Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial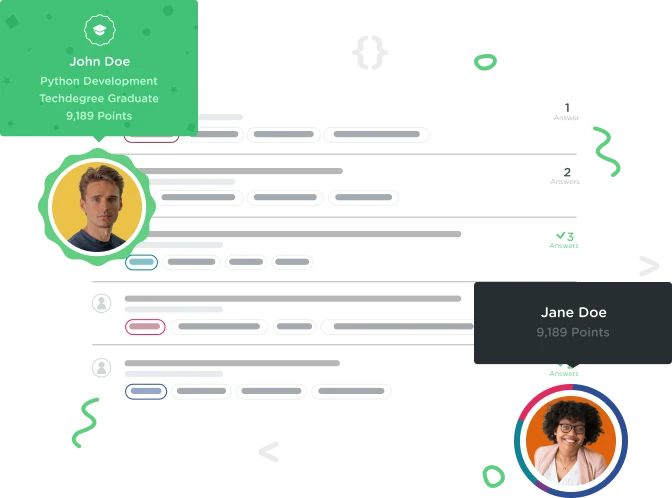

Alexei Ferreira
13,986 PointsMy question is no related to the Jquery: Just want to know how he got the triangle with the css on the box?
How was it done. I'm trying to simulate but I'm having a hard time.
<!DOCTYPE html>
<html>
<head>
<title>Sign Up Form</title>
<link rel="stylesheet" href="css/style.css" type="text/css" media="screen" title="no title" charset="utf-8">
</head>
<body>
<form action="#" method="post">
<p>
<label for="username">Username</label>
<input id="username" name="username" type="text">
</p>
<p>
<label for="password">Password</label>
<input id="password" name="password" type="password">
<span>Enter a password longer than 8 characters</span>
</p>
<p>
<label for="confirm_password">Confirm Password</label>
<input id="confirm_password" name="confirm_password" type="password">
<span>Please confirm your password</span>
</p>
<p>
<input type="submit" value="SUBMIT">
</p>
</form>
<script src="http://code.jquery.com/jquery-1.11.0.min.js" type="text/javascript" charset="utf-8"></script>
<script src="js/app.js" type="text/javascript" charset="utf-8"></script>
</body>
</html>
body {
background: #384047;
font-family: sans-serif;
font-size: 10px
}
form {
background: #fff;
padding: 4em 4em 2em;
max-width: 400px;
margin: 100px auto 0;
box-shadow: 0 0 1em #222;
border-radius: 5px;
}
p {
margin: 0 0 3em 0;
position: relative;
}
label {
display: block;
font-size: 1.6em;
margin: 0 0 .5em;
color: #333;
}
input {
display: block;
box-sizing: border-box;
width: 100%;
outline: none
}
input[type="text"],
input[type="password"] {
background: #f5f5f5;
border: 1px solid #e5e5e5;
font-size: 1.6em;
padding: .8em .5em;
border-radius: 5px;
}
input[type="text"]:focus,
input[type="password"]:focus {
background: #fff
}
span {
border-radius: 5px;
display: block;
font-size: 1.3em;
text-align: center;
position: absolute;
background: #2F558E;
left: 105%;
top: 25px;
width: 160px;
padding: 7px 10px;
color: #fff;
}
span:after {
right: 100%;
top: 50%;
border: solid transparent;
content: " ";
height: 0;
width: 0;
position: absolute;
pointer-events: none;
border-color: rgba(136, 183, 213, 0);
border-right-color: #2F558E;
border-width: 8px;
margin-top: -8px;
}
input[type="submit"] {
background: #2F558E;
box-shadow: 0 3px 0 0 #1D3C6A;
border-radius: 5px;
border: none;
color: #fff;
cursor: pointer;
display: block;
font-size: 2em;
line-height: 1.6em;
margin: 2em 0 0;
outline: none;
padding: .8em 0;
text-shadow: 0 1px #68B25B;
}
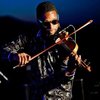
Joshua Harman
8,821 PointsI know it's somewhere here: https://teamtreehouse.com/library/jquery-basics , but I don't recall anything about a triangle.. What video did you see it in?

Alexei Ferreira
13,986 Pointsthat's the thing, it is not on the course, the course is about jquery, the project uses this comments on the password and password confirmation, I want to learn How the did the note box.
1 Answer

Craig Watson
27,930 PointsHi,
I think you are referring to this bit of CSS
span:after {
right: 100%;
top: 50%;
border: solid transparent;
content: " ";
height: 0;
width: 0;
position: absolute;
pointer-events: none;
border-color: rgba(136, 183, 213, 0);
border-right-color: #2F558E;
border-width: 8px;
margin-top: -8px;
Creating the triangle or arrow on the note?
CSS Tricks has a great page on CSS shapes here
You would add the triangle as above using a ::after pseudo element with absolute positioning.
Hope this helps Craig

Alexei Ferreira
13,986 PointsThanks Craig that's exactly what I was looking for!

Craig Watson
27,930 PointsNo problem at all Alexei,
Happy Coding :)
rydavim
18,814 Pointsrydavim
18,814 PointsCould you link the files or video you're referencing?