Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial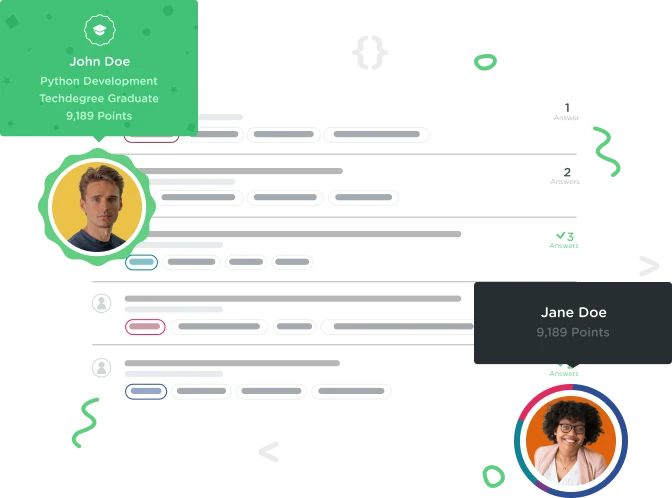
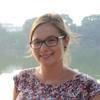
Birthe Vandermeeren
17,146 PointsMy solution for the checkForWin() method
I've attempted my own solution for the checkForWin() method. The difference with Ashley's solution: Ashley checks the entire board for a 4 in a row sequence in each of the 4 directions, while I only check for a 4 in a row sequence in the target column in the vertical direction and in the target row in the horizontal column. If there's a 4 in a row sequence in another column/row, that's been detected when that token was dropped, so no need to search the entire board for a 4 in a row sequence. Hope this makes sense.
Here's my code:
checkForWin(target){
let targetColumn = target.x;
let targetRow = target.y;
let owner = target.owner;
let win = false;
//vertical
if ( targetRow <= this.board.rows - 4 ) {
if ( this.board.spaces[targetColumn][targetRow].owner === owner &&
this.board.spaces[targetColumn][targetRow + 1].owner === owner &&
this.board.spaces[targetColumn][targetRow + 2].owner === owner &&
this.board.spaces[targetColumn][targetRow + 3].owner === owner) {
win = true;
}
}
//horizontal
for ( let x = 0; x <= this.board.columns - 4; x++ ) {
if ( this.board.spaces[x][targetRow].owner === owner &&
this.board.spaces[x + 1][targetRow].owner === owner &&
this.board.spaces[x + 2][targetRow].owner === owner &&
this.board.spaces[x + 3][targetRow].owner === owner) {
win = true;
}
}
//upwardly diagonal
for ( let x = 0; x <= this.board.columns - 4; x++ ) {
for ( let y = 3; y <= this.board.rows - 1; y ++ ) {
if ( this.board.spaces[x][y].owner === owner &&
this.board.spaces[x + 1][y - 1].owner === owner &&
this.board.spaces[x + 2][y - 2].owner === owner &&
this.board.spaces[x + 3][y - 3].owner === owner) {
win = true;
}
}
}
//downwardly diagonal
for ( let x = 3; x <= this.board.columns - 1; x++ ) {
for ( let y = 3; y <= this.board.rows - 1; y ++ ) {
if ( this.board.spaces[x][y].owner === owner &&
this.board.spaces[x - 1][y - 1].owner === owner &&
this.board.spaces[x - 2][y - 2].owner === owner &&
this.board.spaces[x - 3][y - 3].owner === owner) {
win = true;
}
}
}
console.log(win);
return win;
}
2 Answers
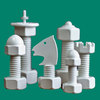
Steven Parker
231,275 PointsIt's a good idea, and would be an efficiency improvement over the original. Another student was working on a similar concept just last Saturday.
At first glance, it appears you have vertical checks covered, but it looks like horizontal checks only look for wins where the new token is dropped onto the left side. To make the check complete, it should also detect wins where the token is dropped on the right side or inside the row.
If you wanted even more challenge, you could also adapt the diagonal tests to only check the diagonal lines that include the new token.
And good job on finding creative ways to expand your learning!
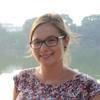
Birthe Vandermeeren
17,146 PointsYou're right, Steven! Thanks for your thoughts on this!
Birthe Vandermeeren
17,146 PointsBirthe Vandermeeren
17,146 PointsFor the vertical direction I'm just checking if there are 3 more tokens of the same owner right below the spot where the current token is dropped, because that's the only way a 4 in a row sequence can be made in this turn.
For the horizontal direction I'm looping through all spaces which have at least 3 spots behind them in the target row, starting with x=0. Which means if I'm making a 4 in a row sequence by dropping a token in the third column between 2 tokens in front and 1 token behind the token I just dropped, it's already detected in the first iteration, when checking with x=0. So I believe my code is correct.
Doing the diagonal checks in this way seemed to much of a hassle! 😅
Steven Parker
231,275 PointsSteven Parker
231,275 PointsMy "first glance" was a bit hasty, I see now that the horizontal check is thorough. I was thinking it started with the token position. It could still be optimized a bit: