Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial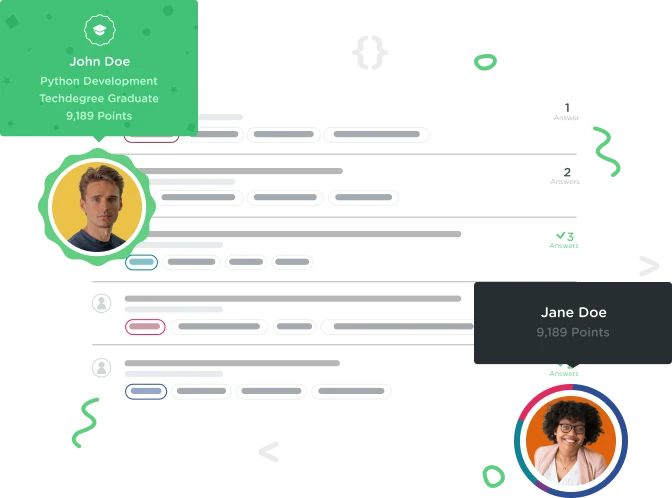
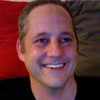
Darryn Smith
32,043 PointsMy Quiz solution. Comments welcome.
This was a fun challenge. I'm pretty happy with the result, but am always open to comments from the pros. If you have a minute (who actually does!?) let me know if you would (or at least think I should) handle something differently.
Thanks!
Quiz Challenge: working version:
// questionsAndAnswers[][] - a 2D array with 3 fields per row. I decided to use the array to track answers so I could
// use a for loop to quickly print out a custom list of correct answers the test-taker missed
var questionsAndAnswers= [
["color?", "RED", false],
["flavor?", "SPICY", false],
["time?", "DAWN", false]
];
// correctAnswerCount - used as a simple count of correct answers for scorekeeping and flow control
var correctAnswerCount = 0;
// response - storage of the test-taker's response
var response = '';
// message - used to build the final message conveying score and corrected answers
var message = '';
// for loop to ask the questions, get the answers, keep the score
// responses are normalized to uppercase and compared
// correct answers set the third field of each array row to 'true' and adds one to the score
for( i=0; i<questionsAndAnswers.length; i += 1) {
response = prompt('What is my favorite ' + questionsAndAnswers[i][0]);
if (response .toUpperCase() === questionsAndAnswers[i][1]) {
questionsAndAnswers[i][2] = true;
correctAnswerCount += 1;
}
}
// A perfect score gets a simple message.
// A score of zero gets a rude message.
// A mixed score displays the score and lists the missed questions with their correct answers
if (correctAnswerCount === questionsAndAnswers.length) {
document.write('Congratulations, you got a perfect score!');
} else if (correctAnswerCount === 0) {
document.write( "Total fail. I'm out." );
} else {
message = '<p>You got ' + correctAnswerCount + ' correct. Here is where you went wrong:</p>';
for(i=0; i<questionsAndAnswers.length; i+=1) {
if (questionsAndAnswers[i][2] === false) {
message = message + '<p>' + i + '. What is my favorite ' + questionsAndAnswers[i][0] + ' Answer: ' + questionsAndAnswers[i][1] + '</p>';
}
}
document.write(message);
}
1 Answer

Iain Simmons
Treehouse Moderator 32,305 PointsNice!
A few suggestions/comments:
First, I like the idea of using a question prefix 'What is my favorite', but since you have to write it out a couple of times, why not store it in a variable also? That way you could change it easily too, if you wanted a different style of question.
Second, in the conditional statement at the end, you only use the message
variable in the else case, but you could actually use it in all three and then just stick document.write(message)
on the outside of the conditional statement.
Third, you don't use any paragraph tags if the user got all or none right... might as well throw those in for consistency!