Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial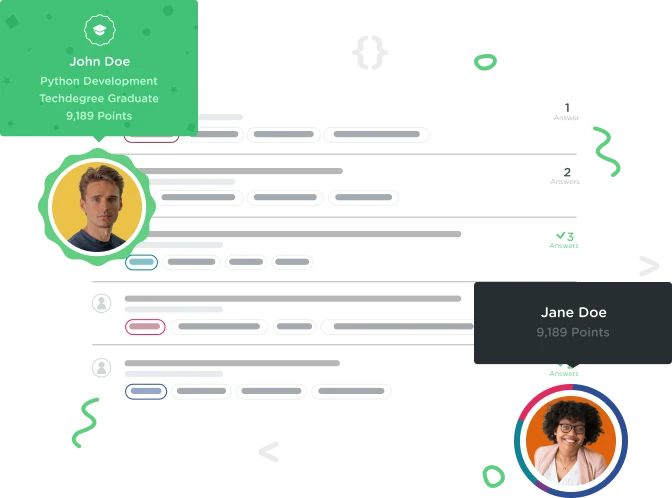

Fidel Severino
3,514 PointsMy Solution to Build a Quiz Challenge w/ comments
This newer challenge was even better than the last one! Dave's teaching methods are amazing. I'm grasping concepts much better than from other resources I've tried in the past. Can't wait to continue learning JS from him!
Below is my solution to the challenge. Hope it all makes sense. The comments are for my self-learning purposes. Although they should also help anyone understand my thought process (hopefully) :P
I also played around with adding alternate answers that people could provide for 2 of the questions.
// two-dimensional array holding questions + answer pairs inside of arrays
var questions = [
['Which language adds interactivity to a website?', 'javascript', 'js'],
['What allows for reusable blocks of code in JavaScript?', 'functions'],
['What is the JavaScript framework that is used server-side?', 'node.js', 'node']
];
/*
variables to hold the correct/incorrect questions
will be used to print question
*/
var correctQuestions = [],
incorrectQuestions = [],
// variables to keep track of the number of right vs wrong
correctAnswers = 0,
incorrectAnswers = 0;
// function to write to the page
function print( message ) {
document.write( message );
}
// function to print array of right/wrong questions
function printQuestions ( array ) {
for ( var i = 0; i < array.length; i++ ) {
print('<p>' + array[i] + '</p>');
}
}
// loop through each question in the array
for ( var i = 0; i < questions.length; i++ ) {
var currentQuestion = prompt( questions[i][0] );
/*
set returned string to lowercase for easier comparison.
If right, increase correctAnswers and push question to
correctQuestions array. Else, increase incorrectAnswers
and push to incorrectQuestions array
*/
currentQuestion = currentQuestion.toLowerCase();
if ( currentQuestion === questions[i][1] || questions[i][2] ){
correctAnswers++;
correctQuestions.push( questions[i][0] );
} else {
incorrectAnswers++;
incorrectQuestions.push( questions[i][0] );
}
}
print('<h3 id="correct">Correct Questions: ' + correctAnswers + '</h3>');
sortQuestions(correctQuestions);
print('<h3 id="incorrect">Incorrect Questions: ' + incorrectAnswers + '</h3>');
sortQuestions(incorrectQuestions);
Stanley Wilkins
685 PointsStanley Wilkins
685 PointsHey I have a question. How did you define variables without using var?
Another question: in the last 3 lines there is "sortQuestions" but how does that work? We have not defined such function and there is only built-in arr.sort() method.