Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial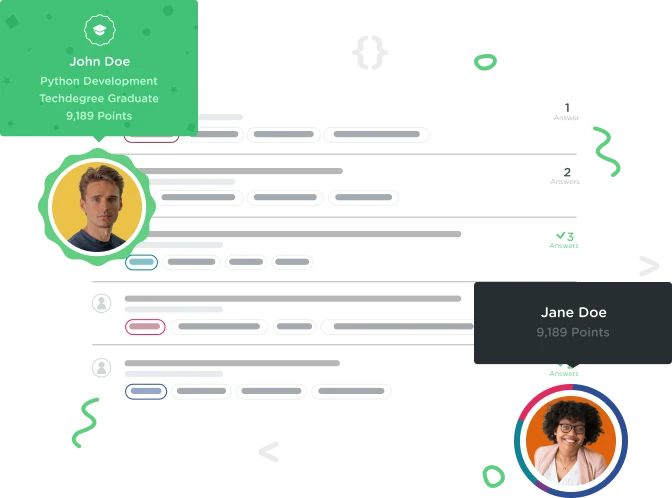

Maria Te
8,521 PointsMy purple and blue views aren't showing up. What is wrong?
I've been copying most of the code Pasaan shows but the purple and blue views aren't there. Only my orange view shows up. Can you explain? Oh, and can someone explain why ".topLayoutGuide" isn't showing up on my compiler. If it is just that I have a new iOS style, just say it. Thanks!
6 Answers

Simon Di Giovanni
8,429 PointsOk so thanks for sending your code!
I have checked it and found your problem. In the 'viewDidLoad' method, you've given a colour for the orange view, but not the purple and blue views!
You also need to 'add' the views using view.addSubview()
So, your 'viewDidLoad' method should look like this -
override func viewDidLoad() {
super.viewDidLoad()
orangeView.backgroundColor = UIColor(red: 255/255.0, green: 148/255.0, blue: 0/255.0, alpha: 1)
view.addSubview(orangeView)
purpleView.backgroundColor = UIColor(red: 204/255.0, green: 102/255.0, blue: 255/255.0, alpha: 1)
view.addSubview(purpleView)
blueView.backgroundColor = UIColor(red: 102/255.0, green: 204/255.0, blue: 255/255.0, alpha: 1)
view.addSubview(blueView)
}
Try that out, and let me know if it works!

Maria Te
8,521 PointsSure! It really helped, Simon!

Simon Di Giovanni
8,429 PointsNo worries. Glad I could help!

Simon Di Giovanni
8,429 PointsCould you please show your code? Then I can check for any inconsistencies.
For this second question - try using .topMargin for the TopSpaceConstraint for both the purple and blue views.
Example -
let blueViewTopSpaceConstraint = NSLayoutConstraint(item: blueView, attribute: .top, relatedBy: .equal, toItem: view, attribute: .topMargin, multiplier: 1.0, constant: 8)

Maria Te
8,521 PointsThanks, but I meant the "self.topLayoutGuide" for the "toItem" parameter. And I can't really show my code. But thanks for trying anyway.

Simon Di Giovanni
8,429 PointsSo self.topLayoutGuide is deprecated, meaning it is no longer used. The layout guide for the view controller is now called the “safe area”
Regarding this particular video, using. .topMargin will achieve the same result as self.topLayoutGuide
Finallly - why can’t you show your code? Just copy and paste the code, and make sure to check the “markdown cheatsheet” written in bold at the bottom for any tips on pasting your code in.

Maria Te
8,521 PointsOkay, Thanks Simon! This is all of the code right after I did your answer :
'''swift import UIKit
class ViewController: UIViewController {
let orangeView = UIView()
let purpleView = UIView()
let blueView = UIView()
override func viewDidLoad() {
super.viewDidLoad()
orangeView.backgroundColor = UIColor(red: 255/255.0, green: 148/255.0, blue: 0/255.0, alpha: 1.0)
view.addSubview(orangeView)
}
override func viewWillLayoutSubviews() {
setupOrangeViewConstraints()
setupPurpleViewConstraints()
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
// Dispose of any resources that can be recreated.
}
func setupOrangeViewConstraints() {
orangeView.translatesAutoresizingMaskIntoConstraints = false
let orangeViewCenterXConstraint = NSLayoutConstraint(item: orangeView, attribute: .centerX, relatedBy: .equal, toItem: view, attribute: .centerXWithinMargins, multiplier: 1.0, constant: 0.0)
let orangeViewBottomSpaceConstraint = NSLayoutConstraint(item: orangeView, attribute: .bottom, relatedBy: .equal, toItem: view, attribute: .bottomMargin, multiplier: 1.0, constant: -50.0)
let orangeViewHeightConstraint = NSLayoutConstraint(item: orangeView, attribute: .height, relatedBy: .equal, toItem: nil, attribute: .notAnAttribute, multiplier: 1.0, constant: 57.0)
let orangeViewWidthConstraint = NSLayoutConstraint(item: orangeView, attribute: .width, relatedBy: .equal, toItem: nil, attribute: .notAnAttribute, multiplier: 1.0, constant: 200.0)
view.addConstraints([orangeViewCenterXConstraint, orangeViewBottomSpaceConstraint, orangeViewHeightConstraint, orangeViewWidthConstraint])
}
func setupPurpleViewConstraints() {
purpleView.translatesAutoresizingMaskIntoConstraints = false
let purpleViewLeadingSpaceConstraint = NSLayoutConstraint(item: purpleView, attribute: .leading, relatedBy: .equal, toItem: view, attribute: .leadingMargin, multiplier: 1.0, constant: 8.0)
let purpleViewBottomSpaceConstraint = NSLayoutConstraint(item: purpleView, attribute: .bottom, relatedBy: .equal, toItem: orangeView, attribute: .top, multiplier: 1.0, constant: -8.0)
let purpleViewTrailingEdgeConstraint = NSLayoutConstraint(item: purpleView, attribute: .trailing, relatedBy: .equal, toItem: view, attribute: .trailingMargin, multiplier: 1.0, constant: -8.0)
let purpleViewTopSpaceConstraint = NSLayoutConstraint(item: purpleView, attribute: .top, relatedBy: .equal, toItem: self.additionalSafeAreaInsets, attribute: .topMargin, multiplier: 1.0, constant: 8.0)
view.addConstraints([purpleViewBottomSpaceConstraint, purpleViewLeadingSpaceConstraint, purpleViewTrailingEdgeConstraint, purpleViewTopSpaceConstraint])
}
func setupBlueViewConstraints() {
blueView.translatesAutoresizingMaskIntoConstraints = false
let blueViewTopSpaceConstraint = NSLayoutConstraint(item: blueView, attribute: .top, relatedBy: .equal, toItem: self.additionalSafeAreaInsets, attribute: .top, multiplier: 1.0, constant: 8)
let blueViewBottomSpaceConstraint = NSLayoutConstraint(item: blueView, attribute: .bottom, relatedBy: .equal, toItem: orangeView, attribute: .top, multiplier: 1.0, constant: -8.0)
let blueViewTrailingSpaceConstraint = NSLayoutConstraint(item: blueView, attribute: .trailing, relatedBy: .equal, toItem: view, attribute: .trailingMargin, multiplier: 1.0, constant: 8.0)
let equalWidthConstraints = NSLayoutConstraint(item: blueView, attribute: .width, relatedBy: .equal, toItem: purpleView, attribute: .width, multiplier: 1.0, constant: 0.0)
view.addConstraints([blueViewTopSpaceConstraint, blueViewBottomSpaceConstraint, blueViewTrailingSpaceConstraint, equalWidthConstraints])
}
} '''
And by the way, in my Xcode instead of "self.topLayoutGuide" it's "self.additionalSafeAreaInsets". Just so you know.

Maria Te
8,521 PointsThanks again! This time it does work!

Simon Di Giovanni
8,429 PointsNo worries! Please mark my answer as best answer so question is resolved!