Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial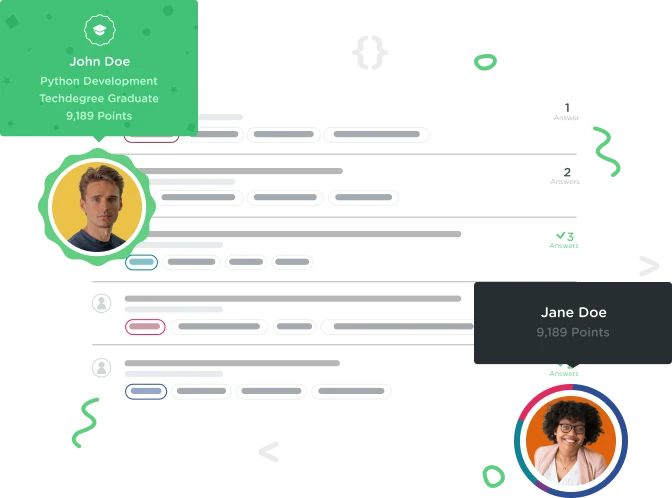
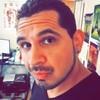
Daniel LittleThunder
6,975 PointsMy Random Number Challenge Part II Solution
Hey there everyone. I just wanted to share my solution to the Random Number Challenge Part II. It's not really what was required of the task, but I went ahead and used all of the skills from the previous videos to create a function that accepts 2 arguments for the parameters based on user input.
This solution uses numeric data provided by user input as the arguments for the function. I employed the prompts, alerts, loops, parseInt, and several variable uses, and functions. Below is the code. I hope it helps others see what could be done and how to accept user input with this particular function.
The HTML:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<link rel="stylesheet" href="css/main.css">
<title>Let's Make a Function</title>
</head>
<body>
<div class="container">
<!--<h1>Let's Make a Function</h1>-->
<div id="start">
</div>
<script src="random.js"></script>
</div>
</body>
</html>
The JS:
//Sets up function with 2 parameters to return random number based on 2 arguments
function returnRandNum(upper, lower) {
if (isNaN(upper) || isNaN(lower)) {
throw new Error("Slow your horses! One of these values is not a number!");
} else {
return Math.floor(Math.random() * (upper - lower + 1)) + lower;
}
}
//Sets up prompts and alerts as function which can be called by button
function playGame() {
//Prompts user for upper numeric input
var upNum = parseInt(Math.round(prompt('Okay! To start, please enter any number greater than 0.')));
//Prompts user for numeric input while input isNaN && <= 0
while (isNaN(upNum) || upNum <= 0) {
var entNum = prompt("I'm sorry, but you must enter a number which is greater than 0.");
upNum = parseInt(entNum);
}
//Prompts user for lower numeric input
var lowNum = parseInt(Math.round(prompt('Awesome! Now enter any number greater or less than the previously entered number. The number you enter must also be greater than 0.')));
//Prompts user for numeric input while input isNaN && <=0 && === previous input
while (isNaN(lowNum) || lowNum <= 0 || lowNum === upNum) {
var entNum = prompt("I'm sorr, but you must enter a number which is greater than 0, and greater or less than your previous number.");
lowNum = parseInt(entNum);
}
//Alerts user of next step
alert("Great! You entered " + upNum + " and " + lowNum + ". Now we're going to generate a random number between the two numbers you chose. Let's go!")
//Alerts user of random number
var message = "Your random number between " + upNum + " and " + lowNum + " is ";
message += returnRandNum(upNum, lowNum) + "!";
alert(message);
}
//Creates button for starting game
//Calls <div> element with id="start" from index.html
document.getElementById("start").innerHTML = `
<h1>LET'S GUESS SOME NUMBERS!</h1>
<div>
<button onclick="playGame()">Play</button>
</div>
`;
1 Answer

Jamin Pratt
Full Stack JavaScript Techdegree Student 1,920 PointsWow, that is impressive. It was much better than the original example but more complicated. Great Job!