Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial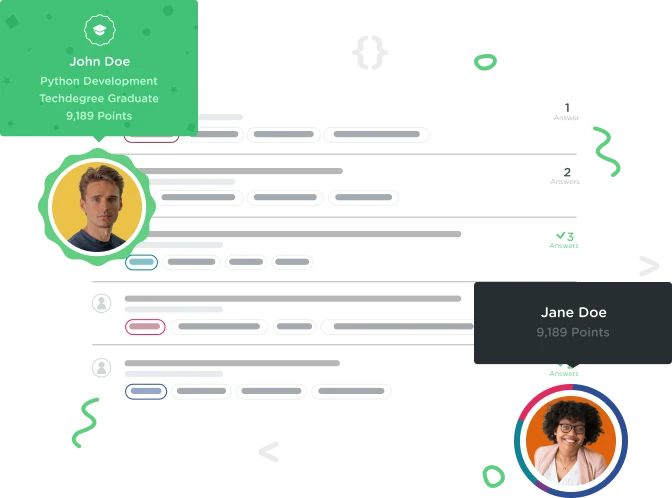

Mark Tripney
8,666 PointsMy solution (including the two extra problems).
let search;
let html;
function print(studentData) {
document.querySelector('#output').innerHTML = studentData;
}
function buildOutput(student) {
html += `<h2>Student: ${student.name}</h2>`;
html += `<p>Track: ${student.track}</p>`;
html += `<p>Achievements: ${student.achievements}</p>`;
html += `<p>Points: ${student.points}</p>`;
print(html);
}
function findStudent() {
// Flag required to prevent 'not on file' message in every loop.
let notOnFile = true;
for (let i = 0; i < students.length; i += 1) {
if (students[i].name.toLowerCase() === search.toLowerCase()) {
buildOutput(students[i]);
notOnFile = false;
} else if (notOnFile) {
const output = `<h2>${search} is not on file.</h2>`;
print(output);
}
}
}
while (true) {
search = prompt("Type a name (or 'quit' to close):");
// null is returned if user presses the prompt's 'Cancel' button.
if (search.toLowerCase() === 'quit' || search === null) {
break;
} else {
// Clear screen before showing next result.
html = '';
findStudent();
}
}
(My students array (const students = [ ... ]
), was in an external file, hence the references to students
in this script.)
I was working in Firefox Developer Edition, where the output is shown dynamically, behind the prompt box. Therefore, I made the design decision to have the screen clear each time a new search is made. This keeps the output cleaner and more readable. If your browser doesn't work this way, simply remove the html = ''
line in the while
loop.
function findStudent()
uses a flag, notOnFile
, to control whether or not the 'not on file' message is shown. This is necessary because, otherwise, this message would be shown for every iteration of the loop which didn't match the search term. In other words, you'd always get the 'not on file' message!
3 Answers
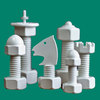
Steven Parker
231,275 PointsAnother approach for the "not on file" message is to wait until the search loop has completely finished before showing it.
That will also be helpful if you want to enhance the code to show the results of multiple searches instead of just the last one done.

Trevor Maltbie
Full Stack JavaScript Techdegree Graduate 17,021 PointsI don't understand how this shows us all students of the same name. It works, yes, but how? Is it because you made the findStudent() function and added that to the while loop?

Mark Tripney
8,666 PointsHey Trevor. No, the critical work is being done in findStudent()
. The while
loop is relatively banal β it's handling user input and, when appropriate, asking findStudent()
to get to work.
The key is the relationship between findStudent()
and buildOutput()
, and the for
loop in findStudent()
. The for
loop is iterating over all students and, every time a match is found between the user input and a student's name
value, buildOutput()
assembles the HTML and asks print()
to insert it into the DOM. This process continues so long as there are student records to check βΒ when there are none left unchecked, the for
loop is exhausted and the process ends.
Hope this helps. Any questions, gimme a shout.