Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial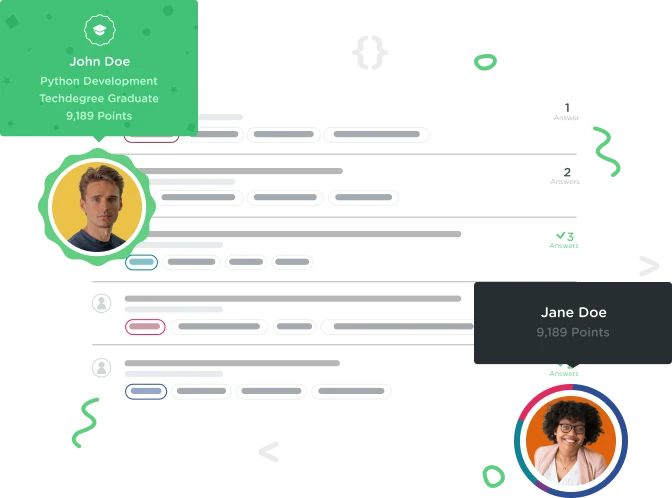
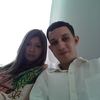
Raul Vega
Courses Plus Student 3,803 PointsMy solution for the refactor challenge (Feedback appreciated)
Here is my solution for the refactor challenge using while commented out and for.
var html = '';
var red;
var green;
var blue;
var rgbColor;
/* CODE USING WHILE
var colorCount = 0;
while ( colorCount!==10 ){
red = Math.floor(Math.random() * 256 );
green = Math.floor(Math.random() * 256 );
blue = Math.floor(Math.random() * 256 );
rgbColor = 'rgb(' + red + ',' + green + ',' + blue + ')';
html += '<div style="background-color:' + rgbColor + '"></div>';
colorCount+=1;
}
*/
//CODE USING FOR START
for (var colorCount = 0; colorCount!==10; colorCount+=1){
red = Math.floor(Math.random() * 256 );
green = Math.floor(Math.random() * 256 );
blue = Math.floor(Math.random() * 256 );
rgbColor = 'rgb(' + red + ',' + green + ',' + blue + ')';
html += '<div style="background-color:' + rgbColor + '"></div>';
}
//CODE USING FOR END
document.write(html);

fbriygig
15,370 PointsAlso worth mentioning that
var html, red, green, blue, rgbColor;
html = '';
is the same as
var html = '';
var red;
var green;
var blue;
var rgbColor;
but uses less code and saves you from typing 'var' repeatedly.

Jordan Gauthier
5,552 PointsYour code looks good. I recommend using the < operator in the condition rather than !== as it is safer, but in this example it works. From here, you could improve upon the code by placing all of the code in a function and then calling it at the bottom. You could also put Math.floor(...) in its own function and call it. If the program was extended, then the function could provide more flexibility. Good job!
Gunhoo Yoon
5,027 PointsGunhoo Yoon
5,027 PointsFactor out the '''Math.floor(Math.random() * 256 )''' into helper function.