Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial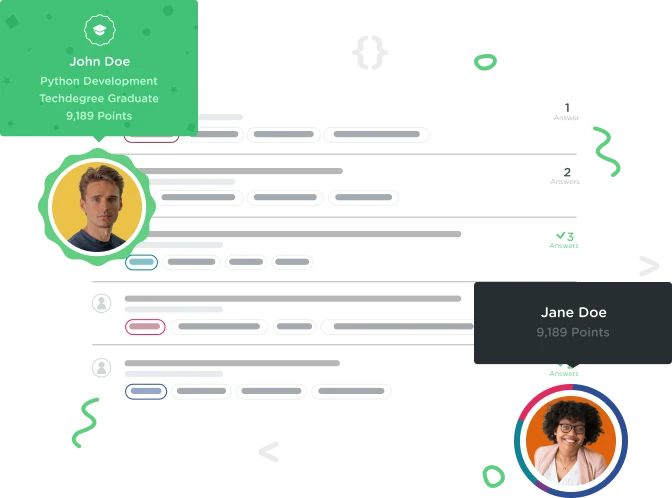
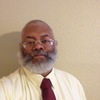
Adiv Abramson
6,919 PointsMy search method never executes
Other than displaying the prompt, my program doesn't do anything. I even added an alert(response) statement directly beneath the response = prompt() statement and nothing was displayed.
JSLint complains about an "Unexpected for". I have no clue why my code won't work at all. Can anyone advise? Thanks!
"use strict";
function search(name) {
var index = -1;
var message;
var student;
var response;
name = name.toLowerCase();
for (var i = 0; i < students.length; i++) {
if (students[i].name.toLowerCase() === name) {
index = i;
break;
}//if (students[i].name.toLowerCase() === name
}//for (var i = 0; i < students.length; i++)
if (index === -1) {
return null;
} else {
student = students[index];
}//if (index === -1)
message += "----------------------------------------------<br />";
message += "Record for student " + student.name + ":<br />";
for (var prop in student) {
message += prop.toString() + ": " + student[prop] + "<br />";
}//for (var prop in student)
message += "----------------------------------------------<br />";
print(message);
}//search()
do {
response = prompt("Search for student:");
if (response != null && response.toLowerCase() != "quit") {
search(response);
}//(response != null && response.toLowerCase() != "quit")
} while(response.toLowerCase() != "quit");
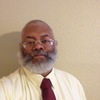
Adiv Abramson
6,919 PointsThank you, Marcus.
Here is the snapshot of my workspace: https://w.trhou.se/x77tkpr6bj
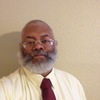
Adiv Abramson
6,919 PointsThank you so much. So I don't need to test the innerHTML property to see if it's an empty string or undefined before adding the value of the message parameter to it?
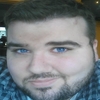
Marcus Parsons
15,719 PointsNope, because since you are setting innerHTML with some value, you don't have to check to see if innerHTML is undefined.
2 Answers
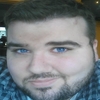
Marcus Parsons
15,719 PointsAdiv,
Here is the new snapshot of your workspace. You had a few errors in your JS files and one mistake that was causing the output to not function correctly. Comments are in both the "search.js" file and the "student_report.js" for what was changed and/or deleted. https://w.trhou.se/t6064tibax
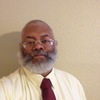
Adiv Abramson
6,919 PointsThanks so much. I didn't realize the problem arising from not placing the response variable in proper scope. And I adjusted the print() function per your suggestions. Now my code is working!
I guess the only remaining issue is that the output has the word "undefined" appearing in the top border of "-" marks that I print before writing out the student object, .e.g.:
undefined----------------------------------------------
Record for student Dave:
name: Dave
track: Front End Development
achievements: 158
points: 14730
----------------------------------------------
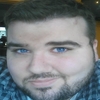
Marcus Parsons
15,719 PointsIn the snapshot, I set the message
variable equal to an empty string which eliminates that undefined
error. What happens is that message
, and any variable for that matter, by default is undefined
if it is not set with a default value. So, when you start adding string values to that variable, you get undefined
plus a string. JavaScript automatically converts values to strings when concatenation is used and undefined
is considered a value. Thus, you get "undefined---------------------" for your first string.
I got around that by setting message = '' (an empty string). So, now, when the first concatenation happens it is '' + '-----------' and not undefined
+ '------------------'. Does that make sense?
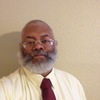
Adiv Abramson
6,919 PointsThank you, Marcus. What I don't understand is why the need for var message = ''; outside of the print() function. The message variable is the parameter of the print() function and so when print() is invoked, message is assigned the value of the argument used when calling the print() function.
I tried the following code and still have undefined appearing in the output, something that makes no sense at all to me.
function print(message) {
var outputDiv = document.getElementById('output');
var existingHTML = outputDiv.innerHTML;
if (typeof existingHTML === 'undefined' || existingHTML.length === 0) {
existingHTML = "";
}
outputDiv.innerHTML = existingHTML + message;
}
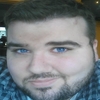
Marcus Parsons
15,719 PointsThere is a message variable and a message placeholder. The message variable is the one used in your search
function. It is the one that has to be set to an ''. It exists totally separate from the message placeholder used in your print
function. The message placeholder used in your print
function is just the data that was sent from the search
function after it was compiled. It has nothing to do with the undefined error.
Again, look at which message entity I changed in the snapshot and refer back to my comment above.
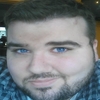
Marcus Parsons
15,719 PointsBy the way, be sure to change your print function back to the default setting except for the += operator.
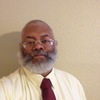
Adiv Abramson
6,919 PointsThank you, Marcus. Now I "get the message", so to speak. LOL I am used to coding in VBA where declared variables have a default value based on type. Oh well, I get the hang of this yet! Thanks!!
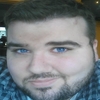
Marcus Parsons
15,719 PointsIt's no problem, Adiv. I don't mind explaining so long as a person is willing to listen. It seems like you are, so you get the picture :)
To avoid confusion in the future, I would set my placeholders in the function to be different names than any variables created. Maybe use "data" instead of "message" for the print function that way there's no confusion as to which entity you refer to.
In any case, I know you'll get the swing of it with enough practice! Happy Coding, Adiv!

Daewon Kim
15,016 PointsI ran your script, and there were some errors! ( variable "students" in your for loop is not defined, and it does not have any value)
you will need to var name = name.toLowerCase()
use document.write() instead of print() (or console.log if you prefer)
There are some other errors. Check your javascript console. it should tell you everything you are looking for.
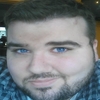
Marcus Parsons
15,719 PointsIn this course, the students
object is in another JS file, but it looks like it might not be linked correctly. Also, there is no need to put var name
because the compiler knows to use the proper variable name from the function.
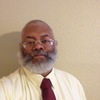
Adiv Abramson
6,919 PointsThe students array is defined in the students.js file. Why do I have to declare the name variable? It is a parameter of the search() function Why do I need to use document.write()? I thought the print() method was created by the instructor especially for use in these code chanllenges
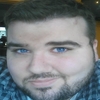
Marcus Parsons
15,719 PointsAdiv,
You do not need to declare the variable name because it is a placeholder used by the function. Also, the print()
function, by default, is used to print the current page of content. The print()
function used by the instructor is a custom function that is used to send the data to an element on the page.
Marcus Parsons
15,719 PointsMarcus Parsons
15,719 PointsHi Adiv,
I know that in this challenge the "students" object is in another js file. If you're getting an unexpected for loop error, your js files might not be linked correctly. Can you please share a snapshot of your workspace? If you don't know how to do that, here is a forum post that explains this: http://www.teamtreehouse.com/forum/workspace-snapshots