Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial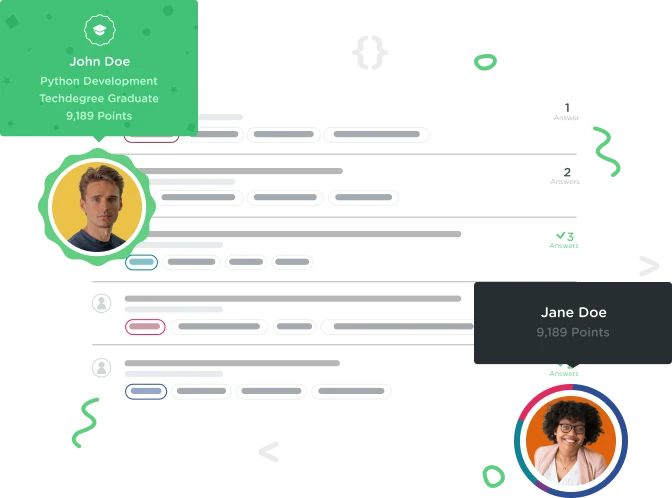
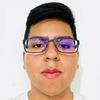
Diego A. J. V.
8,049 PointsMy solution for the extra challenge
function newStudent (name, track, achievements, points) {
var student = {};
student.name = name;
student.track = track;
student.achievements = achievements;
student.points = points;
return student;
}
function getReport (id) {
var student = students[id]
var studentReport = "";
studentReport += "<h2>Student: " + student.name + "</h2>";
studentReport += "<p> Track: " + student.track + "</p>";
studentReport += "<p> Achievements: " + student.achievements + "</p>";
studentReport += "<p> Points: " + student.points + "</p>";
return studentReport;
}
function searchStudent(name) {
var result = "";
var flag = 0;
for(var i = 0; i < students.length; i += 1) {
if( name === students[i].name ) {
flag = 1;
result += getReport(i);
}
}
if ( flag === 0 ) {
result += "<h2>The student does not exist.</h2>"
}
return result;
}
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
var students = [];
var studentSearch;
students.push(newStudent("Diego", "Front End Development", 15, 1450));
students.push(newStudent("Roberto", "Web Design", 17, 1234));
students.push(newStudent("Frank", "iOS", 12, 1378));
students.push(newStudent("Frank", "Android", 11, 1900));
students.push(newStudent("Jimena", "Back End Development", 7, 956));
while(true) {
studentSearch = prompt("What student looking for?");
if ( studentSearch.toLowerCase() === 'quit' || studentSearch === null ) {
break;
} else {
print(searchStudent(studentSearch));
}
}
What do you think about my solution?
3 Answers
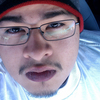
Chyno Deluxe
16,936 PointsGreat Job!

Markus Ylisiurunen
15,034 PointsIt looks good! There are couple of things I would have done a little differently and you can see those changes here if you'd like to check them out. But as I said, your solution looks good.
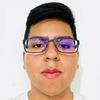
Diego A. J. V.
8,049 PointsThank you!, but I don't understand this part of the code, can you explain me or where I can find more information about it.
(function promptSearch() {
studentSearch = prompt("What student looking for?");
if (studentSearch && studentSearch.toLowerCase() != 'quit') {
print(searchStudent(studentSearch));
setTimeout(promptSearch, 0);
}
})();

Markus Ylisiurunen
15,034 PointsYeah sure I can.
// this is a self executing function
// I used it to start the loop of prompting the user for names
(function promptSearch() {
// code here
})();
// we first check that there indeed is some 'truthy' value (not null, undefined, false etc.)
// after that we make sure that the user didn't type 'quit'
if (studentSearch && studentSearch.toLowerCase() != 'quit') {
// code here
}
// after that if we indeed got some valid input we run this code inside the if statement
// setTimeout is here to call this same function again to ask for new name
// it works the same way as your while loop
print(searchStudent(studentSearch));
setTimeout(promptSearch, 0);
I used setTimeout() instead of while loop for one reason. When you're using while loop the changes in the page might not get rendered before blocking prompt() call and your UI might appear to be frozen. When using setTimeout() instead it doesn't block the javascript execution the same way as using your method would. This is a quite advanced topic if you want to go any deeper.
Here's a little explanation if you'd like to read more about it.
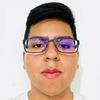
Diego A. J. V.
8,049 PointsThank you!
Diego A. J. V.
8,049 PointsDiego A. J. V.
8,049 PointsThank you :)!