Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial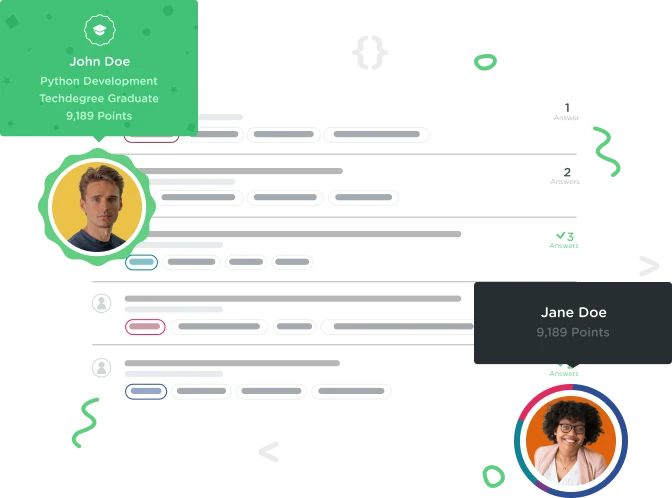
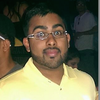
Mathew Kurian
4,698 PointsMy program only outputs the third question. :(
Hi, and thanks in advance for taking a look at my problem! I basically have two questions; one is about my program and the other is about getElementById().
1) What is wrong with my program? It only outputs the third question. I've been coding everything in workspaces, and the HTML page has the correct div tag. Everything was working fine and the program was outputting correctly, until I use the .getElementById() method. I've spent a while looking for errors, but anything I fix seems to just make a problem somewhere else. Here is my code.
var rightCounter = 0;
var correct = [0,0,0,0];
var answer = [];
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = '<p>' + message + '</p>';
}
var questions = [ ['What color is the sky?', 'Blue'],
['What color is a Gala apple?', 'Red'],
['Can a hummingbird fly backwards?', 'Yes'],
['What color are sunflowers?', 'Yellow'] ];
for(var i = 0; i < questions.length; i++) {
answer[i] = prompt(questions[i][0]).toLowerCase();
if (answer[i] === questions[i][1].toLowerCase()) {
correct[i] = 1;
rightCounter++; } }
print("You got " + rightCounter + " question(s) right. Congratulations!");
print("You got these questions right: ");
for (var i = 0; i < questions.length; i += 1) {
if (correct[i] === 1) {
print(i+1 + '. ' + questions[i][0]); }
}
print("You got these questions wrong: ");
for (var i = 0; i < questions.length; i += 1) {
if (correct[i] === 0) {
print(i+1 + '. ' + questions[i][0]); }
}
Also I don't really understand the getElementById() method. How does it work? What's the role of the div tag in the method? From my understanding the compiler would search for the div tag in the document and write that variable into the code?
1 Answer
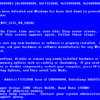
Daniel Powell
23,321 PointsIn the lesson, Dave first makes an HTML string before printing it only once. With your current code you are actually overwriting the inner HTML in your output DIV every single time you print to it. This is happening because you are printing to it with an expression (=). You should instead be concatenating (+=) it like a string to get the results you are expecting.
function print(message) {
document.getElementById('output').innerHTML += '<p>' + message + '</p>'
}
As to your second question:
The div element doesn't really represent anything. Its only purpose is to group together content for styling or scripting purposes.
Then, in your HTML, you are naming this blank region "output" with an ID tag. By doing this you can then refer to this specific region within your Javascript and/or CSS files.
Lastly, all the getElementByID method does is return a reference to some section of your HTML code specified by an ID.
These three topics allow you to programmatically reference and output code to a named region in your HTML document. Your output can then be interpreted as HTML by the browser. By doing this you are dynamically creating HTML with JavaScript.
Mathew Kurian
4,698 PointsMathew Kurian
4,698 PointsAh that makes sense. I didn't realize that we needed to concatenate it. Thank you.
I still don't understand the getElementByID. I read the Mozilla resources on it, but they're very brief. How does getElementByID act as a replacement for document.write()? What advantages does it have over document.write? You said it returns a reference to HTML code, so do we have declare our variables within the HTML code, or does it also work with Javascript?
Daniel Powell
23,321 PointsDaniel Powell
23,321 PointsThe getElementById method is not replacing document.write. In this exercise, to be completely accurate, you aren't actually printing anything at all.
In this instance you are assigning a string to the named element's innerHTML property. This JavaScript string is then parsed as HTML during runtime.
Let's break this code down into smaller bits and step through it.
First we want to select the entire webpage object.
document
Then we want to, more specifically, select a single named div found somewhere in the document object. We know the div has been given the name 'output'. We can do this by using dot notation and the getElementById method that is associated with the document object.
document.getElementById('output')
Now we will need to access the actual HTML found within the div (it currently holds no HTML). We can do this by accessing the output div's innerHTML property.
document.getElementById('output').innerHTML
Lastly, now that we have targeted the named div's content, we can assign this innerHTML property the string we want it to display in the index.html document.
document.getElementById('output').innerHTML += '<p>' + message + '</p>'
In essence, all this code is just inserting a string into the HTML document (this string will then be parsed into actual HTML during runtime). getElementById is just a method to target the HTML code you want to work with, nothing more.
I hope this helps. Let me know if this still isn't making sense.