Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial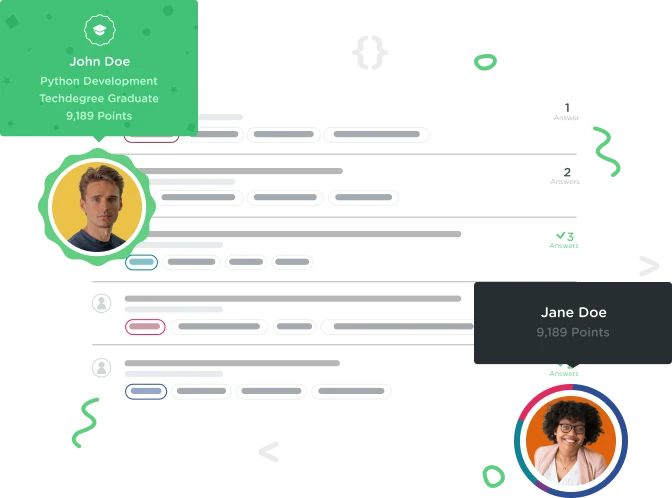

Anthony Branze
4,887 PointsMy solution (Feedback welcome Please, All critisism is considered constructive and be brutally honest.)
Here is My solution to the challenge. Took me two days to hone it all in but it works.
// initial score
var answer = 0;
// asking questions
var question1 = prompt("What are the similarities of Java & JavaScript?");
if (question1.toUpperCase() === "NONE") {
answer+= 1;
}
var question2 = prompt("What language was named after a gem?");
if (question2.toUpperCase() === "RUBY") {
answer+= 1;
}
var question3 = prompt("What syntax style is JavaScript?");
if (question3.toUpperCase() === "C FAMILY") {
answer+= 1;
}
var question4 = prompt("What is OOP?");
if (question4.toUpperCase() === "OBJECT ORIENTED PROGRAMMING"){
answer+= 1;
}
var question5 = prompt("Who is the creater of JavaScript?");
if(question5.toUpperCase() === "BRENDAN EICH"){
answer+= 1;
}
/*
Display final score
*/
var finale = alert("Congratulations you answered " + answer + " questions correctly!");
/*
Ranking the player based on score.
*/
if (answer === 5) {
document.write("<p>Congratulations! You've earned the Golden Crown!!</p>");
} else if (answer <= 4 && answer >= 3) {
document.write("<p>Congratulations! You've earned the Silver Crown!!</p>");
} else if (answer <= 2 && answer >= 1) {
document.write("<p>Congratulations! You've earned the Bronze Crown!!</p>");
} else if (answer === 0) {
document.write("<p><b>LOSER!</b></p>");
}
// debuging purposes
console.log(answer);

Anthony Branze
4,887 Pointscasthrademosthene I used the && (And Operator) because I wanted my expression to evaluate to true if One of the two were true. if I used the || (Or operator) the computer would skip that code block because of the OR but with && (And Operator) it will execute the code block with just needing One of the conditions not both. I am still learning. But that is my thought process. Please correct me if I'm wrong.
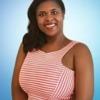
casthrademosthene
6,275 PointsYour answer makes sense, I'm also learning and that why I asked the question. So thank you for explaining why you used the && instead of the ||. It helped me understand the difference between the two of them in regards to which one to use best. When I originally did the challenge I used the || and it worked out ok, but after reading your comment I realized that although it worked ok in that situation, that using the && would of been better.
5 Answers
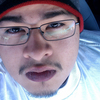
Chyno Deluxe
16,936 PointsGreat Job Anthony!
Here are a few things I would change from your code. Considering you are in Basic Javascript I won't give you anything advanced but here is a way to shorten your code.
//the added variable is not neccessary here and will still run without it.
alert("Congratulations you answered " + answer + " questions correctly!");
/*
Ranking the player based on score.
*/
if (answer === 5) {
document.write("<p>Congratulations! You've earned the Golden Crown!!</p>");
} else if (answer >= 3) { //answer can be 3 or 4 to get Silver
document.write("<p>Congratulations! You've earned the Silver Crown!!</p>");
} else if (answer >= 1) { //answer can be 1 or 2 to get Bronze
document.write("<p>Congratulations! You've earned the Bronze Crown!!</p>");
} else if (answer === 0) {
document.write("<p><b>LOSER!</b></p>");
}
// debuging purposes
console.log(answer);
I hope this helps.

mike sanford
2,389 PointsQuestion.I thought you need to have at the end of the if () {} , else if (){}a "else {}" i only see for answer the 'else if 'all the way to 0 loser. Hope that question makes sense curious of the answer.
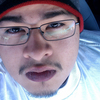
Chyno Deluxe
16,936 PointsHey Mike!
Yes your question did make sense. It is not necessary for if statements to have a catch all else statement, however we could replace the final else if to an else and it would still work.
if (answer === 5) {
document.write("<p>Congratulations! You've earned the Golden Crown!!</p>");
} else if (answer >= 3) { //answer can be 3 or 4 to get Silver
document.write("<p>Congratulations! You've earned the Silver Crown!!</p>");
} else if (answer >= 1) { //answer can be 1 or 2 to get Bronze
document.write("<p>Congratulations! You've earned the Bronze Crown!!</p>");
} else {
document.write("<p><b>LOSER!</b></p>");
}
// debuging purposes
console.log(answer);
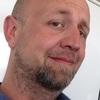
Shawn Denham
Python Development Techdegree Student 17,801 PointsNot sure what you want us to be brutally honest about? Does it do what it's supposed to do? Then everything is perfect :)

Anthony Branze
4,887 PointsLol I mean, If theres things I should have shortend, If I should have made questions an array of objects instead of doing this way? Should I have done switches instead of if else? lol Idk I am probably just over analyzing everything and jumping way further ahead than I need to in terms of complexity. i mean it is basic course. not advanced algorithm scripting. lol. It does work. and I dont repeat myself. It looks clean and clear to me. But I guess I am looking for validation from someone more experienced than myself.
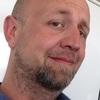
Shawn Denham
Python Development Techdegree Student 17,801 PointsI was just simply saying good job on writing a well documented app! :) There is always ways to write code "cleaner" or DRYer, but when people are learning it is more important that the code works! Writing more compact and dryer code will come with time.
Some may argue but I think that putting the questions in an array would not have saved you much in the way of typing, but imho making the ask the question part a function that pulls the questions from an array would make your application more dynamic. All you would need to do is just add a new Array if you wanted different questions.
A good exercise would be to build a quiz builder. Prompt the user and see if they want to run a pre-built quiz or if they would like build a quiz.
Display a prompt asking them to enter in their questions then store those questions in an Array (or an object if you have gotten that far...if not build it as an Array then change it over to objects once you go though that part of the course!)
Once that is done prompt the user if they would like to take their quiz.
That is the kind of stuff I did when I was learning. I would take what teacher assigned and get that working then get I would get it as DRY as I could. Once that was done I would start adding "features". Then as we went through the course I would update my app with new features that took advantage of what I had learned.
Sorry that was so longwinded and I kind of got off track there :)
But honestly your code looks fantastic! Great Job!
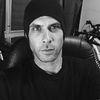
jack AM
2,618 PointsI think your work looks good! Although, is the variable finale really needed? You could have easily displayed the alert message without the need to create a new variable...imo :)

Anthony Branze
4,887 PointsThank you jack! So my thoughts behind the var finale, are for some reason I thought I had to store the alert in the variable, I guess it was just purely reflex.
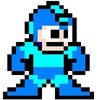
Robert Richey
Courses Plus Student 16,352 PointsHi Anthony,
Nice work! Here is an alternate way of writing your quiz, though not necessarily shorter. These questions can handle more than a single answer. I'm not presenting this as "the best way". I wanted to show something different that you might learn from.
var score = 0;
var quiz = [
{
question: "What are the similarities of Java & JavaScript?",
answer: ["none", "coffee"]
},
{
question: "What language was named after a gem?",
answer: ["ruby"]
},
{
question: "What syntax style is JavaScript?",
answer: ["c family", "c style"]
},
{
question: "What is OOP?",
answer: ["object oriented programming"]
},
{
question: "Who is the creater of JavaScript?",
answer: ["brendan eich"]
}
];
quiz.forEach(function(obj) {
var q = obj.question;
var a = obj.answer;
if (a.indexOf(prompt(q).toLowerCase()) >= 0) { ++score; }
});
function displayResult() {
var crown;
var p = document.createElement("p");
document.body.appendChild(p);
switch (score) {
case 0:
p.textContent = "LOSER!";
return;
case 1:
case 2:
crown = "Bronze Crown";
break;
case 3:
case 4:
crown = "Silver Crown";
break;
case 5:
crown = "Gold Crown";
}
p.textContent = "Congratulations! You've earned the " + crown + "!!";
}
displayResult();
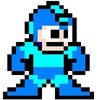
Robert Richey
Courses Plus Student 16,352 PointsAnother way to iterate through the quiz might be with the .reduce()
method.
score = quiz.reduce(function(acc, obj) {
var q = obj.question;
var a = obj.answer;
var res = prompt(q).toLowerCase();
return a.indexOf(res) >= 0 ? ++acc : acc;
}, 0);

Anthony Branze
4,887 PointsRobert Thank you!! I had been thinking about several ways to come to the objective. using an array and switch satements I just didnt really know how to visualize it. Thank you! I am most definitely learning from it :)

Aaron Graves
1,849 PointsThanks for posting your code. It actually showed me a way of doing it that I couldn't figure out. I was having trouble figuring out how to write the Javascript for keeping track of how many questions were answered correctly. It helped me. I typically don't like looking at answers unless I have no idea how to proceed. It made total sense though.
casthrademosthene
6,275 Pointscasthrademosthene
6,275 Pointsquick question I noticed that you used the && wouldn't it have been easier to use the || instead. I'm just curious