Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial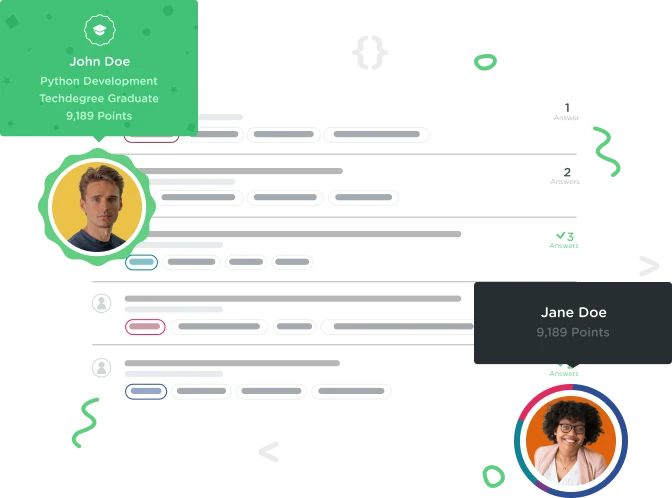

Fuseini Isaac
Courses Plus Student 2,225 PointsMy Ribbit app crashes anytime i click on the send button
this is my recipient activity
package com.instantmedia.swerve;
import java.util.ArrayList;
import java.util.List;
import android.app.AlertDialog;
import android.app.ListActivity;
import android.net.Uri;
import android.os.Bundle;
import android.support.v4.app.NavUtils;
import android.util.Log;
import android.view.Menu;
import android.view.MenuItem;
import android.view.View;
import android.view.Window;
import android.widget.ArrayAdapter;
import android.widget.ListView;
import android.widget.Toast;
import com.parse.FindCallback;
import com.parse.ParseException;
import com.parse.ParseFile;
import com.parse.ParseObject;
import com.parse.ParseQuery;
import com.parse.ParseRelation;
import com.parse.ParseUser;
import com.parse.SaveCallback;
public class RecipientsActivity extends ListActivity {
public static final String TAG = RecipientsActivity.class.getSimpleName();
protected List<ParseUser> mFriends;
protected ParseRelation<ParseUser> mFriendsRelation;
protected ParseUser mCurrentUser;
protected MenuItem mSendMenuItem;
protected Uri mMediaUri;
protected String mFileType;
protected String mMyMessage;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
requestWindowFeature(Window.FEATURE_INDETERMINATE_PROGRESS);
setContentView(R.layout.activity_recipients);
//show up button in action bar
setupActionBar();
getListView().setChoiceMode(ListView.CHOICE_MODE_MULTIPLE);
mMediaUri = getIntent().getData();
mFileType = getIntent().getExtras().getString(ParseConstants.KEY_FILE_TYPE);
mMyMessage = getIntent().getExtras().getString("themessage");
}
@Override
public void onResume() {
super.onResume();
mCurrentUser = ParseUser.getCurrentUser();
mFriendsRelation = mCurrentUser.getRelation(ParseConstants.KEY_FRIENDS_RELATION);
setProgressBarIndeterminateVisibility(true);
ParseQuery<ParseUser> query = mFriendsRelation.getQuery();
query.addAscendingOrder(ParseConstants.KEY_USERNAME);
query.findInBackground(new FindCallback<ParseUser>() {
@Override
public void done(List<ParseUser> friends, ParseException e) {
setProgressBarIndeterminateVisibility(false);
if (e == null){
mFriends = friends;
String[] usernames = new String[mFriends.size()];
int i = 0;
for (ParseUser user : mFriends) {
usernames[i] = user.getUsername();
i++;
}
ArrayAdapter<String> adapter = new ArrayAdapter<String>(
getListView().getContext(),
android.R.layout.simple_list_item_checked,
usernames);
setListAdapter(adapter);
}
else {
Log.e(TAG, e.getMessage());
AlertDialog.Builder builder = new AlertDialog.Builder(RecipientsActivity.this);
builder.setMessage(e.getMessage())
.setTitle(R.string.error_title)
.setPositiveButton(android.R.string.ok, null);
AlertDialog dialog = builder.create();
dialog.show();
}
}
});
}
private void setupActionBar(){
getActionBar().setDisplayHomeAsUpEnabled(true);
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.recipients, menu);
mSendMenuItem = menu.getItem(0);
return true;
}
@Override
public boolean onOptionsItemSelected(MenuItem item) {
switch (item.getItemId()) {
case android.R.id.home:
// This ID represents the Home or Up button. In the case of this
// activity, the Up button is shown. Use NavUtils to allow users
// to navigate up one level in the application structure. For
// more details, see the Navigation pattern on Android Design:
//
// http://developer.android.com/design/patterns/navigation.html#up-vs-back
//
NavUtils.navigateUpFromSameTask(this);
return true;
case R.id.action_send:
ParseObject message = createMessage();
if (message == null) {
// error
AlertDialog.Builder builder = new AlertDialog.Builder(this);
builder.setMessage(R.string.error_selecting_file)
.setTitle(R.string.error_selecting_file_title)
.setPositiveButton(android.R.string.ok, null);
AlertDialog dialog = builder.create();
dialog.show();
}
else {
send(message);
finish();
}
return true;
}
return super.onOptionsItemSelected(item);
}
@Override
protected void onListItemClick(ListView l, View v, int position, long id) {
super.onListItemClick(l, v, position, id);
if (l.getCheckedItemCount() > 0) {
mSendMenuItem.setVisible(true);
}
else {
mSendMenuItem.setVisible(false);
}
}
protected ParseObject createMessage(){
ParseObject message = new ParseObject(ParseConstants.CLASS_MESSAGES);
message.put(ParseConstants.KEY_SENDER_ID, ParseUser.getCurrentUser().getObjectId());
message.put(ParseConstants.KEY_SENDER_NAME, ParseUser.getCurrentUser().getUsername());
message.put(ParseConstants.KEY_RECIPIENT_IDS, getRecipientIds());
message.put(ParseConstants.KEY_FILE_TYPE, mFileType);
if (mFileType.equals(ParseConstants.TYPE_TEXT)) {
message.put("themessage", mMyMessage);
message.put(ParseConstants.KEY_FILE_TYPE, "message");
return message;
}else{
byte[] fileBytes = FileHelper.getByteArrayFromFile(this, mMediaUri);
if (fileBytes == null) {
return null;
}
else {
if (mFileType.equals(ParseConstants.TYPE_IMAGE)) {
fileBytes = FileHelper.reduceImageForUpload(fileBytes);
}
String fileName = FileHelper.getFileName(this, mMediaUri, mFileType);
ParseFile file = new ParseFile(fileName, fileBytes);
message.put(ParseConstants.KEY_FILE, file);
return message;
}}
}
protected ArrayList<String> getRecipientIds(){
ArrayList<String> recipientIds = new ArrayList<String>();
for (int i = 0; i < getListView().getCount(); i++) {
if (getListView().isItemChecked(i)){
recipientIds.add(mFriends.get(i).getObjectId());
}
}
return recipientIds;
}
protected void send(ParseObject message) {
message.saveInBackground(new SaveCallback() {
@Override
public void done(ParseException e) {
if (e == null) {
//Success!
Toast.makeText(RecipientsActivity.this, R.string.success_message, Toast.LENGTH_LONG).show();
}
else {
AlertDialog.Builder builder = new AlertDialog.Builder(RecipientsActivity.this);
builder.setMessage(R.string.error_sending_message)
.setTitle(R.string.error_selecting_file_title)
.setPositiveButton(android.R.string.ok, null);
AlertDialog dialog = builder.create();
dialog.show();
}
}
});
}
}
this is my logcat
03-28 04:20:54.426: W/dalvikvm(7938): threadid=1: thread exiting with uncaught exception (group=0x4193f700) 03-28 04:20:54.431: E/AndroidRuntime(7938): FATAL EXCEPTION: main 03-28 04:20:54.431: E/AndroidRuntime(7938): java.lang.RuntimeException: Unable to start activity ComponentInfo{com.instantmedia.swerve/com.instantmedia.swerve.RecipientsActivity}: java.lang.NullPointerException 03-28 04:20:54.431: E/AndroidRuntime(7938): at android.app.ActivityThread.performLaunchActivity(ActivityThread.java:2295) 03-28 04:20:54.431: E/AndroidRuntime(7938): at android.app.ActivityThread.handleLaunchActivity(ActivityThread.java:2349) 03-28 04:20:54.431: E/AndroidRuntime(7938): at android.app.ActivityThread.access$700(ActivityThread.java:159) 03-28 04:20:54.431: E/AndroidRuntime(7938): at android.app.ActivityThread$H.handleMessage(ActivityThread.java:1316) 03-28 04:20:54.431: E/AndroidRuntime(7938): at android.os.Handler.dispatchMessage(Handler.java:99) 03-28 04:20:54.431: E/AndroidRuntime(7938): at android.os.Looper.loop(Looper.java:137) 03-28 04:20:54.431: E/AndroidRuntime(7938): at android.app.ActivityThread.main(ActivityThread.java:5419) 03-28 04:20:54.431: E/AndroidRuntime(7938): at java.lang.reflect.Method.invokeNative(Native Method) 03-28 04:20:54.431: E/AndroidRuntime(7938): at java.lang.reflect.Method.invoke(Method.java:525) 03-28 04:20:54.431: E/AndroidRuntime(7938): at com.android.internal.os.ZygoteInit$MethodAndArgsCaller.run(ZygoteInit.java:1187) 03-28 04:20:54.431: E/AndroidRuntime(7938): at com.android.internal.os.ZygoteInit.main(ZygoteInit.java:1003) 03-28 04:20:54.431: E/AndroidRuntime(7938): at dalvik.system.NativeStart.main(Native Method) 03-28 04:20:54.431: E/AndroidRuntime(7938): Caused by: java.lang.NullPointerException 03-28 04:20:54.431: E/AndroidRuntime(7938): at com.instantmedia.swerve.RecipientsActivity.onCreate(RecipientsActivity.java:54) 03-28 04:20:54.431: E/AndroidRuntime(7938): at android.app.Activity.performCreate(Activity.java:5372) 03-28 04:20:54.431: E/AndroidRuntime(7938): at android.app.Instrumentation.callActivityOnCreate(Instrumentation.java:1104) 03-28 04:20:54.431: E/AndroidRuntime(7938): at android.app.ActivityThread.performLaunchActivity(ActivityThread.java:2257) 03-28 04:20:54.431: E/AndroidRuntime(7938): ... 11 more
and this is my MainActivity
package com.instantmedia.swerve;
import java.io.File;
import java.io.FileNotFoundException;
import java.io.IOException;
import java.io.InputStream;
import java.text.SimpleDateFormat;
import java.util.Date;
import java.util.Locale;
import android.app.ActionBar;
import android.app.AlertDialog;
import android.app.FragmentTransaction;
import android.content.DialogInterface;
import android.content.Intent;
import android.net.Uri;
import android.os.Bundle;
import android.os.Environment;
import android.provider.MediaStore;
import android.support.v4.app.FragmentActivity;
import android.support.v4.view.ViewPager;
import android.util.Log;
import android.view.Menu;
import android.view.MenuItem;
import android.view.Window;
import android.widget.EditText;
import android.widget.Toast;
import com.parse.ParseAnalytics;
import com.parse.ParseUser;
public class MainActivity extends FragmentActivity implements
ActionBar.TabListener {
public static final String TAG = MainActivity.class.getSimpleName();
public static final int TAKE_PHOTO_REQUEST = 0;
public static final int TAKE_VIDEO_REQUEST = 1;
public static final int PICK_PHOTO_REQUEST = 2;
public static final int PICK_VIDEO_REQUEST = 3;
public static final int MEDIA_TYPE_IMAGE = 4;
public static final int MEDIA_TYPE_VIDEO = 5;
public static final int FILE_SIZE_LIMIT = 1024*1024*10; // 10MB
protected Uri mMediaUri;
protected DialogInterface.OnClickListener mDialogListener =
new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
switch(which){
case 0: // take picture
Intent takePhotoIntent = new Intent(MediaStore.ACTION_IMAGE_CAPTURE);
mMediaUri = getOutputMediaFileUri(MEDIA_TYPE_IMAGE);
if (mMediaUri == null) {
// display error
Toast.makeText(MainActivity.this, R.string.error_external_storage,
Toast.LENGTH_LONG).show();
}
else {
takePhotoIntent.putExtra(MediaStore.EXTRA_OUTPUT, mMediaUri);
startActivityForResult(takePhotoIntent, TAKE_PHOTO_REQUEST);
}
break;
case 1: // take video
Intent videoIntent = new Intent(MediaStore.ACTION_VIDEO_CAPTURE);
mMediaUri = getOutputMediaFileUri(MEDIA_TYPE_VIDEO);
if (mMediaUri == null) {
// display error
Toast.makeText(MainActivity.this, R.string.error_external_storage,
Toast.LENGTH_LONG).show();
}
else {
videoIntent.putExtra(MediaStore.EXTRA_OUTPUT, mMediaUri);
videoIntent.putExtra(MediaStore.EXTRA_DURATION_LIMIT, 10);
videoIntent.putExtra(MediaStore.EXTRA_VIDEO_QUALITY, 0); // 0 for lowest res
startActivityForResult(videoIntent, TAKE_VIDEO_REQUEST);
}
break;
case 2: // choose picture
Intent choosePhotoIntent = new Intent(Intent.ACTION_GET_CONTENT);
choosePhotoIntent.setType("image/*");
startActivityForResult(choosePhotoIntent, PICK_PHOTO_REQUEST);
break;
case 3: // choose video
Intent chooseVideoIntent = new Intent(Intent.ACTION_GET_CONTENT);
chooseVideoIntent.setType("video/*");
Toast.makeText(MainActivity.this, R.string.video_file_size_warning,
Toast.LENGTH_LONG).show();
startActivityForResult(chooseVideoIntent, PICK_VIDEO_REQUEST);
break;
}
}
private Uri getOutputMediaFileUri(int mediaType) {
// To be Safe, You should Check that the SDCard is mounted
// using Environment.getExternalStorageState() before doing this
if (isExternalStorageAvailable()) {
// get the URI
// 1. Get the external Storage directory
String appName = MainActivity.this.getString(R.string.app_name);
File mediaStorageDir = new File(
Environment.getExternalStoragePublicDirectory(Environment.DIRECTORY_PICTURES),
appName);
// 2. Create our Subdirectory
if (! mediaStorageDir.exists() ) {
if (! mediaStorageDir.mkdir()) {
Log.e(TAG, "Failed to create directory.");
return null;
}
}
// 3. Create a file name
// 4. create the file
File mediaFile;
Date now = new Date();
String timestamp = new SimpleDateFormat("yyyyMMdd_HHmmss", Locale.US).format(now);
String path = mediaStorageDir.getPath() + File.separator;
if (mediaType == MEDIA_TYPE_IMAGE){
mediaFile = new File(path + "IMG_" + timestamp + ".jpg");
}
else if (mediaType == MEDIA_TYPE_VIDEO) {
mediaFile = new File(path + "VID_" + timestamp + ".mp4");
}
else {
return null;
}
Log.d(TAG, "file: " + Uri.fromFile(mediaFile));
// 5. Return the file's URI
return Uri.fromFile(mediaFile);
}
else{
return null;
}
}
private boolean isExternalStorageAvailable(){
String state = Environment.getExternalStorageState();
if (state.equals(Environment.MEDIA_MOUNTED)) {
return true;
}
else {
return false;
}
}
};
/**
* The {@link android.support.v4.view.PagerAdapter} that will provide
* fragments for each of the sections. We use a
* {@link android.support.v4.app.FragmentPagerAdapter} derivative, which
* will keep every loaded fragment in memory. If this becomes too memory
* intensive, it may be best to switch to a
* {@link android.support.v4.app.FragmentStatePagerAdapter}.
*/
SectionsPagerAdapter mSectionsPagerAdapter;
/**
* The {@link ViewPager} that will host the section contents.
*/
ViewPager mViewPager;
@Override
protected void onCreate(Bundle savedInstanceState) {
ParseAnalytics.trackAppOpened(getIntent());
super.onCreate(savedInstanceState);
requestWindowFeature(Window.FEATURE_INDETERMINATE_PROGRESS);
setContentView(R.layout.activity_main);
ParseAnalytics.trackAppOpened(getIntent());
ParseUser currentUser = ParseUser.getCurrentUser();
if (currentUser == null){
navigateToLogin();
}
else {
Log.i(TAG, currentUser.getUsername());
}
// Set up the action bar.
final ActionBar actionBar = getActionBar();
actionBar.setNavigationMode(ActionBar.NAVIGATION_MODE_TABS);
// Create the adapter that will return a fragment for each of the three
// primary sections of the app.
mSectionsPagerAdapter = new SectionsPagerAdapter(this,
getSupportFragmentManager());
// Set up the ViewPager with the sections adapter.
mViewPager = (ViewPager) findViewById(R.id.pager);
mViewPager.setAdapter(mSectionsPagerAdapter);
// When swiping between different sections, select the corresponding
// tab. We can also use ActionBar.Tab#select() to do this if we have
// a reference to the Tab.
mViewPager
.setOnPageChangeListener(new ViewPager.SimpleOnPageChangeListener() {
@Override
public void onPageSelected(int position) {
actionBar.setSelectedNavigationItem(position);
}
});
// For each of the sections in the app, add a tab to the action bar.
for (int i = 0; i < mSectionsPagerAdapter.getCount(); i++) {
// Create a tab with text corresponding to the page title defined by
// the adapter. Also specify this Activity object, which implements
// the TabListener interface, as the callback (listener) for when
// this tab is selected.
actionBar.addTab(actionBar.newTab()
.setText(mSectionsPagerAdapter.getPageTitle(i))
.setTabListener(this));
}
}
@Override
protected void onActivityResult(int requestCode, int resultCode, Intent data) {
super.onActivityResult(requestCode, resultCode, data);
if (resultCode == RESULT_OK) {
// ADD TO THE GALLERY and pick them
if (requestCode == PICK_PHOTO_REQUEST || requestCode == PICK_VIDEO_REQUEST) {
if (data == null) {
Toast.makeText(this, getString(R.string.general_error), Toast.LENGTH_LONG).show();
}
else {
mMediaUri = data.getData();
}
Log.i(TAG, "Media URI: " + mMediaUri);
if (requestCode == PICK_VIDEO_REQUEST) {
// make sure the file is less than 10 MB
int fileSize = 0;
InputStream inputStream = null;
try {
inputStream = getContentResolver().openInputStream(mMediaUri);
fileSize = inputStream.available();
}
catch (FileNotFoundException e) {
Toast.makeText(this, R.string.error_opening_file, Toast.LENGTH_LONG).show();
return;
}
catch (IOException e) {
Toast.makeText(this, R.string.error_opening_file, Toast.LENGTH_LONG).show();
return;
}
finally {
try {
inputStream.close();
} catch (IOException e) {/*intentionally blank */}
}
if (fileSize >= FILE_SIZE_LIMIT){
Toast.makeText(this, R.string.error_file_size_too_large, Toast.LENGTH_LONG).show();
return;
}
}
}
else {
Intent mediaScanIntent = new Intent(Intent.ACTION_MEDIA_SCANNER_SCAN_FILE);
mediaScanIntent.setData(mMediaUri);
sendBroadcast(mediaScanIntent);
}
Intent recipientsIntent = new Intent(this, RecipientsActivity.class);
recipientsIntent.setData(mMediaUri);
startActivity(recipientsIntent);
String fileType;
if (requestCode == PICK_PHOTO_REQUEST || requestCode == TAKE_PHOTO_REQUEST) {
fileType = ParseConstants.TYPE_IMAGE;
}
else{
fileType = ParseConstants.TYPE_VIDEO;
}
recipientsIntent.putExtra(ParseConstants.KEY_FILE_TYPE, fileType);
startActivity(recipientsIntent);
}
else if (resultCode != RESULT_CANCELED) {
Toast.makeText(this, R.string.general_error, Toast.LENGTH_LONG).show();
}
}
private void navigateToLogin() {
Intent intent = new Intent(this, LoginActivity.class);
intent.addFlags(Intent.FLAG_ACTIVITY_NEW_TASK);
intent.addFlags(Intent.FLAG_ACTIVITY_CLEAR_TASK);
startActivity(intent);
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.main, menu);
return true;
}
@Override
public boolean onOptionsItemSelected(MenuItem item) {
int itemId = item.getItemId();
switch(itemId) {
case R.id.action_logout:
ParseUser.logOut();
navigateToLogin();
break;
case R.id.action_edit_friends:
Intent intent = new Intent(this, EditFriendsActivity.class);
startActivity(intent);
break;
case R.id.action_camera:
AlertDialog.Builder builder = new AlertDialog.Builder(this);
builder.setItems(R.array.camera_choices, mDialogListener);
AlertDialog dialog = builder.create();
dialog.show();
break;
case R.id.action_message:
//*********************************************************
AlertDialog.Builder alert = new AlertDialog.Builder(this);
alert.setTitle("Send Message.");
alert.setMessage("Type your message below.");
// Set an EditText view to get user input
final EditText input = new EditText(this);
alert.setView(input);
alert.setPositiveButton("Ok", new DialogInterface.OnClickListener() {
public void onClick(DialogInterface dialog, int whichButton) {
String value = input.getText().toString();
Intent recipientsIntent = new Intent(MainActivity.this, RecipientsActivity.class);
recipientsIntent.putExtra(ParseConstants.KEY_MESSAGE, value);
recipientsIntent.putExtra(ParseConstants.KEY_FILE_TYPE, ParseConstants.TYPE_TEXT);
startActivity(recipientsIntent);
}
});
alert.setNegativeButton("Cancel", new DialogInterface.OnClickListener() {
public void onClick(DialogInterface dialog, int whichButton) {
// Cancelled.
}
});
alert.show();
//******************************************************
break;
}
return true;
}
//return super.onOptionsItemSelected(item)
//}
@Override
public void onTabSelected(ActionBar.Tab tab,
FragmentTransaction fragmentTransaction) {
// When the given tab is selected, switch to the corresponding page in
// the ViewPager.
mViewPager.setCurrentItem(tab.getPosition());
}
@Override
public void onTabUnselected(ActionBar.Tab tab,
FragmentTransaction fragmentTransaction) {
}
@Override
public void onTabReselected(ActionBar.Tab tab,
FragmentTransaction fragmentTransaction) {
}
}
Ben Jakuben @paul stevens
5 Answers
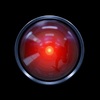
Paul Stevens
4,125 PointsThe error seems to be happening in the on create method. I'm a bit confused why it doesn't crash straight away when it is created. I would try stepping through the program using the debugger to find out exactly which path you are taking through the code that leads to that error. I would be looking for somewhere that the intent is created and called without having the extra data put into it.
Looking for this line:
startActivity(recipientsIntent);
It should have a like this before it, where a value (fileType in this case) is passed along when the intent is started:
recipientsIntent.putExtra(ParseConstants.KEY_FILE_TYPE, fileType);
I know it's not much help, sorry. I will keep thinking. When I have a bit more time I will try reading through the code and seeing if I spot anything, but I think the debugger could help a bit here.
Paul
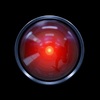
Paul Stevens
4,125 PointsHello,
This seems to be the problem. You are getting a null value on line 54 in the recipients activity. Can you paste the recipients activity and use a comment to show which is line 54 as there are no line numbers on here.
Caused by: java.lang.NullPointerException 03-28 04:20:54.431: E/AndroidRuntime(7938): at com.instantmedia.swerve.RecipientsActivity.onCreate(RecipientsActivity.java:54)
Paul :)

Fuseini Isaac
Courses Plus Student 2,225 Pointsthis is it
package com.instantmedia.swerve;
import java.util.ArrayList;
import java.util.List;
import android.app.AlertDialog;
import android.app.ListActivity;
import android.net.Uri;
import android.os.Bundle;
import android.support.v4.app.NavUtils;
import android.util.Log;
import android.view.Menu;
import android.view.MenuItem;
import android.view.View;
import android.view.Window;
import android.widget.ArrayAdapter;
import android.widget.ListView;
import android.widget.Toast;
import com.parse.FindCallback;
import com.parse.ParseException;
import com.parse.ParseFile;
import com.parse.ParseObject;
import com.parse.ParseQuery;
import com.parse.ParseRelation;
import com.parse.ParseUser;
import com.parse.SaveCallback;
public class RecipientsActivity extends ListActivity {
public static final String TAG = RecipientsActivity.class.getSimpleName();
protected List<ParseUser> mFriends;
protected ParseRelation<ParseUser> mFriendsRelation;
protected ParseUser mCurrentUser;
protected MenuItem mSendMenuItem;
protected Uri mMediaUri;
protected String mFileType;
protected String mMyMessage;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
requestWindowFeature(Window.FEATURE_INDETERMINATE_PROGRESS);
setContentView(R.layout.activity_recipients);
//show up button in action bar
setupActionBar();
getListView().setChoiceMode(ListView.CHOICE_MODE_MULTIPLE);
mMediaUri = getIntent().getData();
****************************************************************************************************************
mFileType = getIntent().getExtras().getString(ParseConstants.KEY_FILE_TYPE);
****************************************************************************************************************
mMyMessage = getIntent().getExtras().getString("themessage");
}
@Override
public void onResume() {
super.onResume();
mCurrentUser = ParseUser.getCurrentUser();
mFriendsRelation = mCurrentUser.getRelation(ParseConstants.KEY_FRIENDS_RELATION);
setProgressBarIndeterminateVisibility(true);
ParseQuery<ParseUser> query = mFriendsRelation.getQuery();
query.addAscendingOrder(ParseConstants.KEY_USERNAME);
query.findInBackground(new FindCallback<ParseUser>() {
@Override
public void done(List<ParseUser> friends, ParseException e) {
setProgressBarIndeterminateVisibility(false);
if (e == null){
mFriends = friends;
String[] usernames = new String[mFriends.size()];
int i = 0;
for (ParseUser user : mFriends) {
usernames[i] = user.getUsername();
i++;
}
ArrayAdapter<String> adapter = new ArrayAdapter<String>(
getListView().getContext(),
android.R.layout.simple_list_item_checked,
usernames);
setListAdapter(adapter);
}
else {
Log.e(TAG, e.getMessage());
AlertDialog.Builder builder = new AlertDialog.Builder(RecipientsActivity.this);
builder.setMessage(e.getMessage())
.setTitle(R.string.error_title)
.setPositiveButton(android.R.string.ok, null);
AlertDialog dialog = builder.create();
dialog.show();
}
}
});
}
private void setupActionBar(){
getActionBar().setDisplayHomeAsUpEnabled(true);
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.recipients, menu);
mSendMenuItem = menu.getItem(0);
return true;
}
@Override
public boolean onOptionsItemSelected(MenuItem item) {
switch (item.getItemId()) {
case android.R.id.home:
// This ID represents the Home or Up button. In the case of this
// activity, the Up button is shown. Use NavUtils to allow users
// to navigate up one level in the application structure. For
// more details, see the Navigation pattern on Android Design:
//
// http://developer.android.com/design/patterns/navigation.html#up-vs-back
//
NavUtils.navigateUpFromSameTask(this);
return true;
case R.id.action_send:
ParseObject message = createMessage();
if (message == null) {
// error
AlertDialog.Builder builder = new AlertDialog.Builder(this);
builder.setMessage(R.string.error_selecting_file)
.setTitle(R.string.error_selecting_file_title)
.setPositiveButton(android.R.string.ok, null);
AlertDialog dialog = builder.create();
dialog.show();
}
else {
send(message);
finish();
}
return true;
}
return super.onOptionsItemSelected(item);
}
@Override
protected void onListItemClick(ListView l, View v, int position, long id) {
super.onListItemClick(l, v, position, id);
if (l.getCheckedItemCount() > 0) {
mSendMenuItem.setVisible(true);
}
else {
mSendMenuItem.setVisible(false);
}
}
protected ParseObject createMessage(){
ParseObject message = new ParseObject(ParseConstants.CLASS_MESSAGES);
message.put(ParseConstants.KEY_SENDER_ID, ParseUser.getCurrentUser().getObjectId());
message.put(ParseConstants.KEY_SENDER_NAME, ParseUser.getCurrentUser().getUsername());
message.put(ParseConstants.KEY_RECIPIENT_IDS, getRecipientIds());
message.put(ParseConstants.KEY_FILE_TYPE, mFileType);
if (mFileType.equals(ParseConstants.TYPE_TEXT)) {
message.put("themessage", mMyMessage);
message.put(ParseConstants.KEY_FILE_TYPE, "message");
return message;
}else{
byte[] fileBytes = FileHelper.getByteArrayFromFile(this, mMediaUri);
if (fileBytes == null) {
return null;
}
else {
if (mFileType.equals(ParseConstants.TYPE_IMAGE)) {
fileBytes = FileHelper.reduceImageForUpload(fileBytes);
}
String fileName = FileHelper.getFileName(this, mMediaUri, mFileType);
ParseFile file = new ParseFile(fileName, fileBytes);
message.put(ParseConstants.KEY_FILE, file);
return message;
}}
}
protected ArrayList<String> getRecipientIds(){
ArrayList<String> recipientIds = new ArrayList<String>();
for (int i = 0; i < getListView().getCount(); i++) {
if (getListView().isItemChecked(i)){
recipientIds.add(mFriends.get(i).getObjectId());
}
}
return recipientIds;
}
protected void send(ParseObject message) {
message.saveInBackground(new SaveCallback() {
@Override
public void done(ParseException e) {
if (e == null) {
//Success!
Toast.makeText(RecipientsActivity.this, R.string.success_message, Toast.LENGTH_LONG).show();
}
else {
AlertDialog.Builder builder = new AlertDialog.Builder(RecipientsActivity.this);
builder.setMessage(R.string.error_sending_message)
.setTitle(R.string.error_selecting_file_title)
.setPositiveButton(android.R.string.ok, null);
AlertDialog dialog = builder.create();
dialog.show();
}
}
});
}
}
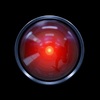
Paul Stevens
4,125 PointsWhen the intent is being created before being run, it looks like there is no value being added on as an extra KEY_FILE_TYPE.
mFileType = getIntent().getExtras().getString(ParseConstants.KEY_FILE_TYPE);
Need to find out what is calling the intent and not putting that extra bit of data in there. When does the error occur, when you press the send button after selecting recipients? Does it happen for all message types? Or you could step through the code using the debugger. to see at what is happening.
Paul :)

Fuseini Isaac
Courses Plus Student 2,225 Pointsit happens when i click on the send button.. it takes a few seconds and it crashes