Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial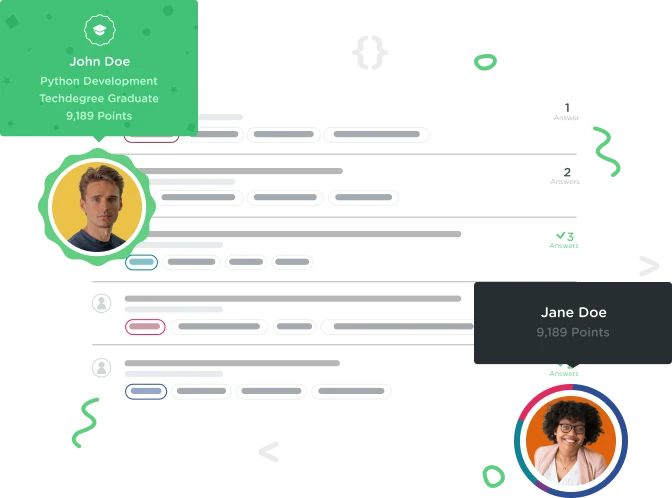
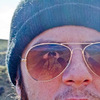
Dan MacDonagh
4,615 PointsMy solution
So this was my solution, it all seems to work. I didn't end up using the quiz_ui.js file because it confused me to break apart the quiz logic.
Submitting this before watching the next video to see how Andrew did it.
My issue is always "Alright, this works, but is it the best way to do it?"
// Grab the DOM elements we need to interact with
var quizWrapper = document.getElementById('quiz');
var questionElement = document.getElementById('question');
var firstAnswerText = document.getElementById('choice0');
var secondAnswerText = document.getElementById('choice1');
var firstAnswerButton = document.getElementById('guess0');
var secondAnswerButton = document.getElementById('guess1');
var quizStatus = document.getElementById('progress');
// Create a constructor function to build a quiz
function Quiz() {
this.score = 0;
this.currentQuestion = 0;
this.questions = [];
}
//Add functionality to add questions to the quiz. Loops over each argument passed into the addQuestion method and pushes them to the questions array
Quiz.prototype.addQuestion = function() {
for(var i = 0; i < arguments.length; i++) {
this.questions.push(arguments[i]);
}
}
// Logic to see if the submitted answer is the correct one. Writes the next question to the quiz, or clears the quiz and displays the final score in the status
Quiz.prototype.submitAnswer = function(answer) {
console.log('Submitted ' + 1 + ', right answer is ' + this.questions[this.currentQuestion].rightAnswer);
if (answer === this.questions[this.currentQuestion].rightAnswer) {
this.score++;
this.currentQuestion++;
} else {
this.currentQuestion++;
}
if(this.currentQuestion < this.questions.length) {
this.renderQuiz();
} else {
this.clearUI();
quizWrapper.removeChild(firstAnswerButton);
quizWrapper.removeChild(secondAnswerButton);
quizStatus.innerHTML = 'End of the quiz! Your score is ' + this.score + ' out of ' + this.questions.length;
}
}
// Seperate method to clear out the questions, answers, and status in preperation for a new question, or the end of the quiz
Quiz.prototype.clearUI = function() {
questionElement.innerHTML = '';
firstAnswerText.innerHTML = '';
secondAnswerText.innerHTML = '';
quizStatus.innerHTML = '';
}
//Method to display the next question and update the status if there are questions left
Quiz.prototype.renderQuiz = function() {
this.clearUI();
if (this.currentQuestion < this.questions.length) {
questionElement.innerHTML = this.questions[this.currentQuestion].question;
firstAnswerText.innerHTML = this.questions[this.currentQuestion].firstAnswer;
secondAnswerText.innerHTML = this.questions[this.currentQuestion].secondAnswer;
quizStatus.innerHTML = 'Question ' + (this.currentQuestion + 1) + ' of ' + this.questions.length;
}
}
// Consctructor function to build questions
function Question(question, firstAnswer, secondAnswer, rightAnswer) {
this.question = question;
this.firstAnswer = firstAnswer;
this.secondAnswer = secondAnswer;
this.rightAnswer = rightAnswer;
}
// Create new instance of the Quiz object
var newQuiz = new Quiz();
// Declare some questions, first argument is the question, second is the first answer, third is the second answer, and fourth is the answer thats correct
var firstQuestion = new Question('How much is 12+12?', '24', '26', 1);
var secondQuestion = new Question('Where is Seattle Located?', 'Oregon', 'Washington', 2);
var thirdQuestion = new Question('How many days in a year?', '314', '365', 2);
var fourthQuestion = new Question('How many months are there?', '12', '13', 1);
//Push all questions into the quiz's question array
newQuiz.addQuestion(firstQuestion, secondQuestion, thirdQuestion, fourthQuestion);
//First render of the quiz, displays the first question
newQuiz.renderQuiz();
//Ran if the first answer button is clicked
firstAnswerButton.onclick = function() {
newQuiz.submitAnswer(1);
}
//Ran if the second button is clicked
secondAnswerButton.onclick = function() {
newQuiz.submitAnswer(2);
}
2 Answers
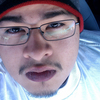
Chyno Deluxe
16,936 PointsNice and clean...great job.

Eric Hutchison
4,791 PointsSomehow looking at this answer helped me understand OOP more than the last 4 hours of re-watching this course. Great job marking every step!