Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial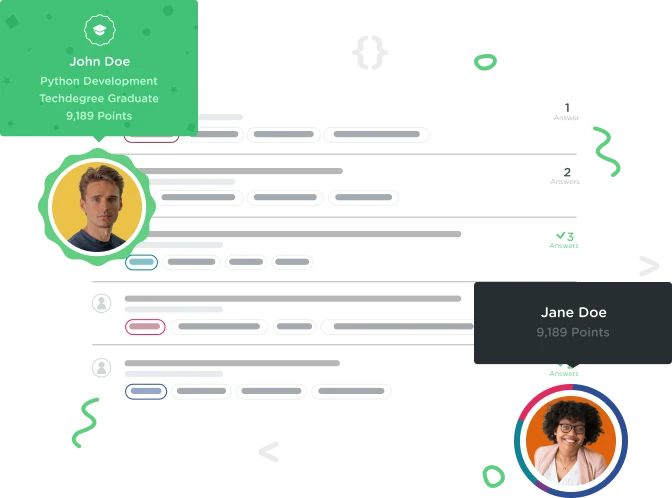
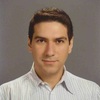
Burak Samra
2,431 PointsMy result for displaying a random number between two inputs.
Hi. I completed Jim's Introduction to Programming course before this one, so I kind of went ahead and used some conditional stuff and a loop :) My code seems to work but I feel like I have written a little too much and repeated myself a little. Could I ask for some suggestions for improvement?
Thanks & cheers!
/***** RANDOM NUMBER GENERATOR *****/
// Ask for user input
var input1 = parseInt(prompt('Give me a number:'));
var input2 = parseInt(prompt('Nice! Now give me another number:'));
// Make sure two inputs are different
while(input1 == input2) {
input2 = parseInt(prompt('Please give me a different number:'));
}
// Display inputs
console.log('Input 1: ' + input1);
console.log('Input 2: ' + input2);
// Make sure the difference of the two inputs is positive.
var diff = input1 - input2;
console.log('Difference of inputs: ' + diff);
if(diff < 0) {
diff *= -1;
}
// Filler
alert('Thank you! Now I\'ll give you a number back, generated between the ones you provided.');
// Random number between 1 and the absolute value of the difference of two inputs.
var result = Math.floor(Math.random() * diff) + 1;
console.log('Result before: ' + result);
// Find and add the smaller input to the result so that the result becomes between the two inputs, including both of them.
if(input1 < input2){
result += Math.floor(input1);
} else {
result += Math.floor(input2);
}
console.log('Result after: ' + result);
// Display result
alert('Your random number is ' + result + '!');
1 Answer

LaVaughn Haynes
12,397 PointsOK. I'll give it a shot. I added some extra stuff as well such as verifying that numbers entered are numbers and throwing errors.
/*----------------
THE SETUP
----------------*/
// vars
var minNumber,
maxNumber,
randomNum,
requestMinNumber = "Give me a number:",
minNumberConfirmation = "Nice!",
requestMaxNumber = "Now give me another number that is bigger than the first number:",
maxNumberConfirmation = "Thank you! Now I'll give you a random integer between the numbers that you provided.";
// prompt user for a valid integer
var getInt = function(question){
var theNumber;
do{
theNumber = parseInt( prompt(question) );
}while( isNaN(theNumber) );
return theNumber;
};
// generate a random number between 2 values
var getRandomIntegerBetween = function( minLimit, maxLimit ){
if( isNaN(minLimit) || isNaN(maxLimit) ){
throw new Error("Error: getRandomIntegerBetween(num1, num2) requires 2 numeric values");
}
return Math.floor( Math.random() * ( maxLimit - minLimit + 1 )) + minLimit;
};
/*----------------
START THE WORK
----------------*/
// get the low number
minNumber = getInt( requestMinNumber );
// confirm reciept of the first number
alert( minNumberConfirmation );
// get the high number
do{
maxNumber = getInt( requestMaxNumber );
}while( minNumber >= maxNumber );
// confirm reciept of the second number
alert( maxNumberConfirmation );
// get random number
randomNum = getRandomIntegerBetween( minNumber, maxNumber );
// display number
alert( 'Your random number is ' + randomNum + '!' );
// log results
console.log( 'min: ' + minNumber );
console.log( 'max: ' + maxNumber );
console.log( 'random: ' + randomNum );
Burak Samra
2,431 PointsBurak Samra
2,431 PointsOK, I just found a glitch on line 27. Before the correction, it does not include the smaller number in the result interval, now it does.