Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial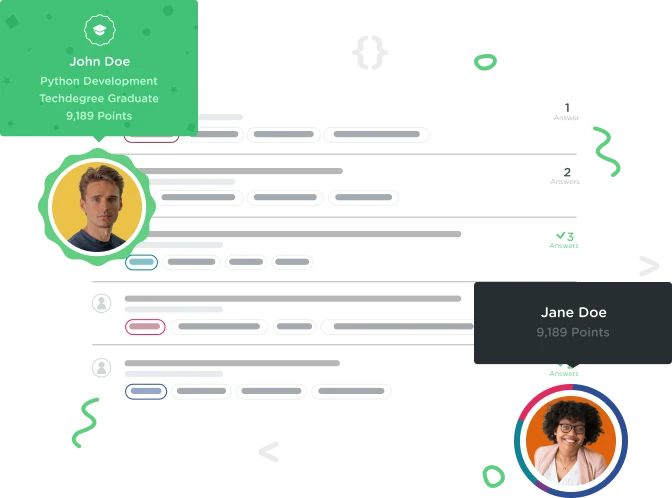

Jesse Benedict
Courses Plus Student 4,260 PointsMy solution for the Refactor Challenge
For future reference or usage, I've decided to leave the RGB variables alone and put them in an array instead and did it this way by implementing another loop
let html = '', red, green, blue, randomRGB;
const colors = [red, green, blue];
const RandColorPicker = (colArray) => {
for(let i = 0; i < colArray.length; i++) {
colArray[i] = Math.floor(Math.random() * 256);
}
};
for(let i = 1; i < 11; i++) {
RandColorPicker(colors);
randomRGB = `rgb( ${colors[0]}, ${colors[1]}, ${colors[2]} )`;
html += `<div style="background-color: ${randomRGB}">${i}</div>`;
}
document.querySelector('main').innerHTML = html;
It's not as compact but can be efficient for a further manipulation of RGB values if needed
1 Answer
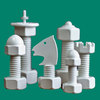
Steven Parker
231,268 PointsI'm not sure if your array would be considered an improvement over the video example, but it's clever!
It occurred to me that you don't really need to declare variables for the individual color values, you can use temporary literal values when creating the array:
let html = '', randomRGB;
const colors = [0, 0, 0];
And since you want to loop through the entire array, you could use for...in:
for (let i in colArray) {
colArray[i] = Math.floor(Math.random() * 256);
}
You could also use the array's forEach method instead of a loop:
colArray.forEach((_,i,a) => {
a[i] = Math.floor(Math.random() * 256);
});