Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial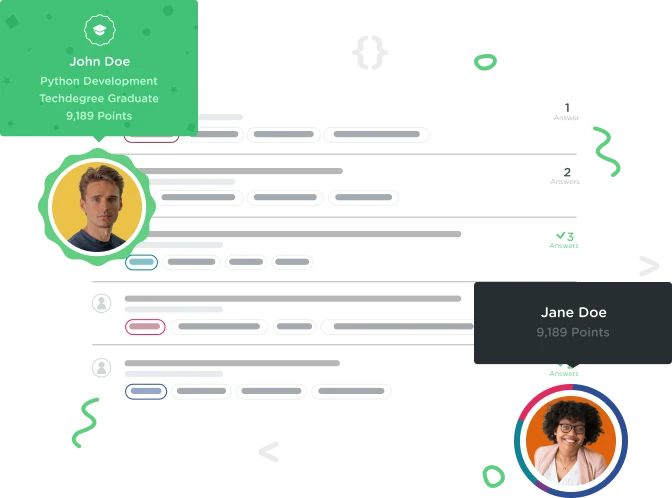
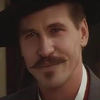
huckleberry
14,636 PointsMy pre-solution-video attempt: I tried something a little different than what Dave initially asked for. Thoughts?
Hola fellow JavaScripter neophyte types and our enthusiastic overlord Dave McFarland
As expected of us I gave it a full on shot before the solution video and here's what I got...
Two things
- I didn't listen entirely to the instructions (I have a tendency to dive in when I just get "the jist"... sometimes it's good, sometimes not so much) so I wound up doing it a different way than Dave suggested
- I tried to incorporate various things in there including multiple functions as well as the <code>.push()</code> method.
Here it is...
//Build a quiz challenge part 1
//Specs: Build a simple quiz program.
//will ask a series of questions and evaluate each answer.
//The program should keep track of the number of questions answered correctly.
//After the quiz is done, the program should display the number of questions that were correctly answered as well as the number of questions the player got wrong.
//ESTABLISH THE ARRAY
var quiz = [
["How many states does the U.S. have?",
"What is 7/2?",
"A common breed of dog is 'Donkey'. True or False?",
"What is the NJ state bird?",
"What is the most widely used VCS?"],
["50",
"3.5",
"false",
"goldfinch",
"git"],
//correct answers get pushed to this array
[],
//incorrect answers get pushed to this array
[]
];
//FUNCTION FOR BUILDING THE PRINTOUT
function answerTrue(answer){
var correctList = "<h1>Correct</h1><br><ol>"
for (var i = 0; i < quiz[2].length; i++){
correctList += "<li>" + answer[i] + "</li>"
}
correctList += "</ol>"
print(correctList);
};
function answerFalse(answer){
var incorrectList = "<h1>You should be ashamed</h1><br><ol>"
for (var i = 0; i < quiz[3].length; i++){
incorrectList += "<li>" + answer[i] + "</li>"
}
incorrectList += "</ol>"
print(incorrectList);
};
//FUNCTION FOR PRINTING OUT FINAL RESULTS
function print(result){
document.write(result);
}
//INITIATING GAME
var letsPlay = prompt("Would you like to take a quick quiz to see how smart you are?");
letsPlay = letsPlay.toLowerCase();
if (letsPlay === 'yes'){
for (var i = 0; i < quiz[0].length; i++){
var answer = prompt(quiz[0][i]);
if (answer === quiz[1][i]){
quiz[2].push("You answered question " + (i + 1) + " correctly with: " + answer + "!");
} else if(answer != quiz[1][i]){
quiz[3].push("You answered question " + (i + 1) + " incorrectly with: " + answer + ". The correct answer was " + quiz[1][i] + ".")
}
}
}
if (letsPlay === 'yes'){
//Function call to print out correct answers
answerTrue(quiz[2]);
//Function call to print out incorrect answers
answerFalse(quiz[3]);
}
else {
alert("Take care, dummy");
}
My thinking was that by keeping the questions and answers separate on their own and then actually storing the resulting answers in different secondary arrays that it would make everything more accessible if I wanted to do something else with it in the long run.
It works fantastically and while I haven't watched the next vid yet I'm off to do that now so any advice on how I could've made it simpler or more compact or whatever, please share!!
Thanks all!
Cheers,
Huck -
1 Answer

Nicholas Jensen
5,969 PointsI like the idea of keeping the arrays separate, but I think the point of the lesson was to use the slightly more complicated question -> answer array as practice. That said there was one part of your code I thought could use a little DRY refresh. Overall it works very well.
I changed:
//FUNCTION FOR BUILDING THE PRINTOUT
function answerTrue(answer){
var correctList = "<h1>Correct</h1><br><ol>"
for (var i = 0; i < quiz[2].length; i++){
correctList += "<li>" + answer[i] + "</li>"
}
correctList += "</ol>"
print(correctList);
};
function answerFalse(answer){
var incorrectList = "<h1>You should be ashamed</h1><br><ol>"
for (var i = 0; i < quiz[3].length; i++){
incorrectList += "<li>" + answer[i] + "</li>"
}
incorrectList += "</ol>"
print(incorrectList);
};
To follow more DRY practice:
function printAnswer(heading, index, answer){
var list="<h1>"+heading+"</h1><br><ol>";
for (var i = 0; i < quiz[2].length; i++){
list += "<li>" + answer[i] + "</li>";
}
list += "</ol>";
print(list);
}
function answerTrue(answer){
printAnswer('Correct', 2, answer);
}
function answerFalse(answer){
printAnswer('You should be ashamed!', 3, answer);
}
I also added .toLowerCase() to your answer prompt
var answer = prompt(quiz[0][i]).toLowerCase();
And also got rid of this:
}
if (letsPlay === 'yes'){
right before answerTrue() because it was redundant. The top close brace closes the exact same if that you start again.
There appears to be a bunch of semi colons missing, but it compiles fine because of close braces. I would like to make the push answers in the middle of the quiz a bit more DRY friendly, but nothing sprang to mind. Hope I don't sound too picky, just trying to push you further. -Nick
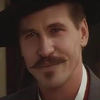
huckleberry
14,636 Pointsnoooo not too picky at all. This is exactly the kind of stuff I'm looking for! Can't improve without critique from those more advanced than I who have experience and know how to do things more efficiently and more cleanly.
I have to put all your stuff together and then look side by side as I'm still at the "wait what?" stage and not at the "oh right right, but of course" stage lol.
But thank you so much!
Cheers,
Huck -
Chyno Deluxe
16,936 PointsChyno Deluxe
16,936 PointsWorked for me. Good Job.