Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial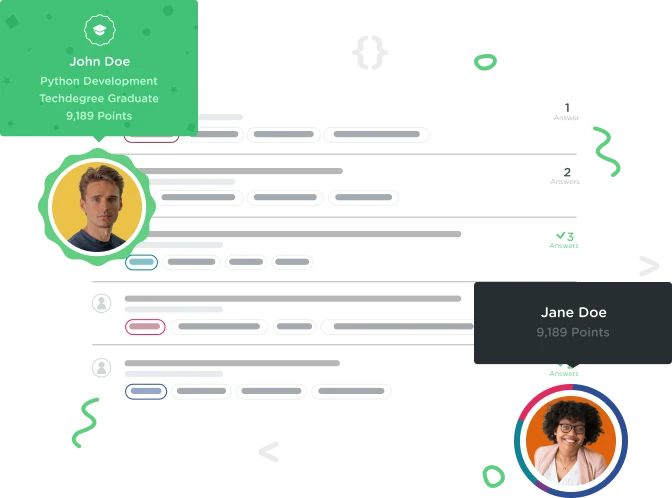

Parimal Raghavan
Courses Plus Student 1,940 PointsMy program works, but it isn't being accepted!
At least it seems to! When I entered "I am that I am" in the IDLE with this code, I got the correct output! What's going on? Am I wrong somehow?
# E.g. word_count("I am that I am") gets back a dictionary like:
# {'i': 2, 'am': 2, 'that': 1}
# Lowercase the string to make it easier.
# Using .split() on the sentence will give you a list of words.
# In a for loop of that list, you'll have a word that you can
# check for inclusion in the dict (with "if word in dict"-style syntax).
# Or add it to the dict with something like word_dict[word] = 1.
def word_count(s):
s=s.split()
x=0
d={}
while x<len(s):
s[x]=s[x].lower()
s[x].strip(" ")
x=x+1
n_list=[]
x=0
c=1
while x<len(s):
if s[x] not in n_list:
n_list.append(s[x])
d.update({s[x]:c})
x=x+1
else:
d.update({s[x]:c+1})
x=x+1
return(d)
1 Answer
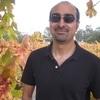
Kourosh Raeen
23,733 PointsIt might be easier to follow the suggestions provided by first lowercasing the sentence and then splitting it into an array of words. Also there is not need for the n_list array. Just check to see if the word is in the dictionary. If not give it a value of 1. If it already is in the dictionary then increment its value.
Here's my code:
def word_count(s):
s = s.lower()
words = s.split()
word_dict = {}
for word in words:
if word in word_dict:
word_dict[word] += 1
else:
word_dict[word] = 1
return word_dict