Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial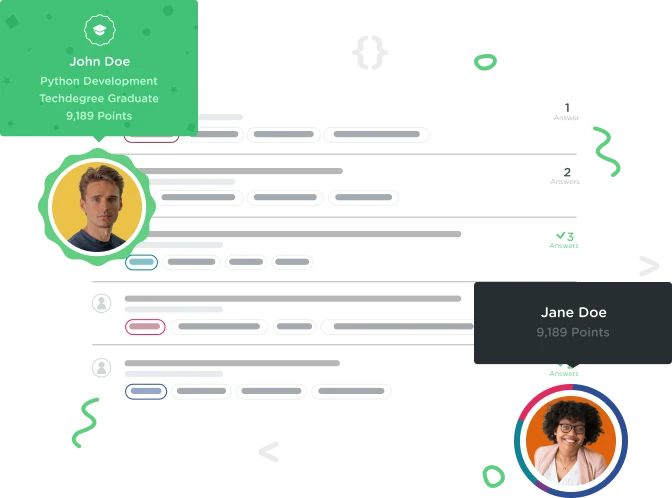
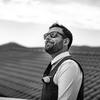
Gary Stevenson
2,310 PointsMy solution. Possible to make it more compact?
Hi,
This is my solution to the problem. The code runs as intended but I'm wondering if there's a way to make the code more compact.
For this section;
red = colorGen();
green = colorGen();
blue = colorGen();
rgbColor = 'rgb(' + red + ',' + green + ',' + blue + ')';
Would it be possible to add the "colorGen()" function into the rgbColor variable therefore eliminating the need to repeat the function as a new variable in 'red', 'green', 'blue', i.e.;
rgbColor = 'rgb( colourGen() + colourGen() + colourGen() )';
// or
rgbColor ='rgb( colourGen() * 3)';
I tried both of these methods but it resulted in no colours being shown. Is this just a problem with my syntax or is it not possible to insert a function into the rgb() function?
Thanks in advance, Gary.
5 Answers
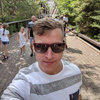
Christian Fynbo
Full Stack JavaScript Techdegree Student 2,910 PointsA modern solution that avoids the global scope would be something along this arrow function:
const coloredCircles = amount => {
const rgb = () => Math.floor(Math.random() * 256);
for (let i = 0; i < amount; i++) {
let html = '';
let rgbColor = `rgb( ${rgb()}, ${rgb()}, ${rgb()})`;
html += `<div style="background-color:${rgbColor}"></div>`;
document.write(html);
}
}
The nested arrow function let rgb = () => Math.floor(Math.random() * 256);
deals with eliminating the red, green and blue variables, as you're suggesting, with the template literal assigned to the rgbColor
variable `rgb( ${rgb()}, ${rgb()}, ${rgb()})`;
.
When called, coloredCircles(10);
would generate 10 randomly colored circles, coloredCircles(100);
would generate 100 and so on.
If you want to specifically generate 10 colored circles within the parameters given, this is probably the most compact solution (while keeping to formatting norms):
const rgb = () => Math.floor(Math.random() * 256);
for (let i = 0; i < 10; i++) {
let html = '';
let rgbColor = `rgb( ${rgb()}, ${rgb()}, ${rgb()})`;
html += `<div style="background-color:${rgbColor}"></div>`;
document.write(html);
}
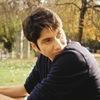
Ignacio Rocha
7,462 PointsHi Gary , Well I just test both solutions and both seems to work fine if you call the same function 3 times inside the rgb variable it shows the colors and that way you could eliminate the variables red, green and blue. Just to note that in the second solution you forget to concatenate the parenthesis.
rgbColor = 'rgb(' + colorTheme() + ',' + colorTheme() + ',' + colorTheme() + ')';
// or in template literal way
rgbColor = `rgb( ${colorTheme()}, ${colorTheme()}, ${colorTheme()} )`;
And to your question about the colors not showing maybe it is because your function is wrong and you didn't post it so I'd assume that that's the problem. Either way I would share you my solution and then comment me back if it works.
function colorTheme() {
var rgb = Math.floor(Math.random()* 256);
return rgb;
}
// Arrow function form
const colorTheme = () => {
const rgb = Math.floor(Math.random()* 256);
return rgb;
};

Mohsin Shafique
5,791 PointsYou dont have to create a const rgb inside the arrow function as you are already returning value to colorTheme const.
Also you dont have put curly braces and use return key in arrow function as it will do return value anyways.
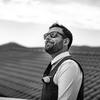
Gary Stevenson
2,310 PointsThanks!
Yeah I believe it was a syntax error in my code which was stopping it from working.
Cheers, Gary

Garrett Hensley
3,351 PointsHere was my solution, trying to make it as compact as possible: https://w.trhou.se/6otkubmfjw
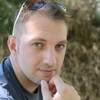
Doron Geyer
Full Stack JavaScript Techdegree Student 13,897 Pointsheres the solution I had for refactoring, let me know if you have any questions
var html = '';
function colorRand(){
return Math.floor(Math.random()*256);
}
function colorDot(iterations){
for(let i=0 ;i<iterations ;i++){
html+= `<div style="background-color: rgb(${colorRand()},${colorRand()},${colorRand()})"></div>`;
}
}
colorDot(10);
document.write(html);
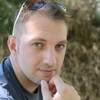
Doron Geyer
Full Stack JavaScript Techdegree Student 13,897 Pointsthis could be made more concise, but sometimes its better to have something easy and clear to read and understand, both for yourself and future readers.