Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial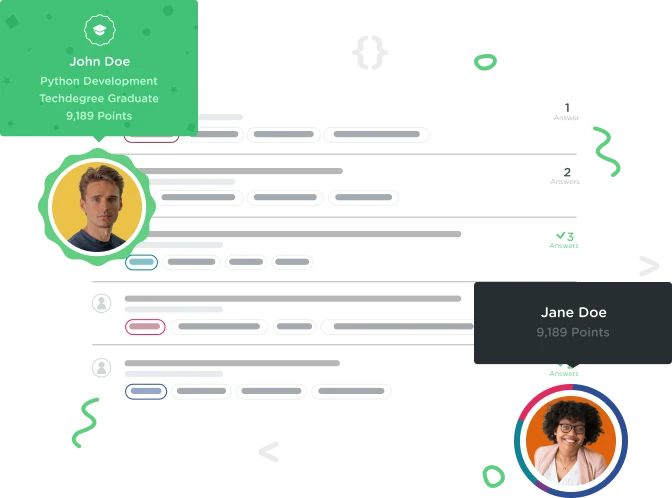
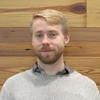
Cody Selman
7,643 PointsMy Solution to Build a Quiz Challenge Part 1
Here is my solution to this challenge: https://w.trhou.se/zkpp6wbqjx
Here's the JavaScript:
var numberCorrect = 0;
var qAndA = [
['What is 2 * 3?', '6'],
['What is 49 / 7?', '7'],
['What is 7 + 5?', '12']
];
function print(message) {
document.write(message);
}
for ( var i = 0; i < qAndA.length; i++){
var userAnswer = prompt( qAndA[i][0] );
if ( userAnswer === qAndA[i][1] ){
numberCorrect++;
}
}
print('You answered ' + numberCorrect + ' out of ' + qAndA.length + ' questions correctly.');
Let me know if you had any comments or improvements. Thank you.
5 Answers

kevin hudson
Courses Plus Student 11,987 Pointsanother version with more conditions
// instantiate global variables (counters)
var qCounter = 0;
var numRight = 0;
var numWrong = 0;
//create arrays for data
var rightAnswer = [];
var wrongAnswer = [];
var questionPool = [
['158=___ + 106. What number will come in the blank to make the number sentence true?', '52'],
['Which moon is larger than Mercury?', 'io'],
['What is the name of the little dragon in the animated movie Mulan?', 'mushu'],
['What is the name of the prison in the film The Rock?', 'alcatraz'],
['Give another name for the study of fossils?', 'paleontology'],
['Which insects cannot fly, but can jump higher than 30 cm?', 'flea'],
['Which plant does the Canadian flag contain?', 'maple'],
['Which is the largest desert on earth?', 'sahara'],
['What is the largest state of the United States?', 'alaska'],
['What is the capital of the American state Hawaii?', 'honolulu'],
];
// functions to be used
// updated print function to html doc
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
//calculate percentage
function calcPercent() {
var total = numRight + numWrong;
return numRight / total * 100;
}
//gather questions and put in html list format
function listQuestions(arr) {
var html = '<ol>';
for (var i = 0; i < arr.length; i++) {
html += '<li>' + arr[i] + '</li>';
}
html += '</ol>';
return html;
}
//main program. Iterate through array. Bring answer to lower case. Count right/wrong.Push to appropriate arrays
for (var i = 0; i < questionPool.length; i++) {
var question = questionPool[i][0];
var answer = questionPool[i][1];
var askQuestion = prompt(question.toLowerCase());
if (answer === askQuestion) {
numRight++;
rightAnswer.push(question);
} else {
numWrong++;
wrongAnswer.push(question);
}
}
// testing values. Note - only for dev testing
console.log('Testing Number right - '+numRight);
console.log('Testing Number wrong - '+numWrong);
//print html will depend on conditions
html = '<p>Here is your results:</p>';
if (numRight > 1 && numRight <10){
html += 'You got ' + numRight + ' correct questions and ' + numWrong + ' wrong questions. '+ calcPercent() + '%';
html += '<h2> Here is a list of questions you got right';
html += listQuestions(rightAnswer);
html += '<h2> Here is a list of questions you got wrong';
html += listQuestions(wrongAnswer);
} else if (numRight == 1){
html += 'You only got ' + numRight + ' correct question and ' + numWrong + ' wrong. ' + calcPercent() + '%';
html += '<h2> Here is the question you got right';
html += listQuestions(rightAnswer);
html += '<h2> Here is a list of questions you got wrong';
html += listQuestions(wrongAnswer);
} else if(numRight == 0) {
html += 'You did not get any correct questions. Please try again next time. '+ calcPercent() + '%';
html += '<h2> Here is a list of all the questions you got wrong';
html += listQuestions(wrongAnswer);
} else {
html += 'You got all ' + numRight + ' questions correct. Good Job! '+ calcPercent() + '%';
html += '<h2> Here is a list of all the questions you got right';
html += listQuestions(rightAnswer);
}
//doc write to div built html variable
print(html);
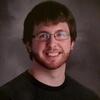
Kevin Michie
6,554 PointsHere is my solution that handles string vs number:
var questions= [
['What is your name?', 'kevin'],
['Who teaches this class?', 'dave mcfarland'],
['What is the meaning of life?', 42]
]
function print(message) {
document.write(message);
}
var correctAnswerCounter = 0;
var correctQuestions = [];
var incorrectQuestions = [];
function evaluator(questionArray) {
var html= '<ol>';
for(var i = 0; i < questionArray.length; i += 1) {
html += '<li>' + questionArray[i] + '</li>';
}
html += '</ol>';
print(html);
}
function quiz(questions) {
for(var i = 0; i < questions.length; i += 1){
answer = prompt(questions[i][0]);
if (typeof answer === "string" && isNaN(parseInt(answer))){
answer = answer.toLowerCase();
} else {
answer = parseInt(answer);
}
if(answer === questions[i][1]){
correctAnswerCounter +=1;
correctQuestions.push(questions[i][0]);
} else{
incorrectQuestions.push(questions[i][0]);
}
}
print('<p>You got ' + correctAnswerCounter + ' question(s) right.</p>');
print('<h3>You got these questions correct:</h3>');
evaluator(correctQuestions);
print('<h3>You got these questions wrong:</h3>');
evaluator(incorrectQuestions);
}
quiz(questions);

kevin hudson
Courses Plus Student 11,987 Pointsmy solution
function print(message) {
document.write(message);
}
//initial dimentional array questions with answers
var quizQuestions = [
['How many states are in the U.S.?', '50'],
['How many legs does an insect have?', '6'],
['How many continents are there?', '7']
];
// need to make array collectors for right/wrong answers
var rightQ = [];
var wrongQ = [];
//counter for right/wrong answers
var rightA = 0;
var wrongA = 0;
// iterate through dimentional array and push to collector array as well as add to counters
for (var i=0;i < quizQuestions.length; i++) {
var answer = prompt(quizQuestions[i][0]);
if (answer === quizQuestions[i][1] ) {
rightA++;
rightQ.push(quizQuestions[i][0]);
}else {
wrongA++;
wrongQ.push(quizQuestions[i][0]);
}
}
//function to collect array values and build html list to print
function collectQ(arrayQ){
html = '<ol>';
for (var i=0; i < arrayQ.length; i++){
html+= '<li>' + arrayQ[i] + '</li>';
}
html += '</ol>';
print(html);
}
//print titles with final values using array data and function output
print('You got ' + rightA + ' question(s) right.');
print('<h2 style="font-weight: bold"> You got these question(s) right.</h2>');
collectQ(rightQ);
print('<h2 style="font-weight: bold"> You Got these question(s) wrong.</h2>');
collectQ(wrongQ);

kevin hudson
Courses Plus Student 11,987 PointsSorry for the weird code post - I followed the markdown for posting code and I got this

kevin hudson
Courses Plus Student 11,987 Pointsanother version with added conditions
// instantiate global variables (counters)
var qCounter = 0;
var numRight = 0;
var numWrong = 0;
//create arrays for data
var rightAnswer = [];
var wrongAnswer = [];
var questionPool = [
['158=___ + 106. What number will come in the blank to make the number sentence true?', '52'],
['Which moon is larger than Mercury?', 'io'],
['What is the name of the little dragon in the animated movie Mulan?', 'mushu'],
['What is the name of the prison in the film The Rock?', 'alcatraz'],
['Give another name for the study of fossils?', 'paleontology'],
['Which insects cannot fly, but can jump higher than 30 cm?', 'flea'],
['Which plant does the Canadian flag contain?', 'maple'],
['Which is the largest desert on earth?', 'sahara'],
['What is the largest state of the United States?', 'alaska'],
['What is the capital of the American state Hawaii?', 'honolulu'],
];
// functions to be used
// updated print function to html doc
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
//calculate percentage
function calcPercent() {
var total = numRight + numWrong;
return numRight / total * 100;
}
//gather questions and put in html list format
function listQuestions(arr) {
var html = '<ol>';
for (var i = 0; i < arr.length; i++) {
html += '<li>' + arr[i] + '</li>';
}
html += '</ol>';
return html;
}
//main program. Iterate through array. Bring answer to lower case. Count right/wrong.Push to appropriate arrays
for (var i = 0; i < questionPool.length; i++) {
var question = questionPool[i][0];
var answer = questionPool[i][1];
var askQuestion = prompt(question.toLowerCase());
if (answer === askQuestion) {
numRight++;
rightAnswer.push(question);
} else {
numWrong++;
wrongAnswer.push(question);
}
}
// testing values. Note - only for dev testing
console.log('Testing Number right - '+numRight);
console.log('Testing Number wrong - '+numWrong);
//print html will depend on conditions
html = '<p>Here is your results:</p>';
if (numRight > 1 && numRight <10){
html += 'You got ' + numRight + ' correct questions and ' + numWrong + ' wrong questions. '+ calcPercent() + '%';
html += '<h2> Here is a list of questions you got right';
html += listQuestions(rightAnswer);
html += '<h2> Here is a list of questions you got wrong';
html += listQuestions(wrongAnswer);
} else if (numRight == 1){
html += 'You only got ' + numRight + ' correct question and ' + numWrong + ' wrong. ' + calcPercent() + '%';
html += '<h2> Here is the question you got right';
html += listQuestions(rightAnswer);
html += '<h2> Here is a list of questions you got wrong';
html += listQuestions(wrongAnswer);
} else if(numRight == 0) {
html += 'You did not get any correct questions. Please try again next time. '+ calcPercent() + '%';
html += '<h2> Here is a list of all the questions you got wrong';
html += listQuestions(wrongAnswer);
} else {
html += 'You got all ' + numRight + ' questions correct. Good Job! '+ calcPercent() + '%';
html += '<h2> Here is a list of all the questions you got right';
html += listQuestions(rightAnswer);
}
//doc write to div built html variable
print(html);
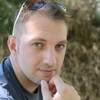
Doron Geyer
Full Stack JavaScript Techdegree Student 13,897 PointsGot the code to work fine but HTML formatting I have no idea what im doing, hoping at some point this fullstackJS course is going to cover html and css.
function print(message) {
document.write(message);
}
var html = "<ol>";
var incorrectQ = [];
var correctQ = [];
var corAns = 0;
var questions=[
["which programming lanugage are we using?", "JavaScript"],
["which programming language is named after a snake?", "Python"],
["which programming language is named after a gemstone", "Ruby"]
]
for(i=0;i<questions.length;i+=1){
var ques = prompt(questions[i][0]);
if(ques.toLowerCase() === (questions[i][1]).toLowerCase()){
correctQ.push(questions[i][0]);
corAns++
}else if(ques.toLowerCase() !== (questions[i][1]).toLowerCase()){
incorrectQ.push(questions[i][0]);
}
}
print(`you got ${corAns} questions correct and ${questions.length - corAns} incorrect.<br>`);
print("<h1>you got the following questins correct</h1>"+ "<li>"+ correctQ.join(", ")+"</li>" + "</ol>");
print("<h1>you got the following questins incorrect</h1>" + incorrectQ.join(", ") + "</ol>");
Joshua Petry
6,313 PointsJoshua Petry
6,313 PointsHere's my solution!