Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial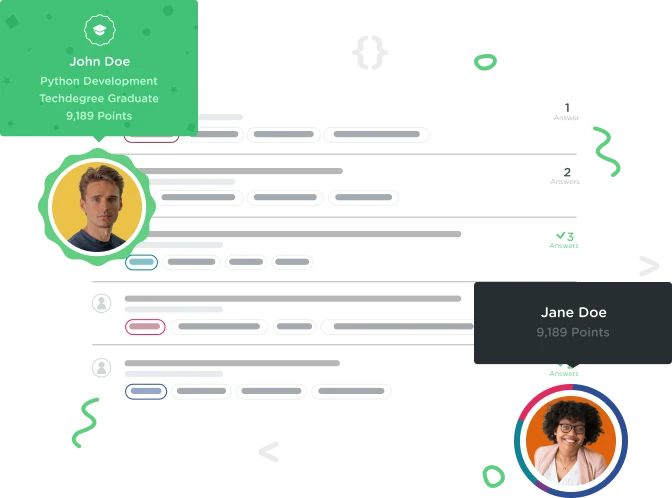

Marc Lim
7,705 PointsMy Solution for the "Build a Quiz Challenge" (and a few questions)
Well this is the solution I derived:
var quiz = [
["What is 3 + 5?", "8"],
["How many continents are there?", "7"],
["What comes after life?", "death"]
];
var question;
var score = 0;
var correctAnswers = "";
var wrongAnswers = "";
//Function for ease of printing to doc.
function print(message) {
document.write(message);
}
//Prompting the Quiz Questions and Storing Ans.
for (var i = 0; i < quiz.length; i += 1) {
question = prompt( quiz[i][0] );
question = question.toLowerCase();
if (question === quiz[i][1]) {
correctAnswers += "<li>You answered \"" + quiz[i][0] + "\" correctly!</li>"
score += 1;
} else {
wrongAnswers += "<li>You failed to get \"" + quiz[i][0] + "\" correct.</li>"
}
}
//"Your Score" Message
print("You got " + score + " question(s) right.");
//Correct Answers
print("<h2>You got these questions correct:</h2>");
var correctList = print("<ol>" + correctAnswers + "</ol>");
//Wrong Answers
print("<h2>You got these questions wrong:</h2>");
var wrongList = print("<ol>" + wrongAnswers + "</ol>");
The code does meet the objectives.
Firstly, a technical question -- why is it that I am assigning an empty value to some of the variables. I only did so, as testing revealed that the browser would render "undefined" onto the page without an empty value of "". I am not too clear as to when I have to assign an empty value when creating variables.
I have tried thinking through that, realized that couldn't I just created all variables right from the start with = " ". Even if I didn't, I am rule-bound to test my code, and any undefined errors would then get fixed.
I guess what I do want to know, is whether there is a more intuitive way to know when I have to assign = " " right from the start. Hopefully you all have some insight.
Next question, then would be more about the approach to take when coding JS.
After comparing the instructor's solution to my own, I just didn't know whether I can feel as though my solution is acceptable. I felt that I had a pretty clear sense during the coding as to why I was writing it the way I did. Even discounting the bias that I wrote it, I felt I had a easier time digesting my version over the instructor's.
So it just bothered me, that I was not using more "fancier" stuff I'm being taught to complete the challenge. And that it didn't just intuitively occur to me like, "Hey, I need a new function here". Or the .push() method etc.
To sum it up, I feel that maybe my code is too simplistic or non-optimal. Could someone maybe explain the intuitive reason the instructor had when creating the other function. Hopefully something in my mind can click after hearing new reasoning.
Thank you for your time. Cheers.
1 Answer
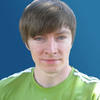
Alexander Efremov
15,409 PointsMy thoughts: We should assign a value to variable when we do something with it after. We're telling the type of our variable. I mean, try this one:
var emptyString;
var newString = emptyString + " concatenate something";
//and that one:
var emptyString = "";
var newString = emptyString + " concatenate something";
When we haven't assigne value yet it doesn't have a type and we can't use functions - for exemple:
var newString;
newString.length; //error
var newString = "";
newString.length; // 0
About your solution - I think it's completely ok. We just have a topic - arrays - so we're using arrays. But I think your solution is good in this situation - maybe even better - because you use less loops - so maybe your code runs faster. It's good that you are able to find alternative solutions.
Marc Lim
7,705 PointsMarc Lim
7,705 PointsThanks for the explanation mate! I think I now got a pretty good idea about the empty variables.
I supposed I coded according to the objectives at hand, and if there had been different objectives I would have used more functions/methods that would meet them too. But it's still nice to know that the isn't any glaring issues with my solution. Thanks again.