Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial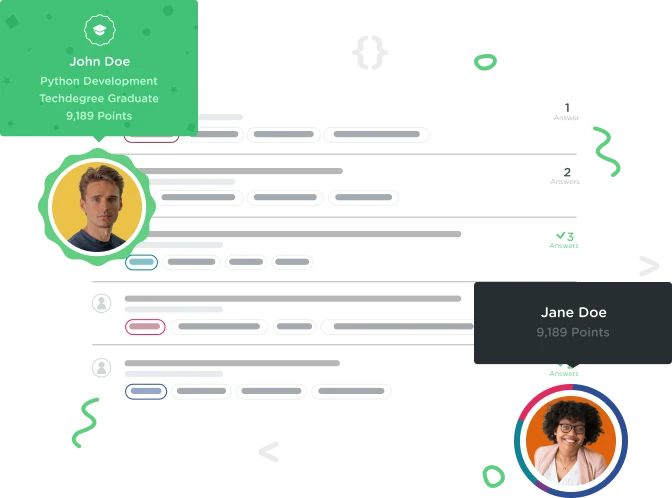
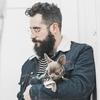
Alexander D
8,685 PointsMy solution for this challenge. Adding the total cost of ALL the products in the store
Hello folks,
I was confused about the guidelines for this challenge. I thought Dave wanted us to calculate the whole value of all items in the shop. The solution includes something that hasn't been discussed in this course yet, and I will link an article below:
https://medium.com/@chrisburgin95/rewriting-javascript-sum-an-array-dbf838996ed0
Here's the solution:
let products = [
{
name: "chair",
inventory: 5,
unit_price: 45.99
},
{
name: "table",
inventory: 10,
unit_price: 123.75
},
{
name: "sofa",
inventory: 2,
unit_price: 399.50
}
];
function totalValue () {
var storedValues = [];
var sum = 0;
for (let i=0; i < products.length; i++) {
storedValues.push(products[i].inventory * products[i].unit_price)
sum = sum + storedValues[i]
}
return sum
}
alert(totalValue (products))
1 Answer
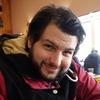
Eric M
11,546 PointsInteresting article! It's using concepts that are part of what's referred to as "functional programming".
There's no functional JavaScript course on Treehouse yet, and it is probably best to get the basics of the language down first, functional programming concepts can be confusing for many developers to learn. There is a good functional Python course available though, and there's plenty of functional programming in JavaScript articles on the web. Once you've found your feet as a developer I highly recommend checking it out - in some cases it can be a huge time saver!
In regards to the code you posted, be careful about your indentation. Clear indendation makes your code a lot easier for a human to parse (you are probably a human so this helps you too). The clearer the code is the less likely that something unexpected happens and the easier bugs are to fix.
Here's how I might lay out the code after your array, though there are many valid styles.
function totalValue ()
{
var storedValues = [];
var sum = 0;
for (let i=0; i < products.length; i++)
{
storedValues.push(products[i].inventory * products[i].unit_price)
sum = sum + storedValues[i]
}
return sum
}
alert(totalValue (products))
For me, this is much easier to read!
Alexander D
8,685 PointsAlexander D
8,685 PointsThanks for your feedback! Your code goes down like an iced glass of water on a summer day!