Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial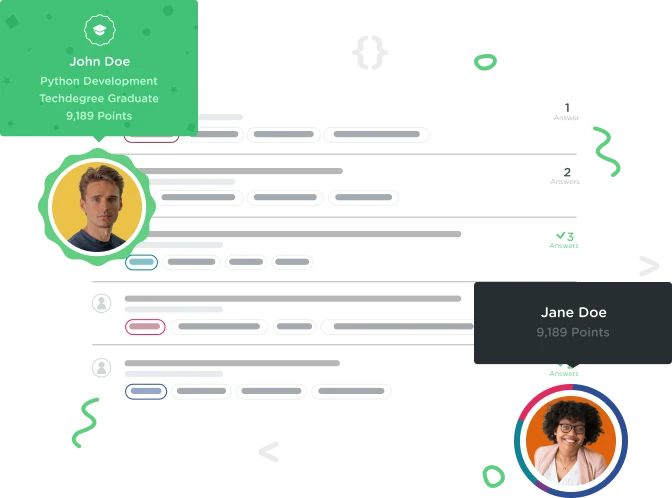

francisobrien
2,131 PointsMy prompt() won't prompt... am I missing something here?
let correct = 0;
let answeredCorrectly = [];
let answeredIncorrectly = [];
let quiz = [
["What is the meaning of life?", 42],
["What color is Donald Trump?", "Orange"],
["Who is the richest man in the world?", "Jeff Bezos"]
];
for (let item = 0; item === quiz.length; item++) {
let question = quiz[item][0];
let answer = quiz[item][1];
let userResponse = parseInt( prompt(question) );
if (userResponse === answer) {
correct++;
answeredCorrectly.push(question);
} else {
answeredIncorrectly.push(question);
}
}
document.querySelector("p").innerHTML = `You got ${correct} out of 3 correct! <br>You got these right: ${answeredCorrectly.join(', ')}. <br> And these wrong: ${answeredIncorrectly.join(', ')}.`;
2 Answers

Blake Larson
13,014 Pointsitem === quiz.length <-- your for loop never runs. You want the for loop to run while item is less than quiz.length.

francisobrien
2,131 PointsThanks Blake. Guess I should have spotted that one! Hm.

Blake Larson
13,014 PointsNo problem.
Xavier Ritch
11,099 PointsXavier Ritch
11,099 PointsIt seems you stored your prompt in a variable thats a part of the local scope of your for loop. Which should be fine, but in order for the prompt to run, that variable needs to be called somewhere in your code, which did not happen. In order for your quiz app to run the way I think you intend it to, i'd recommend you declare the userResponse, answer, and question variables in global scope outside of your for loop and then call on them in the loop. Hope this helps.