Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial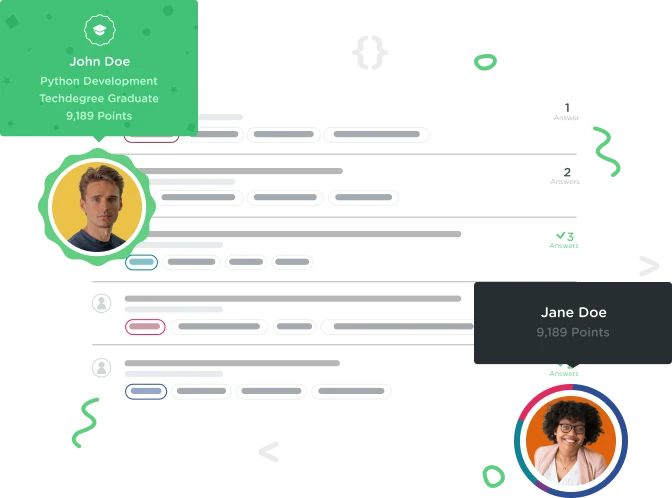

Alexandru Vlasnita
5,542 PointsMy solution
I'd like to get some feedback on this, is this good, is it bad, is it overkill? I really find it easier to understand than the video solution, but it's just me, maybe it's actually harder.
let answer;
let wrong = [];
let right = [];
let questionList = [
[`In what city is the Effeil Tower?`, `paris`],
[`Capital of Englang?`, `london`],
[`What's 2 + 2?`, `4`],
[`What is my name?`, `alex`]
]
function print(message) {
document.write(message);
}
function askQuestion(questions) {
for(let i = 0; i < questions.length; i += 1) {
answer = prompt(questionList[i][0]);
if(answer.toLowerCase() === questionList[i][1]) {
right.push(questionList[i][0]);
} else {
wrong.push(questionList[i][0])
}
}
}
function printAnswered(answered) {
let list = '<ol>';
for (let i = 0; i < answered.length; i += 1) {
list += `<li> ${answered[i]} </li>`
}
list += '</ol>';
print(list);
}
askQuestion(questionList);
print(`<p>You got ${right.length} answers right!</p>`);
print(`<b>You got these right:</b>`);
printAnswered(right);
print(`<b>You got these wrong:</b>`);
printAnswered(wrong);
1 Answer
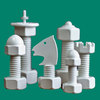
Steven Parker
231,275 PointsIt's probably not a good habit to put all strings in template literals. But using them where interpolation is being done does give the code a slightly cleaner look.
Alexandru Vlasnita
5,542 PointsAlexandru Vlasnita
5,542 PointsI’ve been thinking about that, is there any case where they could cause issues?
Steven Parker
231,275 PointsSteven Parker
231,275 PointsI doubt it would cause anything serious, but it might be less efficient since the engine would need to scan the template for substitution tokens.