Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial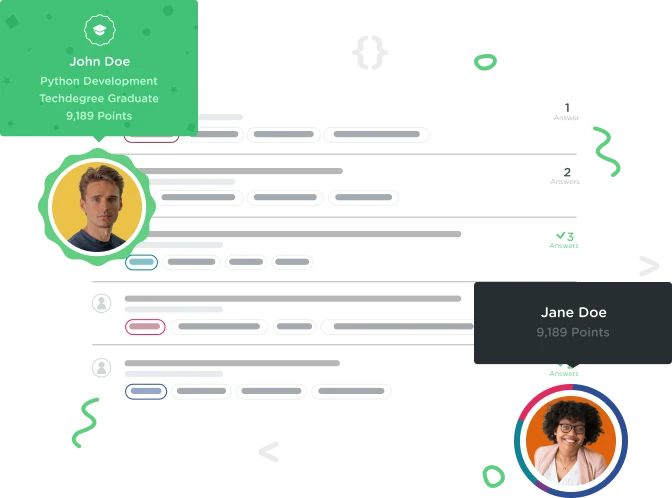

Nelson Lourenco
1,766 PointsMy solution re-writes correct/incorrect answers, help!
Here is my program:
var quiz = [
['What color are Gala apples?', 'Red'],
['What brand of phone do Koreans make?', 'Samsung'],
['What type of bird is a Macaw?', 'Parrot']
];
var numCorrect = 0;
for ( var i = 0; i < quiz.length; i += 1 ) {
var question = prompt(quiz[i][0]); /* response becomes new question value */
var answer = quiz[i][1];
if ( question === answer ) {
numCorrect += 1;
var correctQuestion = quiz[i][0];
document.getElementById('correct-list').innerHTML = ('<li>' + correctQuestion + '</li>');
}
else {
var incorrectQuestion = quiz[i][0];
document.getElementById('incorrect-list').innerHTML = ('<li>' + incorrectQuestion + '</li>');
}
}
document.write('You have answered ' + numCorrect + ' questions correct.');
I don't get why each correct question or answer is being overwritten as it cycles through? Thanks!
1 Answer

elk6
22,916 PointsHi Nelson,
Try this:
var quiz = [
['What color are Gala apples?', 'Red'],
['What brand of phone do Koreans make?', 'Samsung'],
['What type of bird is a Macaw?', 'Parrot']
];
var numCorrect = 0;
var correctQuestion = [];
var incorrectQuestion = [];
var correctMessage = "Correct Questions:";
var incorrectMessage = "Incorrect Questions: ";
for ( var i = 0; i < quiz.length; i += 1 ) {
var question = prompt(quiz[i][0]); /* response becomes new question value */
var answer = quiz[i][1];
if ( question === answer.toLowerCase() ) {
numCorrect += 1;
var correctQuestion = quiz[i][0];
(correctMessage += '<li>' + correctQuestion + '</li>');
}
else {
var incorrectQuestion = quiz[i][0];
(incorrectMessage += '<li>' + incorrectQuestion + '</li>');
}
}
document.getElementById('correct-list').innerHTML = correctMessage;
document.getElementById('incorrect-list').innerHTML = incorrectMessage;
document.write('You have answered ' + numCorrect + ' questions correct.');
Changes:
I first made empty arrays for the correct and incorrect questions to go in. You first need to create an empty array in order to fill it.
After that, i made 2 message-Vars to build up. If a question in correct, the loop puts the question in an <li> tag after the correct message and the same goes for the incorrect message. This way the you get all the correct and incorrect answers displayed instead of overwriting your innerHTML rule over and over. You might want to build the message up a bit further with the <ul> and the </ul> tags. You can build the vars i created up further with += like i did above.
Then i changed the prompt ( the answers the user gives ) to lowercase. And in the first if loop i changed the answer to lowercase as well. This way the answer will be counted as correct when someone types in the correct answer but misses a cap. Like "red" in the prompt box and the answer is "Red". You want to count that as right but in your solution it was counted as a wrong answer. .
Lastly, i displayed the correct and the incorrect message to the proper div with innerHTML.
You might want to change your HTML a tiny bit. Put 2 divs in there if you hadn't done that already. Like this:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>JavaScript Quiz</title>
<link rel="stylesheet" href="css/styles.css">
</head>
<body>
<h1>JavaScript Quiz</h1>
<div id="correct-list">
</div>
<div id="incorrect-list">
</div>
<div id="output">
</div>
<script src="js/quiz.js"></script>
</body>
</html>
Nelson Lourenco
1,766 PointsNelson Lourenco
1,766 PointsThanks for the reply Elian! The key for me was declaring the two variable sets in the beginning as arrays:
And then simply continue adding to them by:
(correctList += '<li>' + correctQuestion + '</li>');
P.S. The '.toLowerCase()' should be on the prompt answer (user response) and not the var answer :)
question = prompt(quiz[i][0]).toLowerCase(); /* response becomes new question value */
elk6
22,916 Pointselk6
22,916 PointsGlad it worked, Nelson. :)
One sidenote to your comment; since you wrote your answer with a capital you should have the toLowerCase() method on both the prompt and the answer. This way the given answer and your answer will always be lowercase. :)