Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial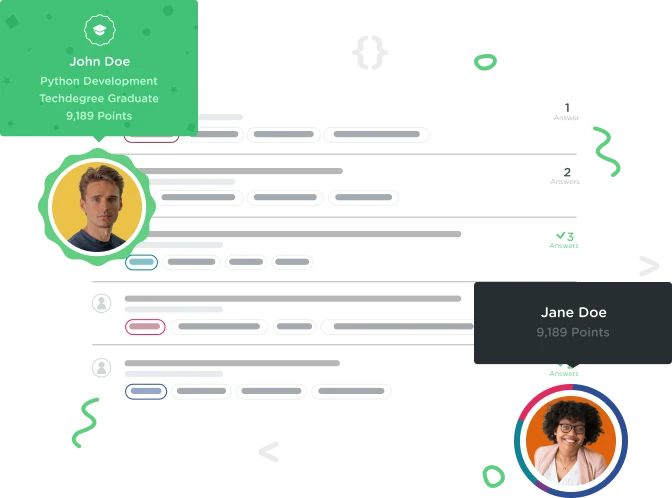
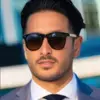
Eddie Boscana
6,966 PointsMy solution
// Step 1: Collect user input let userHighInput = prompt("Enter the highest number for the random range:"); let userLowInput = prompt("Enter the lowest number for the random range:");
// Step 2: Convert the input to a number let maxNumber = Number(userHighInput); let lowNumber = Number(userLowInput); // Fixed typo here
// Check if the input is valid numbers and maxNumber is greater than lowNumber if (isNaN(maxNumber) || isNaN(lowNumber) || maxNumber <= lowNumber) { alert("Please enter valid numbers with max number greater than low number."); } else { // Step 3: Generate a random number from lowNumber to maxNumber let randomNumber = Math.floor(Math.random() * (maxNumber - lowNumber + 1)) + lowNumber;
// Step 4: Display the random number
alert("Your random number is: " + randomNumber); // using alert
console.log("Your random number is:", randomNumber); // using console.log
// Or to display it on the webpage, assuming there's an element with id 'output'
document.getElementById('output').textContent = "Your random number is: " + randomNumber;
}
Step 1: Collect user input
let userHighInput = prompt("Enter the highest number for the random range:"); let userLowInput = prompt("Enter the lowest number for the random range:");
This snippet presents two prompt dialog boxes to the user. The first one asks for the highest number and the second asks for the lowest number. Step 2: Convert the input to a number
let maxNumber = Number(userHighInput); let lowNumber = Number(userLowInput);
The entered strings are then converted to numbers through the Number() function. Input validation
if (isNaN(maxNumber) || isNaN(lowNumber) || maxNumber <= lowNumber) { alert("Please enter valid numbers with max number greater than low number."); }
The isNaN() function checks whether the converted inputs are Not-a-Number. If so, or if the entered maximum number is less than or equal to the minimum number, then an alert message is sent telling the user to enter valid numbers. Step 3: Generate a random number
else { let randomNumber = Math.floor(Math.random() * (maxNumber - lowNumber + 1)) + lowNumber; }
If the inputs pass the check, a random number is generated within the user defined range. Math.random() generates a random decimal number from 0 (inclusive) to 1 (exclusive). It's being multiplied by (maxNumber - lowNumber + 1), to shift the range from [0 – 1) to [0 – maxNumber-lowNumber+1). The result is then floored to the nearest whole number with Math.floor(), making the upper bound (maxNumber) achievable. Finally adding the lowNumber shifts this range to [lowNumber – maxNumber]. Step 4: Display the random number
alert("Your random number is: " + randomNumber); // using alert
console.log("Your random number is:", randomNumber); // using console.log
// Or to display it on the webpage, assuming there's an element with id 'output'
document.getElementById('output').textContent = "Your random number is: " + randomNumber;
}
The random number is then displayed to the user in 3 different ways: using the alert dialog window, printed to the browser's console with console.log, and inserted on the webpage by finding the HTML element with the id output and setting its textual content to display the randomized number.