Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial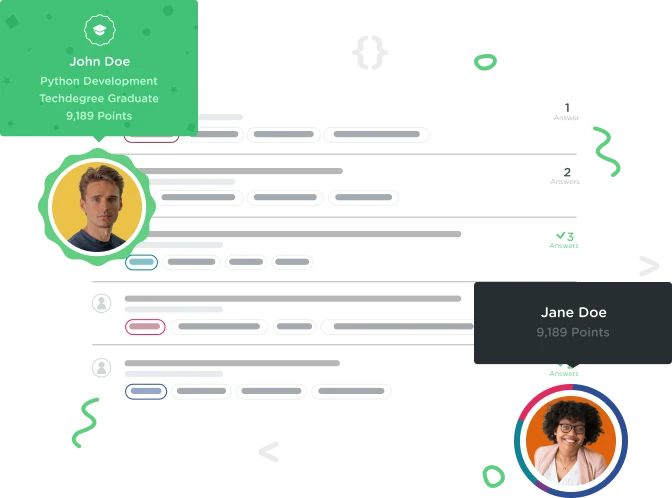
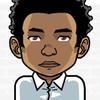
webdesignertroy
Front End Web Development Techdegree Graduate 14,718 PointsMy small circles won't pulse correctly (Hacking the Firefox Glitch)
My small circles won't pulse. the scale is not working correctly although the rules concerning the pulse keyframe and animation are written correctly:
pulse keyframe
@keyframes pulse {
50% {
transform: scale(1.4);
}
}
pulse animation
.star circle {
animation: pulse 0.7s 1.5s;
}
I suspect that it's my setup that's ruining things somewhere. Below is my entire code.
index.html
<!DOCTYPE html>
<html>
<head>
<title>Badge Animation</title>
<link rel="stylesheet" href="css/style.css">
</head>
<body>
<svg class="badge" xmlns="http://www.w3.org/2000/svg" height="440" width="440" viewBox="-40 -40 440 440">
<circle class="outer" fill="#F9D535" stroke="#fff" stroke-width="8" stroke-linecap="round" cx="180" cy="180" r="157"/>
<circle calss="inner" fill="#DFB828" stroke="#fff" stroke-width="8" cx="180" cy="180" r="108.3"/>
<path class="inline" d="M89.4 276.7c-26-24.2-42.2-58.8-42.2-97.1 0-22.6 5.6-43.8 15.5-62.4m234.7.1c9.9 18.6 15.4 39.7 15.4 62.2 0 38.3-16.2 72.8-42.1 97" stroke="#CAA61F" stroke-width="7" stroke-linecap="round" fill="none"/>
<g class="star">
<path fill="#F9D535" stroke="#fff" stroke-width="4" stroke-linecap="round" stroke-linejoin="round" d="M180 107.8l16.9 52.1h54.8l-44.3 32.2 16.9 52.1-44.3-32.2-44.3 32.2 16.9-52.1-44.3-32.2h54.8z"/>
<circle class="circle" fill="#DFB828" stroke="#fff" stroke-width="4" stroke-linecap="round" stroke-linejoin="round" cx="180" cy="107.8" r="4.4"/>
<circle fill="#DFB828" stroke="#fff" stroke-width="4" stroke-linecap="round" stroke-linejoin="round" cx="223.7" cy="244.2" r="4.4"/>
<circle fill="#DFB828" stroke="#fff" stroke-width="4" stroke-linecap="round" stroke-linejoin="round" cx="135.5" cy="244.2" r="4.4"/>
<circle fill="#DFB828" stroke="#fff" stroke-width="4" stroke-linecap="round" stroke-linejoin="round" cx="108.3" cy="160.4" r="4.4"/>
<circle fill="#DFB828" stroke="#fff" stroke-width="4" stroke-linecap="round" stroke-linejoin="round" cx="251.7" cy="160.4" r="4.4"/>
</g>
</svg>
</body>
</html>
css/style.css
/* --------------------------
Base
--------------------------- */
body {
background: #8069a1;
padding-top: 60px;
}
svg {
margin: auto;
display: block;
}
/* --------------------------
Keyframes
--------------------------- */
@keyframes grow {
0% {
transform: scale(0);
}
30% {
transform: scale(1.1);
}
60% {
transform: scale(0.9);
}
}
@keyframes turn {
0% {
transform: rotate(0) scale(0);
opacity: 0;
}
60% {
transform: rotate(375deg) scale(1.1);
}
80% {
transform: rotate(355deg) scale(0.9);
}
100% {
transform: rotate(360deg) scale(1);
}
}
@keyframes pulse {
50% {
transform: scale(1.1);
}
}
/* --------------------------
SVG Styles
--------------------------- */
.badge * {
transform-origin: 180px 180px;
}
.outer,
.inner,
.inline {
animation: grow 1s ease-out backwards;
}
.inner {
animation-delay: .1s;
}
.inline {
animation-delay: .15s;
}
.star{
animation: turn 1.1s .2s ease-out backwards;
}
.star circle {
animation: pulse 0.7s 1.5s;
}
.star circle:nth-of-type(2){
animation-delay: 1.6s;
}
.star circle:nth-of-type(3){
animation-delay: 1.7s;
}
.star circle:nth-of-type(4){
animation-delay: 1.8s;
}
.star circle:nth-of-type(5){
animation-delay: 1.9s;
}
7 Answers

James Anwyl
Full Stack JavaScript Techdegree Graduate 49,960 PointsManaged to get it working in both Chrome and Firefox by using the -moz- vendor prefix. I had to apply transform-origin to individual circles like webdesignertroy did.
.badge * {
transform-origin: 50% 50%;
-moz-transform-origin: 180px 180px;
}
.star circle {
animation: pulse .7s 1.5s;
-moz-transform-origin: 180px 107.8px;
}
.star circle:nth-of-type(2) {
animation-delay: 1.6s;
-moz-transform-origin: 223.7px 244.2px;
}
.star circle:nth-of-type(3) {
animation-delay: 1.7s;
-moz-transform-origin: 135.5px 244.2px;
}
.star circle:nth-of-type(4) {
animation-delay: 1.8s;
-moz-transform-origin: 108.3px 160.4px;
}
.star circle:nth-of-type(5) {
animation-delay: 1.9s;
-moz-transform-origin: 251.7px 160.4px;
}
Apologies for the late reply but thought someone might find this useful.
Hope this helps :)
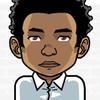
webdesignertroy
Front End Web Development Techdegree Graduate 14,718 PointsI set badge * { transform-origin: 180px 180px }
because I wanted to circumvent the Firefox glitch. It looks like I have to further set up a unique transform-origin
rule for each individual circle inside the <g> element if I wish to use pure css. Since I'm not a css purist, I will probably use JavaScript to set the transform-origin
rule for my future projects.
In the following, using Chrome's Inspector, I found all the origin points of the small circles and added a unique transform-origin
rule for each:
.star circle {
animation: pulse 0.7s 1.5s;
transform-origin: 180px 107.8px;
}
.star circle:nth-of-type(2){
animation-delay: 1.6s;
transform-origin: 233.7px 244.2px;
}
.star circle:nth-of-type(3){
animation-delay: 1.7s;
transform-origin: 135.5px 244.2px;
}
.star circle:nth-of-type(4){
animation-delay: 1.8s;
transform-origin: 108.3px 160.4px;
}
.star circle:nth-of-type(5){
animation-delay: 1.9s;
transform-origin: 251.7px 160.4px;
}
(If no one objects to this answer, I'm marking it as Best Answer. (Even though marking my own answer as 'best answer' is equivalent to giving myself a high-five.))

john Shi
1,791 PointsThe problem with using Javascript is that it can be switched off, along with your animations.
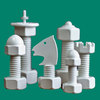
Steven Parker
229,771 PointsThe small circles do pulse, but by moving out and back instead of expanding and contracting.
This is because you have set set transform origin to a specific point, instead of the relative position used in the video:
.badge * {
transform-origin: 50% 50%;
}
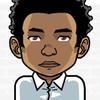
webdesignertroy
Front End Web Development Techdegree Graduate 14,718 PointsI want to use transform-origin: 180px 180px
to account for the Firefox glitch. Do I need to reset an extra transform-origin
rule within each .star circle
target?
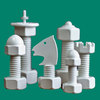
Steven Parker
229,771 PointsSee if this setting fixes the issue without using explicit separate locations:
.badge * {
transform-origin: center;
transform-box: fill-box;
}
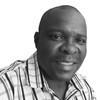
Mark Mangunda
16,652 PointsFirstly, I would thank James Anwyl and Dusan Zivanovic on their valued input. I would like to make the following observations:
- By effecting the grow CSS codes on the SVG image, which was given the class 'badge' & the universal selector(*). I see that the elements with the classes outer, inner & inline are responsive. Yet the class 'star' elements do not. The responsive classes in the HTML have common cx & cy values of '180'. While in the 'star' class they vary, and as such behaviours independently. The transform-origin property pivots responsive elements at the center (50%,50%), but the 'star' class elements are pivoted at the bottom-right point of the star observed on rotation. Thus, does it mean CSS or the browser like chrome effects changes on elements with a common pattern?
- By treating the star class elements as independent from the badge class, we are able to see that by applying transform-origin of (40%,40%) we get a perfect star 360 deg rotation similar to that shown in the video. Likewise, if we do the same with the smaller circles, by allocating them their independent transform-origin cx & cy (px) values they remain rooted or pivoted to the center.
Why does the star pivot at 40% not 50%, is it mathematical, CSS or the browser?

rays0
16,293 PointsSome svg shape elements doesn't have a defined center x and y values, like on the next challenge. There's a clarification of the problem and semi-solution here:
http://stackoverflow.com/questions/40896007/svg-transform-origin-problems-in-firefox
Read the 12/1/16 post by 'myf'
I say semi-solution, although the current release of Firefox (1/5/17) doesn't have the 'svg.transform-box.enabled' set to true in about:config; On Firefox Dev Edition, it's enabled by default. So, this might be enabled by default on future Firefox mainline releases, and possibly only require the transform-box: fill-box; property and value for the svg element.
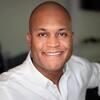
Larry Rhodes
Front End Web Development Techdegree Student 12,046 PointsGreat answers! I tried using explicit locations for each circle first, but I love the simplicity of setting the transform-origin and transform-box properties to "center" and "fill-box" respectively. Both worked. Thanks!

john Shi
1,791 Points2022 here and Steven Parker's badge amendment worked for me.