Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial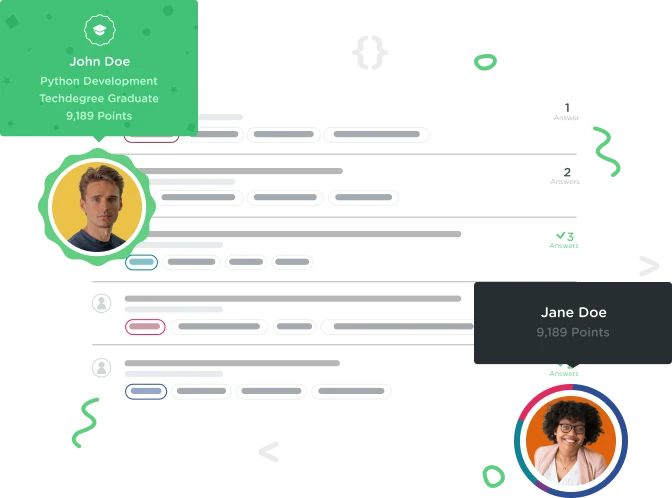

Mayur Pande
Courses Plus Student 11,711 PointsMy solution is slightly different, having difficulty with extra challenges
my solution is slightly different however I cannot solve the extra challenges;
var message = '';
var student;
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
function getStudentDetails(name){
for (var i = 0; i < students.length; i += 1) {
student = students[i];
if(name === student.name.toLowerCase()){
message += '<h2>Student: ' + student.name + '</h2>';
message += '<p>Track: ' + student.track + '</p>';
message += '<p>Points: ' + student.points + '</p>';
message += '<p>Achievements: ' + student.achievements + '</p>';
}else if(name !== student.name.toLowerCase()){
//I cannot get this to work
alert("There is no person with that name in the system");
promptUser();
}
}
return message;
}
function promptUser(){
var result = "";
while(true){
result = prompt("Search student records: type a name [mayur] (or type 'quit' to end)");
if(result.toLowerCase() === "quit"){
break;
}else{
getStudentDetails(result.toLowerCase());
}
}
}
promptUser();
print(getStudentDetails());
I have tried to alert the user when they type in the wrong name however it seems to go to the prompt again but when I enter a correct name, it goes back to saying the name is not in the system
4 Answers

David Service
12,928 PointsHi guys,
I've been able to get both extra features to work. I started off with what David Bath said above about using a new conditional statement tree (by using the second 'if' statement) to check the length of the "message" variable after the "for" loop was finished running through the array of student records (thanks David, I had no idea we could have multiple conditional statement trees in a single loop, and it helped a lot).
Took some time and experimentation to get the multi-record printing to work, but as it turns out all you need to do is place the "message" variable into the function for building student records (instead of using a variable scope variable inside the function). If a student record is successfully found, the string gets added to the "message" variable; and this simply means that if more than one student record is found it all gets added to the "message" variable as a longer string.
Last thing, with the direct inclusion of the "message" variable in the record-building function, we have a string that is constantly being built upon each time we find a student record. This means that if we enter a student name that isn't there, the condition for alerting the user to a non-existent student (for example)
if(message.length == 0){.....}
will not work. To fix this, all I did was declare the "message" variable as an empty string at the beginning of the "while" loop so that the contents of the variable were cleared each time the loop ran.
Hope that helps, and you can find my code below.
David
// PREP
var message = '';
var student;
var search;
function getStudentReport(studentVariable){
message += '<h2>Student: ' + student.name + '</h2>';
message += "<ol>";
message += '<li>Track: ' + student.track + '</li>';
message += '<li>Points: ' + student.points + '</li>';
message += '<li>Achievements: ' + student.achievements + "</li>";
message += "</ol>";
return message;
}
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
// THE PROGRAM
while (true){
message = "";
search = prompt("Please enter a student name to see their records.");
if (search == null || search.toLowerCase() == "quit"){
break;
}
else {for(var i = 0; i < students.length; i += 1){
student = students[i];
if(search.toLowerCase() == student.name.toLowerCase()){
getStudentReport(student);
print(message);
}
}
}
if(message.length == 0){
alert("We don't have that student in our system.");
}
}
// THE EXECUTION
/* There is no need to execute our program by calling upon it, since the infinite loop above automatically starts running when read by the browser.*/

David Bath
25,940 PointsNice work! I have a couple of comments...
Your function getStudentReport has an argument studentVariable
that is not currently used (the lines inside the function are referring to the global student
variable), so you can either remove it or change all references inside the function to studentVariable
.
Another small tweak would be to change
getStudentReport(student);
print(message);
to
print(getStudentReport(student));
Since getStudentReport
returns the message string, that is what will be printed.

David Bath
25,940 PointsI think there are some problems with your logic. Let's talk about the getStudentDetails function. This function is looping through all the student records searching for a match. But if the first record doesn't match, then it goes into the else
condition, which alerts that no student was found that matches. That's not what you want to do.
You should loop through all records, and then if no student was found that matches, then give an alert. So I'd suggest removing the else
. Then after the loop is finished you can check for the length of the message string. If the length is 0 then no student was found and you can give an alert and then re-call the promptUser function. If the length is >0 then a student was found and you can call the print function there, with the message as the argument. Then you don't need to call the print function at the end of the file as you are doing.

Mayur Pande
Courses Plus Student 11,711 PointsHi, thanks for the answer, unfortunately I still seem to be getting problems. I now understand what you were saying about how I should remove the else
otherwise I would only checking for the current student.
However creating another conditional after the for loop, when calling the promptUser()
function it calls the prompt but then if I type in "quit" it keeps running until it eventually displays "undefined"

David Bath
25,940 PointsCan you post your updated code?

David Bath
25,940 PointsThis is kind of what I was thinking:
var message, search, student;
function getStudentReport(student) {
var studentString = '';
studentString += '<h2>Student: ' + student.name + '</h2>';
studentString += "<ol>";
studentString += '<li>Track: ' + student.track + '</li>';
studentString += '<li>Points: ' + student.points + '</li>';
studentString += '<li>Achievements: ' + student.achievements + "</li>";
studentString += "</ol>";
return studentString;
}
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
// THE PROGRAM
while (true) {
message = "";
search = prompt("Please enter a student name to see their records.");
if (search == null || search.toLowerCase() == "quit"){
break;
}
else {
for(var i = 0; i < students.length; i += 1){
student = students[i];
if (search.toLowerCase() == student.name.toLowerCase()){
message += getStudentReport(student);
}
}
if (message.length == 0) {
alert("We don't have that student in our system.");
} else {
print(message);
}
}
}
I haven't tested this, but I think it will work...

David Service
12,928 PointsHi David,
Thanks for the comments and the tweeks. I forgot to take the "studentVariable" parameter out when I wrote the code for the final feature, but thankfully it didn't make any difference (but still good to catch that leftover and take it out for efficiency's sake).
Mayur Pande
Courses Plus Student 11,711 PointsMayur Pande
Courses Plus Student 11,711 PointsI think this might have something do with it;
As when I type enter an incorrect name it shows the alert, then when I type in a correct name it shows the alert again which it shouldn't but when I type quit it shows the alert again which it shouldn't but then it exits and shows the correct details for the name.