Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial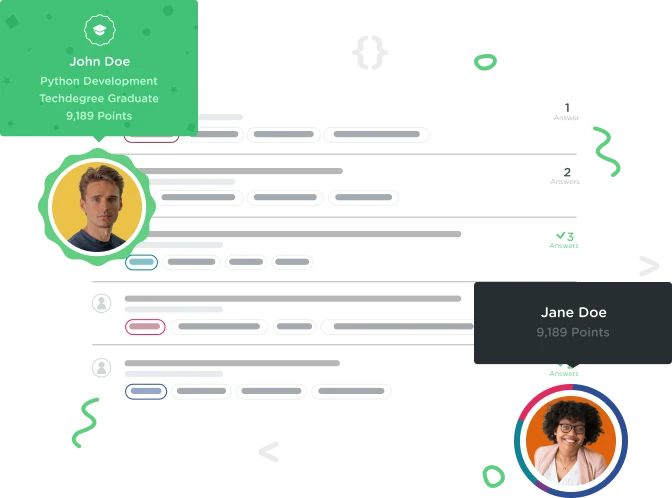

Travis Williams
3,063 PointsMy solution adding clearer error messages and an additional check.
I wasn't able to come up with the math for the random number generator using two numbers without seeing the answer.
However, I added individual checks for each number prompt to provide more clear errors and an additional check to ensure the first number is lower than the second number.
// Collect input from a user
const userLow = prompt("Please input a number.");
const userHigh = prompt("Now input a higher number.");
// Convert the input to a number
lowNum = parseInt(userLow);
highNum = parseInt(userHigh);
console.log(lowNum, highNum);
console.log(isNaN(lowNum), isNaN(highNum));
// validate that both inputs are numbers
if ( isNaN(lowNum) ) {
alert(`Please only enter numbers. ${userLow} is not a number. Reload and try again.`);
} else if ( isNaN(highNum) ){
alert(`Please only enter numbers. ${userHigh} is not a number. Reload and try again.`);
} else if (highNum < lowNum) {
// validate the 2nd number is higher than the 1st
alert(`Your second number, ${userHigh}, was not higher than your first, ${userLow}. Please reload and try again.`);
} else {
// Use Math.random() and the user's numbers to generate a random number
const rand = Math.floor(Math.random() * (highNum - lowNum + 1)) + lowNum;
console.log(rand);
// Create a message displaying the random number
const message = `
<h2>${rand} is a random number between ${lowNum} and ${highNum}.
</h2>`;
document.querySelector('main').innerHTML = message;
}