate the custom fields with the values in the array ?
How and where do I store the values in the database ?
2 Answers

Zac Gordon
Treehouse Guest TeacherHey David!
Two things come to mind:
- Check out wp_instert_post. It will create a new post for you and then return the post ID. You can then use update_post_meta to update the custom field value. This will still work even if you haven't assigned a custom field value for the post yet.
- Double check with FB that you are allowed to save data from FB on your own site. Not sure that you can't, but something that came to mind to make sure about.
Hope that helps!

David Scanu
20,160 PointsThanks a lot Zac.
wp_insert_post and update_post_meta are the function I needed to make this work.
I'll check to see if I am allowed to do it.