Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial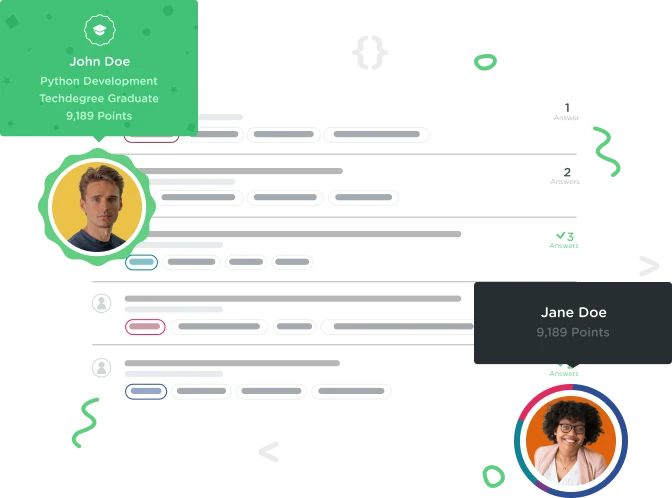

Mamta Chaudhary
527 PointsMy Program is not outputting my if statement.
I am experiencing 2 problems with my program: 1.) My program is not allowing the user to enter their Full Name for the signature, it just ends the code. 2.) My program is not outputting the guest information which is dependent on if the signature matches the name variable.
package Motel; import java.util.Scanner; import java.util.Date;
class Main {
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
Guest Identification = new Guest();
Date date = new Date();
System.out.println(date);
System.out.println("Welcome to the Motel");
System.out.println("Please answer the following Questions to Check In: ");
System.out.println("Enter your Full Name: ");
String name = input.nextLine();
Identification.setGuestName(name);
System.out.println("Enter your Address: ");
String address = input.nextLine();
Identification.setGuestAddress(address);
System.out.println("Date of Birth: ");
String dateOfBirth = input.nextLine();
Identification.setGuestDateOfBirth(dateOfBirth);
System.out.println("Enter your Identification Card Number or SSC number: ");
int identificationNumber = input.nextInt();
Identification.setGuestIdentificationNumber(identificationNumber);
System.out.println("Enter your Full Name for a E-Signature: ");
String signature = input.nextLine();
Identification.setGuestSignature(signature);
System.out.println(signature);
if (signature.equals(name)) {
System.out.println("Please Make sure this information is Correct: ");
System.out.printf(" Name: %s %n", Identification.getGuestName());
System.out.printf(" Address: %s %n", Identification.getGuestAddress());
System.out.printf(" D.O.B: %d %n", Identification.getGuestDateOfBirth());
System.out.printf(" ID#: %d %n", Identification.getGuestIdentificationNumber());
System.out.printf(" E Signature: ", Identification.getGuestSignature());
}
}
}
package Motel; import java.io.Serializable; public class Guest implements Serializable {
private String guestName;
private String guestAddress;
private String guestSignature;
private String guestDateOfBirth;
private int guestIdentificationNumber;
public void Guest (String name, String address, String signature,
String dateOfBirth, int identificationNumber) {
this.guestName = name;
this.guestAddress = address;
this.guestDateOfBirth = dateOfBirth;
this.guestIdentificationNumber = identificationNumber;
this.guestSignature = signature;
}
public String getGuestName () {
return this.guestName;
}
public void setGuestName (String name){
this.guestName = name;
}
public String getGuestAddress () {
return guestAddress;
}
public void setGuestAddress (String address){
this.guestAddress = address;
}
public String getGuestSignature () {
return guestSignature;
}
public void setGuestSignature (String signature){
this.guestSignature = signature;
}
public String getGuestDateOfBirth () {
return guestDateOfBirth;
}
public void setGuestDateOfBirth ( String dateOfBirth){
this.guestDateOfBirth = dateOfBirth;
}
public int getGuestIdentificationNumber () {
return guestIdentificationNumber;
}
public void setGuestIdentificationNumber ( int iD){
this.guestIdentificationNumber = iD;
}
my output
Wed Jan 02 20:32:31 EST 2019 Welcome to the Motel Please answer the following Questions to Check In: Enter your Full Name: Full name Enter your Address: 123 Glenwood Ave Date of Birth: 01/16/99 Enter your Identification Card Number or SSC number: 123456789 Enter your Full Name for a E-Signature:
Process finished with exit code 0
//it is not letting me enter the signature.
1 Answer

Unsubscribed User
1,321 PointsHello Mamta Chaudhary,
I figured out your problem.
the input.nextInt() does not read the newline character in your input created by hitting "Enter," and so the call to input.nextLine() returns a "\n". Basically an automatic blank entry.
You will encounter the similar behavior when you use input.nextLine() after input.next() (except nextLine itself).
Workaround:
- Put an input.nextLine(); call after each Scanner.nextInt to consume the rest of that line including the newline.
System.out.println("Enter your Identification Card Number or SSC number: ");
int identificationNumber = input.nextInt();
Identification.setGuestIdentificationNumber(identificationNumber);
input.nextLine(); // This will clear the buffer and allow you to enter the signature.
System.out.println("Enter your Full Name for a E-Signature: ");
String signature = input.nextLine();
Identification.setGuestSignature(signature);
System.out.println(signature);
Unsubscribed User
1,321 PointsUnsubscribed User
1,321 PointsThis is just a formatted version of your code so I can read it a little better.