Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial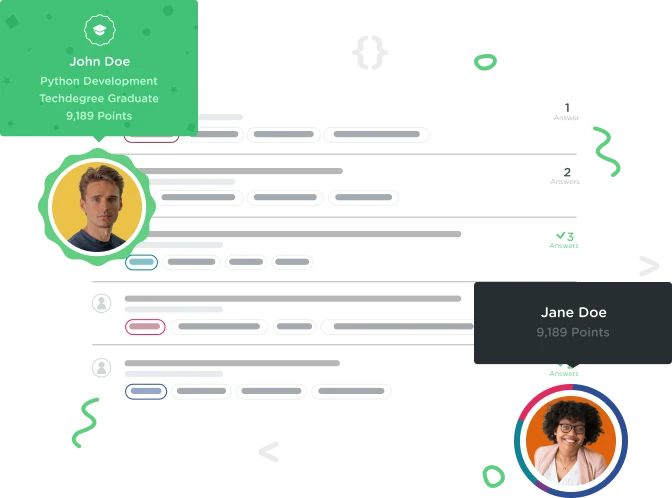
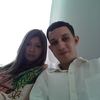
Raul Vega
Courses Plus Student 3,803 PointsMy solution for the Quiz Challenge Pt.1 (Feedback appreciated)
var correctAnswers = [];
var incorrectAnswers = [];
var questions = [
['Which is the biggest planet on our galaxy?', 'jupiter'],
['Which is the second planet from the sun?', 'venus'],
['To which planet we refer to as the "Red Planet"?', 'mars']
];
function print(message) {
document.write(message);
}
for ( var i = 0; i < questions.length; i+= 1 ){
var userInput = prompt(questions[i][0]);
userInput = userInput.toLowerCase();
if (userInput === questions[i][1]){
alert("Correct!");
correctAnswers.push(questions[i][0]);
}else{
alert("Incorrect!");
incorrectAnswers.push(questions[i][0]);
}
}
var html = "<h1>Results</h1>";
html+= "<i>You answered "+correctAnswers.length+" out of "+questions.length+" questions correctly!</i>";
html+= "<h2>Correct Answers</h2>";
if (correctAnswers.length > 0){
html+= "<ol><li>";
html+= correctAnswers.join("</li><li>");
html+= "</li></ol>";
}else{
html+= "<i>You did not answered correctly any of the questions.</i>";
}
if (incorrectAnswers.length > 0){
html+= "<h2>Incorrect Answers</h2>";
html+= "<ol><li>";
html+= incorrectAnswers.join("</li><li>");
html+= "</li></ol>";
}
print(html);
1 Answer
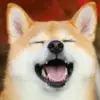
jobbol
Full Stack JavaScript Techdegree Student 16,610 PointsDoing a great job so far! Your solution is pretty creative. Code is clean. Result screen is nicely formatted. I would prefer a few more comments, but the code seems to speak for itself.
I never would have thought of using a arrays to track the questions. Most people used integers and incremented as the user missed or got one right. With arrays you can just use the length method. Plus you have the benefit of knowing which questions were right and wrong, not just the count itself.
Additionally you did some input sanitation by converting the case to lowercase. That way "Jupiter", "jupiter" and "JUPITER" are all valid.
Comments again could help in some parts. This for instance stumped me for a bit. It's great if you understand your own code, but explaining it to others can be just as important. Leave comments in places that aren't self-explanatory.
var userInput = prompt(questions[i][0]);
userInput = userInput.toLowerCase();
Future improvements
Later on you'll get into Modularizing, where things are broken down into easier to understand parts. You'll learn best practices on representing your data. Instead of your code reading like an old wives tale. Where it just goes on and on with no end in sight, no distinction of parts, and everything just blends together.
You want to abstract it down into its most basic parts. For your code this would be something like askQuestion, checkAnswer, and showResults. In javascript this would look something like.
for( var i = 0; i < questions.length; i+= 1 ){
answer = askQuestion();
checkAnswer(answer);
}
showResults();
This has the main advantage of showing the underlying structure more clearly. Plus it also gives more meaning to your code, like it was all commented.
Good luck out there!
Raul Vega
Courses Plus Student 3,803 PointsRaul Vega
Courses Plus Student 3,803 PointsHello Josh,
Thanks a lot for the recommendations, yes I need to practice more on commenting I was just scared to clutter the code since I found it self explanatory probably because I wrote it but I can understand how hard would it be if someone else read it, including myself after a long time without access to the code.
Looking forward to improve my Modularizing skills as well.
Thanks again for taking your time to check my code and giving a quality reply.