Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial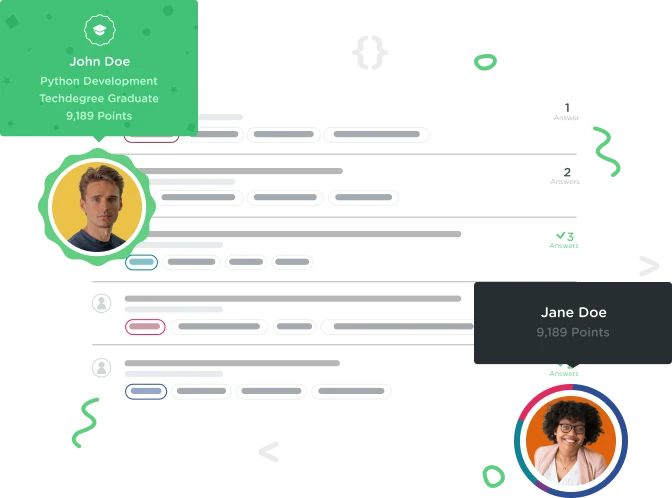

zy93
Python Web Development Techdegree Student 6,879 PointsMy solution for the extra credit assignment
Hi there everyone!
I just thought to share my solution for the extra-credit assignment that Kenneth gave us in the hopes that it would encourage others to try as well! It was quite a lot of fun, actually!
import re
with open('names.txt') as f:
data = f.read()
regex = re.compile(r'''
^(?P<last_name>[-\w ]+)?,\s # Last Name
(?P<first_name>[-\w ]+)\t # First Name
(?P<email>[-\w\d.+]+@[-\w\d.]+)\t # Email
(?P<phone>\(?\d{3}\)?-?\s?\d{3}-\d{4})?\t # Phone
(?P<job>[\w\s]+,\s[\w\s.]+)\t? # Job and company
(?P<twitter>@[\w\d]+)?$ # Twitter
''', re.M|re.X)
class Person:
def __init__(self, *args, **kwargs):
for k, v in kwargs.items():
setattr(self, k, v)
def __repr__(self):
return f"{self.first_name} {self.last_name} <{self.email}>"
class AddressBook:
def __init__(self):
self.people = []
def add(self, other):
self.people.append(other)
def __repr__(self):
return self.people
def __str__(self):
return str(self.people)
def find_where_equals(self, parameter_name, value):
"Return list of people"
return [person for person in self.people if hasattr(person, parameter_name) \
and str(value) == str(getattr(person, parameter_name))]
def find_where_contains(self, parameter_name, value):
"Return list of people"
return [person for person in self.people if hasattr(person, parameter_name) \
and value in str(getattr(person, parameter_name))]
address_book = AddressBook()
# Populating address book with data from file
for entry in regex.finditer(data):
person = Person(**entry.groupdict())
address_book.add(person)
# Some examples of usage of the address book
print(address_book.find_where_equals('first_name', 'Kenneth'))
print(address_book.find_where_contains('phone', '5'))
for person in address_book.find_where_contains('phone', '5'):
print(person.phone)