Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial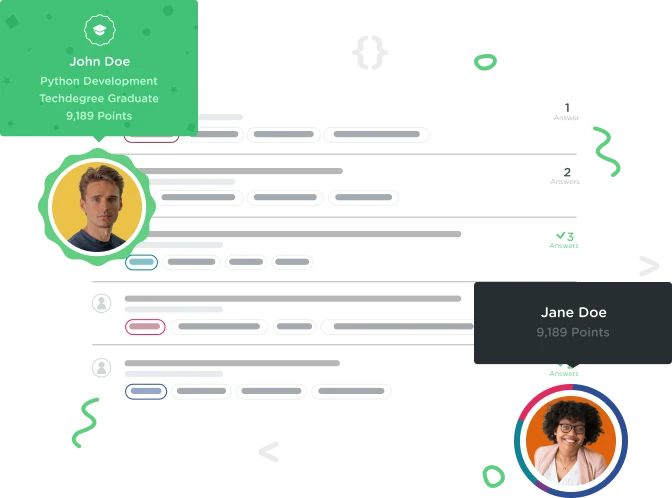
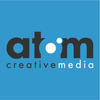
Alex Connor
2,809 PointsMy solution
I approached this problem from a bit of a different angle, however it still works as expected. I guess this shows that there's more than one way to code! I think as long as the solution is (at least) generally efficient, is well formatted, and also makes sense to others then there are no wrong answers.
I challenged myself to ask questions that would provide different types of answers such as numbers and strings, and also that would make use of different comparison operators.
Only thing I think I missed out on was using:
score += 1
...instead of...
score = score + 1
Need to remember that one!
Here's how I did it:
// Start with a score of zero
var score = 0;
// Ask all the questions, storing the answers in new variables
var q1 = prompt("How many hours are there in a day?");
var q2 = prompt("Pick any number from 1 to 10.");
var q3 = prompt("Type either \"A\" or \"B\"");
var q4 = prompt("Is London the capital of England?");
var q5 = prompt("What is 10 + 10?");
// Check the first answer is correct.
if(parseInt(q1) === 24) {
// If the answer is correct, add 1 to the score.
score = score + 1;
}
/*
Check the second answer is correct.
We're checking that the answer is both more than (or equal to) 1,
AND that 10 is more than (or equal to) the answer.
IF this is true, add 1 to the score variable.
*/
if(parseInt(q2) >= 1 && 10 >= parseInt(q2)) {
score = score + 1;
}
/*
Check the third answer is correct.
We're checking that the answer is simply either "A" OR "B".
IF this is true, add 1 to the score variable.
*/
if(q3 === "A" || q3 === "B") {
score = score + 1;
}
/*
Check the fourth answer is correct.
We're converting the answer to uppercase, and then allowing for a couple of different answers.
IF this is true, add 1 to the score variable.
*/
if(q4.toUpperCase() === "YES" || q4.toUpperCase() === "Y" || q4.toUpperCase() === "YES IT IS") {
score = score + 1;
}
/*
Check the fifth answer is correct.
We're looking for an answer of 20 (integer) OR "TWENTY" (string).
IF this is true, add 1 to the score variable.
*/
if(parseInt(q5) === 20 || q5.toUpperCase() === "TWENTY") {
score = score + 1;
}
// Create an empty new variable for the crown prize.
var crown;
// IF they didn't score anything (score is zero), write it to the document.
if (score === 0) {
document.write("<p>Your score was " + score + ". You didn't win anything this time.</p>");
// ELSE if the score WASN'T zero, set the prize based on the score value.
} else {
// IF the score is less than (or equal to) 2, the crown variable is changed to "Bronze".
// Don't worry if the score was zero - that would have been handled in the first statement, and we wouldn't have gotten to this one.
if(score <= 2) {
crown = "Bronze";
// ELSE IF the score is less than (or equal to) 4, the crown variable is changed to "Silver".
} else if(score <= 4) {
crown = "Silver";
// ELSE IF the score is equal to 5, the crown variable is changed to "Gold".
} else if(score === 5) {
crown = "Gold";
}
// Write the winner's message to the document, telling them their final score along with their prize (using the crown variable).
document.write("<p>Your score was " + score + "! You win the " + crown + " Crown!</p>");
}
1 Answer
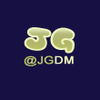
Jonathan Grieve
Treehouse Moderator 91,253 PointsGreat job, Alex.
And yes, don't worry if you miss certain things from time to time. You'll be doing that and looking things up throughout your programming career. The only way to improve is to keep coding! Good luck with it. :)