Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial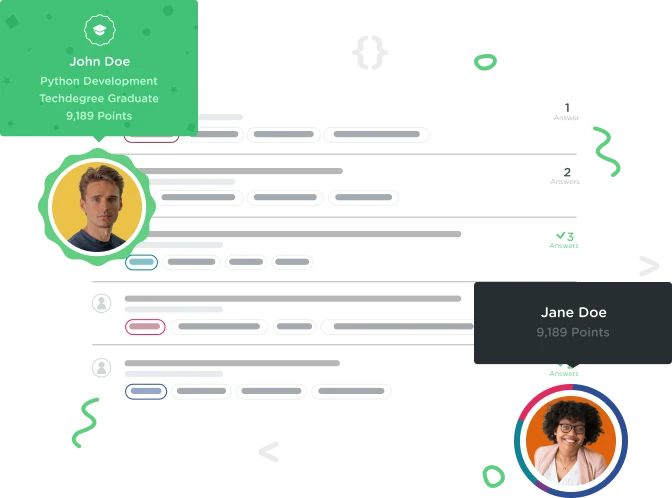
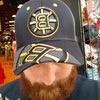
Ammon D'Addabbo
7,866 PointsMy solution for random number challenge
alert('Welcome to my random number generator. Choose a bottom and top number and the random number will fall within those limitations you provide!');
var bottomNumber = parseInt(prompt('Choose a bottom number.'));
var topNumber = parseInt(prompt('Choose a top number.'));
var rndmNumber = Math.floor(Math.random() * (topNumber - bottomNumber + 1)) + bottomNumber;
document.write(rndmNumber);
3 Answers
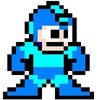
Robert Richey
Courses Plus Student 16,352 PointsI love seeing what everyone writes for the random number challenge, as it gets me to re-consider how I might approach re-writing this for myself.
First of all, thanks for sharing and great job! Your code works as expected. I remember the first time doing this, I got a little stuck trying to figure out how to generate the random value based on a range supplied by the user.
Lately, I've been a fan of creating a function for generating the random number. Here is my current take:
var bottomNumber = parseInt(prompt('Choose a bottom number.'));
var topNumber = parseInt(prompt('Choose a top number.'));
function rng(min, max) {
var min = Number(min) || 0;
var max = Number(max) || 1;
if (min > max) {
var temp = min;
min = max;
max = temp;
}
if (min === 0 && max === 1) {
return Math.random();
} else {
var range = max - min;
return Math.floor(Math.random() * (range + 1)) + min;
}
}
var rndmNumber = rng(bottomNumber, topNumber);
document.write(rndmNumber);
Hope this helps in some way. Feel free to ask if you have any questions.
Cheers
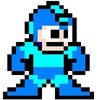
Robert Richey
Courses Plus Student 16,352 PointsI just found a really cool article on stackoverflow How to swap two variables, to replace the swap logic and wanted to share it.
if (min > max) {
max = [min, min = max][0];
}
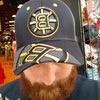
Ammon D'Addabbo
7,866 PointsRobert, This was my first post ever and didn't know about the Markdown, so thank you very much for letting me know! Thank you for sharing your function for generating random numbers, I'm new to JS so I'm going to take my time and dissect it so I can absorb as much as I can from it. Once again, thanks for your help Robert, and for the link to the article!
-Ammon
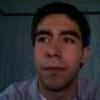
David Trejo
19,914 PointsRobert, Can you please explain how the interpreter is executing the syntax max = [min, min = max][0];
I don't quite get the grasp of how the numbers of being swapped the way the syntax is written.
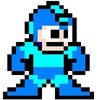
Robert Richey
Courses Plus Student 16,352 PointsI don't have a deep understanding of the interpreter. I found this swap code posted by someone else in a stackoverflow thread. I'll try to share what I think is happening.
Disclaimer: I'm making an educated guess here
To provide a useful example, let's give min and max some values.
var min = 10
var max = 5
When the interpreter gets to this chunk [min, min = max]
, it first populates the 0 index with the current value of min, which is 10.
[10, min = max]
Next, the current value of max is assigned to min and that value is stored at index 1.
[10, min = 5]
[10, 5]
Finally, with min equal to 5, we need to get the value at index 0 and assign to max.
max = [10, 5][0]
Now the swap is complete. min = 5
and max = 10
.
Kirby Abogaa
3,058 PointsHi Guys!
I'm just wondering why functions and loops are being discussed here when the topic is about Number Objects. New students, like me, would not be able to understand this since it is not discussed even in the previous videos.
Anyway, i find this challenge to be very difficult since we have very limited options. I have almost the same code as Ammon but it does not work properly most of the time. Especially if the bottom number is zero and top number is one.
I will stop for a while and try to solve this. I will share my answer if I find one. And I will not watch the next video. Not until I give up! LoL.
Kirby Abogaa
3,058 PointsHi Guys!
This is my answer. Hope it helps! Let me know if there is something wrong.
This is for the hard part where you get two values and create a random number between those two.
Collect your numbers. Make sure the second number is higher than the first so we can use it as a parameter for our random number. Parse the collected value to an integer and store in a var
var numLow = parseInt ( prompt ( "PLease enter the first number." ) );
var numHigh = parseInt ( prompt ( "PLease enter a second number higher than the first." ) );
Create a random number.
var result = Math.random() * ( numHigh - numLow ) + numLow;
This will generate a number between zero and the difference of the highest number to the lowest. It will ensure that the final result will not exceed the highest number.
We add back the lowest number to the random number generated to make sure that the final result is always above or equal to it.
You may use the other Math Object functions such as Math.ceil if you want the result to be a whole number.
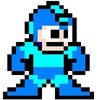
Robert Richey
Courses Plus Student 16,352 PointsHey Kirby,
Great job figuring out the challenge! This was a bit tricky for me when I first tried it, so I understand the initial difficulty.
Robert Richey
Courses Plus Student 16,352 PointsRobert Richey
Courses Plus Student 16,352 PointsHi Ammon,
I added markdown to help make the code more readable. If you're curious about how to add markdown like this on your own, checkout this thread on posting code to the forum . Also, there is a link at the bottom called Markdown Cheatsheet that gives a brief overview of how to add markdown to your posts.
Cheers!