Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial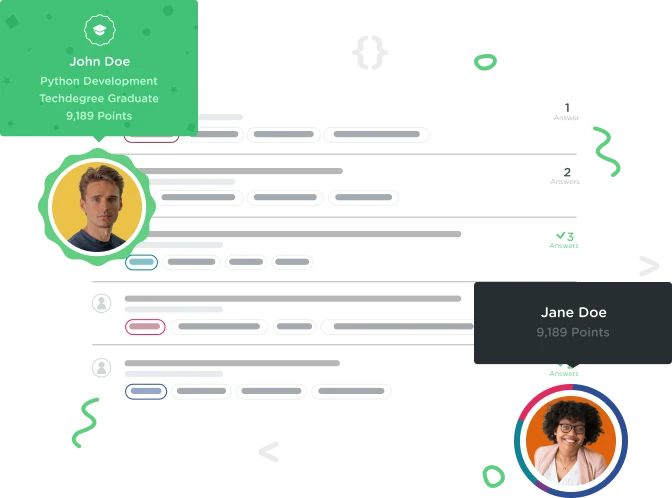

Kevin Bullis
13,770 PointsMy solution. I think I'm missing the point of prototypes.
My solution, below, works. But I think I may be missing the point of prototypes. Will my code only run on the instance of quiz I made, quiz1? I tried changing all the "quiz1." in my functions to "this." to make it more flexible, but then the code didn't work anymore.
Also, my solution to the Quiz project works in Brackets and in Work Spaces previews, but not in Code Pen. Any idea why?
I'll try pasting the javascript below.
I had to add a .hidden style (display set to none) to the CSS, and jquery links to the html
<script src="https://code.jquery.com/jquery-1.11.3.js"></script>
<script src="quiz.js"></script>
Here's the javascript, all in one file, quiz.js
/////////////Constructors/////////////
//template for questions/answers
function Question(question, choice0, choice1, correctChoice) {
this.question = question;
this.choice0 = choice0;
this.choice1 = choice1;
this.correctChoice = correctChoice;
}
//storage for Quiz questions and counters
function Quiz() {
this.questions = [];
this.arrayIndex = 0;
this.correctAnswersCounter = 0;
}
////////////Instances////////////////
// create instances of Question
var question1 = new Question("How many sides does a triangle have?", "3", "24", "choice0");
var question2 = new Question("Is Catwoman hanging from the ceiling?", "no", "yes", "choice1");
var question3 = new Question("Is this quiz awesome?", "Yes", "No", "choice0");
var question4 = new Question("Does the carpet cleaner work?", "Yes", "No", "choice1");
var question5 = new Question("Does this quiz really work?", "No", "Yes", "choice1");
var question6 = new Question("How many questions can I add?", "As many as I want.", "No more than 5.", "choice0");
// create an instance of Quiz
var quiz1 = new Quiz();
////////////Prototype Methods/////////////
//Add question/answers to the quiz.
Quiz.prototype.addQuestion = function (questionAnswer) {
this.questions.push(questionAnswer);
};
quiz1.addQuestion(question1);
quiz1.addQuestion(question2);
quiz1.addQuestion(question3);
quiz1.addQuestion(question4);
quiz1.addQuestion(question5);
quiz1.addQuestion(question6);
//changing the page
//functions for showing and hiding elements
Quiz.prototype.sendHTML = function () {
$("#question").html(quiz1.questions[quiz1.arrayIndex].question);
$("#choice0").html(quiz1.questions[quiz1.arrayIndex].choice0);
$("#choice1").html(quiz1.questions[quiz1.arrayIndex].choice1);
var questionNumber = quiz1.arrayIndex + 1;
$("footer").html("Question " + questionNumber + " of " + quiz1.questions.length + ".");
};
Quiz.prototype.hideQuizElements = function () {
$("#question").addClass("hidden");
$("#choice0").addClass("hidden");
$("#choice1").addClass("hidden");
$("#guess0").addClass("hidden");
$("#guess1").addClass("hidden");
$("footer").addClass("hidden");
};
Quiz.prototype.showQuizElements = function () {
$("#start").addClass("hidden");
$("#question").removeClass("hidden");
$("#choice0").removeClass("hidden");
$("#choice1").removeClass("hidden");
$("#guess0").removeClass("hidden");
$("#guess1").removeClass("hidden");
$("footer").removeClass("hidden");
};
Quiz.prototype.showNextButton = function () {
$("#nextButton").removeClass("hidden");
};
Quiz.prototype.hideNextButton = function () {
$("#nextButton").addClass("hidden");
};
Quiz.prototype.showFinalButton = function () {
$("#finalButton").removeClass("hidden");
};
Quiz.prototype.hideFinalButton = function () {
$("#finalButton").addClass("hidden");
};
Quiz.prototype.activateAnswerButtons = function () {
$("#guess0").on("click", quiz1.checkAnswer);
$("#guess1").on("click", quiz1.checkAnswer);
};
Quiz.prototype.clearButtonClasses = function () {
$("#guess0").removeClass("btn--success btn--error").html("Select Answer");
$("#guess1").removeClass("btn--success btn--error").html("Select Answer");
};
//Adding and removing from DOM
Quiz.prototype.firstLoadQuestionsPage = function () {
quiz1.addNextButton();
quiz1.hideNextButton();
quiz1.sendHTML();
quiz1.showQuizElements();
quiz1.activateAnswerButtons();
};
//quiz mechanics
// checking the answers
Quiz.prototype.checkAnswer = function () { //what happens when you click on one of the quiz answers
$("#guess0").off();
$("#guess1").off();
var correct = quiz1.questions[quiz1.arrayIndex].correctChoice;
if ($(this).prev().attr("id") === correct) {
$(this).addClass("btn--success").html("Right!");
quiz1.correctAnswersCounter += 1;
} else {
$(this).addClass("btn--error").html("Bummer! That's the wrong answer.");
}
quiz1.showNextButton();
};
Quiz.prototype.loadNextSetOfQuestions = function () {
console.log("click is working");
quiz1.arrayIndex += 1;
if (quiz1.arrayIndex < quiz1.questions.length) {
quiz1.clearButtonClasses();
quiz1.activateAnswerButtons();
quiz1.sendHTML();
} else {
quiz1.hideQuizElements();
quiz1.addFinalPageButton();
};
quiz1.hideNextButton();
};
// method to hide the quiz and load a summary page
Quiz.prototype.loadFinalPage = function () {
quiz1.addTryAgainButton();
quiz1.hideFinalButton();
$("#score").html("You got " + quiz1.correctAnswersCounter + " right out of " + quiz1.questions.length + " questions.");
console.log("We've reached the end");
};
// method to start the quiz over again
Quiz.prototype.takeAgain = function () {
window.location.reload();
};
//adding buttons
Quiz.prototype.addStartButton = function () {
var p = '<p></p>',
start = '<button id="start" class="btn--default">Start quiz</button>';
$("#quiz").append(p, start);
$("#start").on("click", quiz1.firstLoadQuestionsPage);
};
quiz1.hideQuizElements();
quiz1.addStartButton(); //to have a start button on the page to start with.
Quiz.prototype.addNextButton = function () {
var p = '<p></p>',
nextButton = '<button id="nextButton" class="btn--default">Next</button>';
$("#quiz").append(p, nextButton);
$("#nextButton").on("click", quiz1.loadNextSetOfQuestions);
};
Quiz.prototype.addFinalPageButton = function () {
var p = '<p></p>',
finalButton = '<button id="finalButton" class="btn--default">See how you did</button>';
$("#quiz").append(p, finalButton);
$("#finalButton").on("click", quiz1.loadFinalPage);
};
Quiz.prototype.addTryAgainButton = function () {
var p = '<p></p>',
restart = '<button id="restart" class="btn--default">Try again?</button>';
$("#quiz").append(p, restart);
$("#restart").on("click", quiz1.takeAgain);
};