Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial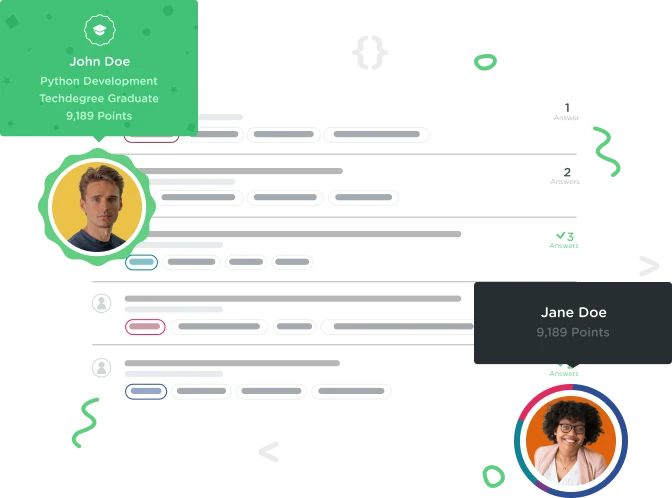
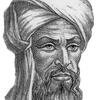
Armend Azemi
27,921 PointsMy solution for when the search results are ambiguous.
So, doing this video in 2022, some names of the astronauts currently in space, return more than one value from the Wikipedia page, thus leaving us with a default image and no real description.
Here is my solution to fixing that problem so that we can correctly display the information about the astronauts even when Wikipedia is returning several results.
function extractJSONDataFromAmbigiousResult(searchTerm){
return new Promise((resolve, reject) => {
let data = getJSON(wikiUrl+searchTerm)
let specificSearchTerm = ''
data.then(json => {
if (json.extract.includes('naut')){
// Regex for words ending with 'naut'
let regex = new RegExp(/\w*naut\b/gm)
// Getting 2 values per search, ['astronaut', 'astronaut']. We only want a single value.
let occupation = json.extract.match(regex)[0]
specificSearchTerm = searchTerm + ' ' +'('+occupation+')'
let specificSearchTermData = getJSON(wikiUrl+specificSearchTerm)
resolve(specificSearchTermData)
}
})
});
}
This is the helper function I used to 'extract' the astronaut data when we get a response with multiple options from Wikipedia.
// Generate the markup for each profile
function generateHTML(data) {
data.map(personalData => {
const section = document.createElement('section');
peopleList.appendChild(section);
// Check if request returns a 'standard' page from Wiki
if (personalData.type === 'standard') {
section.innerHTML = `
<img src=${personalData.thumbnail.source}>
<h2>${personalData.title}</h2>
<p>${personalData.description}</p>
<p>${personalData.extract}</p>
`;
} else {
let data = extractJSONDataFromAmbigiousResult(personalData.title)
data.then(json => {
section.innerHTML = `
<img src=${json.thumbnail.source}>
<h2>${json.title}</h2>
<p>Results unavailable for ${json.title}</p>
${json.extract_html}
`;
})
}
})
}
And this is the modification needed to the generateHTML()
function, as can be seen in the else
clause.
Hope this helps, and perhaps you guys can share your solutions or improvements/suggestions to my solution.
Cheers, and happy coding!
1 Answer
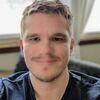
Jack McCauley
Front End Web Development Techdegree Graduate 17,093 PointsVery cool, I was just running into all those undefined errors and your solution fixed all of that!