Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial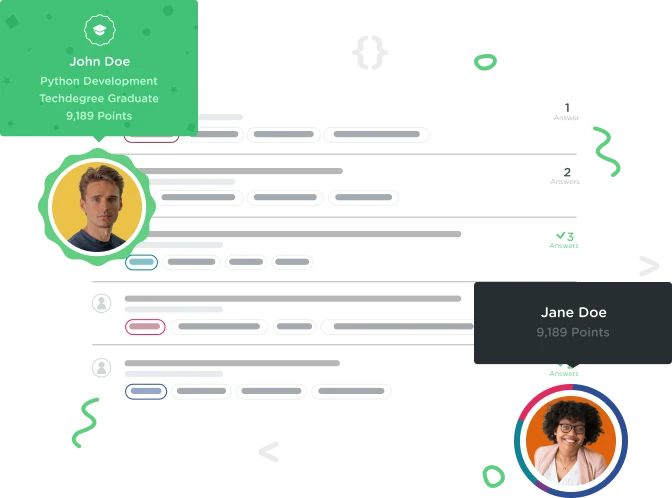
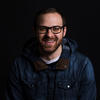
Josh Cummings
16,310 PointsMy Solution - Interactive Web Pages with JavaScript - Perfect
To fix the ability to add blank list item, I used an if statement in the "Add a new task" section of our code.
// Add a new task
var addTask = function() {
if (taskInput.value) {
console.log("Add task...");
// Create a new list item with the text from #new-task
var listItem = createNewTaskElement(taskInput.value);
// Append list item to incompleteTaskHolder
incompleteTasksHolder.appendChild(listItem);
bindTaskEvents(listItem, taskCompleted);
taskInput.value = "";
}
}
The taskInput.value simply checks to see if the filed has a value. If it does, it lets you click add. Otherwise it does not.
To make the edit button say save while in edit mode, I added the following if statement to the "Edit an existing task" section of our code.
// Edit an existing task
var editTask = function() {
console.log("Edit task...");
var listItem = this.parentNode;
var editButton = listItem.querySelector("button.edit");
var editInput = listItem.querySelector("input[type=text");
var label = listItem.querySelector("label");
var containsClass = listItem.classList.contains("editMode");
// If the class of the parent is editMode
if (containsClass) {
// Switch from edit mode
// label text become the input's value
editButton.innerText = "Edit";
label.innerText = editInput.value;
} else {
// switch to editMode
// input value becomes the label's text
editButton.innerText = "Save";
editInput.value = label.innerText;
}
// Toggle editMode the parent
listItem.classList.toggle("editMode");
}
I selected the editButton using querySelector and said that if the button had the class editMode, it should use the innerText method to change it to Save. Otherwise, it should say Edit.
Hope this helps. Appreciate any and all feedback!
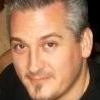
Rick Gates
11,061 PointsWhat about preventing the addition of a task if it only contains spaces as input? Otherwise, a technically blank task can still be added.
Rick
5 Answers
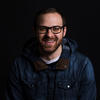
Josh Cummings
16,310 PointsHi Meowls,
The match method in JavaScript checks for a match in a string and returns null if no value is found. The length method returns the number of characters in a string, therefore, the following will throw an error:
var fooNull = null;
console.log(fooNull.length);
I would do something that returns a boolean value for your if statement like so:
if (newTask.value) {
// Do something
} else {
// Do something else
}
See the following fiddle: https://jsfiddle.net/82wgrwsu/6/
Hope this helps!

Greg Kaleka
39,021 PointsNice! I did almost the same thing, except that I checked to see if the input for adding a task was blank, and returned if so.
var addTask = function()
{
if (!taskInput.value) { return; }
var listItem = createNewTaskElement(taskInput.value);
incompleteTaskHolder.appendChild(listItem);
bindTaskEvents(listItem, taskComplete);
taskInput.value = "";
}
My edit/save solution was identical to yours.

James Delaplain
5,872 PointsThis doesnt seem to work for me, I tried what you guyz said and it got even more Frozen. https://w.trhou.se/lxepmkacnf

Greg Kaleka
39,021 PointsHey James,
At the bottom of your app.js file, you reference completeTasksHolder
, but that variable doesn't exist. You created completedTasksHolder
. That's your issue. Add a 'd' to both instances there and you'll be set.
For future reference, if you're experiencing unexpected behavior, check the javascript console. That's how I diagnosed your issue. Chrome told me exactly what line of what file had an error.

meowls
6,840 PointsMy solution for the edit/save is the same, but I tried something different to disallow empty strings being added. The first part works. However, when trying to alert a user that they did not enter anything, I get an error that the length of null cannot be read. Does anyone have any suggestions for how to make the alert work? I originally had it if (value < 1) { alert } else { add the item }, until I realized even just typing a space counts as character.
if (newTask.value.match(/\S/g).length) {
incomplete.appendChild(listItem);
bindTaskEvents(listItem, completedTasks);
newTask.value = '';
} else {
alert("Oops! Looks like you forgot to write something.");
}
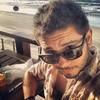
james rochabrun
Courses Plus Student 22,726 PointsGreat job to everyone, can someone tell me why something like this doesnt work?
// a variable that returns true or false if the taskInput.value.lenght is <= than 0
function inputLength() { return taskInput.value.length <= 0; }
//a function that changes the property calling the inputLenghth function.
function disableButton() { addButton.disabled = inputLength(); }
// call the disableButton function
disableButton();
thank you very much
Keith Wallace
3,413 PointsKeith Wallace
3,413 PointsNice!
I did the same code as you when changing the button text from "Edit"/"Save", but I like your solution when checking if the new task string is blank - less code! For my solution I have a IF/ELSE statement and alerting the end user if the task they are submitting is blank: