Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial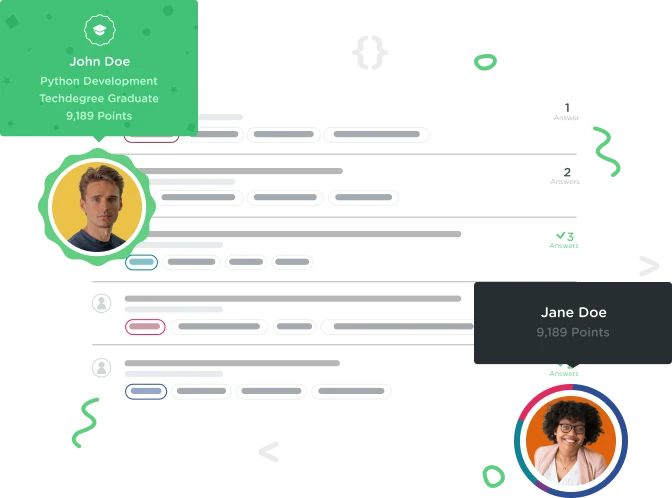
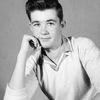
Allen Terzian
3,311 PointsMy solution for the 'Student Not Found' and 'Multiple Students' Search
Posting this to help people as it drove me almost insane to figure it out. Snapshot here: https://w.trhou.se/d5zpdmd2ho
or see code below:
var message = '';
var student;
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
function getStudentReport(student) {
var report = '';
for (var x = 0; x < student.length; x += 1) {
report += '<h2>Student: ' + student[x].name + '</h2>';
report += '<p>Track: ' + student[x].track + '</p>';
report += '<p>Points: ' + student[x].points + '</p>';
report += '<p>Achievements: ' + student[x].achievements + '</p>';
}
return report;
}
while (true) {
var group_students = [];
var search = prompt('Search student records: type a name [Jody] or type quit to end.');
if (search === null || search.toLowerCase === 'quit') {
break;
}
else {
for (var i = 0; i < students.length; i += 1) {
student = students[i];
if (search === student.name) {
group_students.push(student);
message = getStudentReport(group_students);
}
}
if (message === '') {
alert('Student not found.');
}
else {
print(message);
}
}
}
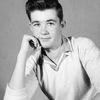
Allen Terzian
3,311 PointsMike Hatch - The 'students.length' is pulled from the separate 'students.js' file that holds the array of student information. You're probably running this code without that file. If you choose to run it in Console, make sure you paste the following code inside the code I posted above, or else it won't work:
var students = [
{
name: 'Dave',
track: 'Front End Development',
achievements: 158,
points: 14730
},
{
name: 'Jody',
track: 'iOS Development with Swift',
achievements: '175',
points: '16375'
},
{
name: 'Jordan',
track: 'PHP Development',
achievements: '55',
points: '2025'
},
{
name: 'Jody',
track: 'Learn WordPress',
achievements: '40',
points: '1950'
},
{
name: 'Trish',
track: 'Rails Development',
achievements: '5',
points: '350'
}
];
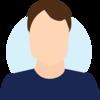
Mike Hatch
14,940 PointsAh, right... Silly me. That's why I didn't Answer. Thank you. That's a good reminder to be mindful of separate files when testing code.
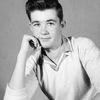
Allen Terzian
3,311 PointsNo worries and that's very true!
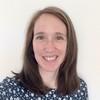
Ellinor Carlstedt
5,869 PointsThank you so much for posting this solution! This assignment drove me insane too, and I didn't have as much patience with it as you apparantly did.
However, when I tried your solution I found that the "Student not found" message only appears if you type in an unknown name during the very first search. If you once have searched for, and found, a student in the register, the variable "message" already has a string in it. Therefore the condition "if (message === ' ' )" will never be true again.
This can be easily solved by declaring the variable "message" within the for-loop, just like the variable "group_students. Then it will be reset for every new search.

Wynston Champion
16,728 PointsI also noticed that when typing 'quit' it did not result in the prompt closing. The response was 'Student not found'. I am not sure of a solution at the moment, but wanted to point that out.
Mike Hatch
14,940 PointsMike Hatch
14,940 PointsI'll comment without answering because I don't have a solution for you. That being said, when I run it in the Console I get an error message for the loop area. See where you have "students.length"? It's undefined. I changed it to "group_students" which is defined and that fixed the Error message issue. However, it doesn't fix the program to run how you want it. Hopefully someone else can help you. This was a difficult challenge for many.