Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial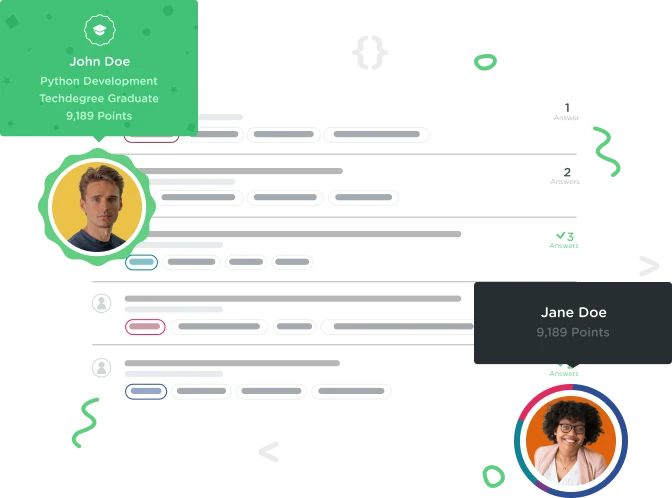

dmiller99
2,835 PointsMy solution to challenge
Also wanted to say used Nate Jonah code for making button disabled instead of hidden.
/* Select the elements we'll need, namely the paragraph, text input, and button, and assign them to const variables. */
const toggleList = document.getElementById("toggleList");
const listDiv = document.querySelector(".list");
const Descriptioninput = document.querySelector("input.description");
const DescriptionP = document.querySelector("p.description");
const DescriptionButton = document.querySelector(".list button");
const listUl = listDiv.querySelector("ul"); //find the ul within the div
const addItemInput = document.querySelector("input.addItemInput");
const addItemButton = document.querySelector("button.addItemButton");
const list = listUl.children;
const addVisibility = (list) => {
let firstListItem = list.firstElementChild;
let lastListItem = list.lastElementChild;
let allUp = list.querySelectorAll( ".up" );
let allDown = list.querySelectorAll( ".down" );
for( let i = 0; i < allUp.length; i++ ){
allUp[i].style.visibility = "visible";
}
for( let i = 0; i < allDown.length; i++ ){
allDown[i].style.visibility = "visible";
}
let topUp = firstListItem.querySelector(".up");
topUp.className = "blocked";
// topUp.style.visibility = "hidden";
let botDown = lastListItem.querySelector(".down");
botDown.className = "blocked";
};
const attachListItemButtons = ( li ) => {
let up = document.createElement("button");
up.className = "up";
up.textContent = "Up";
li.appendChild(up);
let down = document.createElement("button");
down.className = "down";
down.textContent = "Down";
li.appendChild(down);
let remove = document.createElement("button");
remove.className = "remove";
remove.textContent = "Remove";
li.appendChild(remove);
};
const setButtons = (list, listUl) => {
for (let i = 0; i < list.length; i++) {
attachListItemButtons(list[i]);
}
addVisibility(listUl);
};
//these two handlers are attached to the containing div but need to access the element triggering the event to make the changes to that element
listUl.addEventListener("click", (event) => {
if (event.target.tagName === "BUTTON") {
if (event.target.className === "remove") {
//get target target list
let li = event.target.parentNode; //the button is the event so its parent is li
//get target list parent node
let ul = li.parentNode; // the li parent is ul
//remove child
ul.removeChild(li);
}//end remove
if (event.target.className === "up") {
//get target target list
let li = event.target.parentNode; //the button is the event so its parent is li
//need prev sibling as a reference
let prevLi = li.previousElementSibling;
//get target list parent node
let ul = li.parentNode; // the li parent is ul
if (prevLi) {
//insert
ul.insertBefore(li, prevLi);
}
}//end up button check
if (event.target.className === "down") {
//get target target list
let li = event.target.parentNode; //the button is the event so its parent is li
//need prev sibling as a reference
let prevLi = li.previousElementSibling;
let nextLi = li.nextElementSibling;
//get target list parent node
let ul = li.parentNode; // the li parent is ul
if (nextLi) {
//insert
ul.insertBefore(nextLi, li);
}
}//end down button check
}//end check for button
let li = event.target.parentNode;
let sendUl = li.parentNode;
addVisibility(sendUl);
});
toggleList.addEventListener("click", () => {
//If button was clicked then we hide it. If clicked again unhide
if (listDiv.style.display === "none") {
toggleList.textContent = "Hide List";
listDiv.style.display = "block";
} else {
listDiv.style.display = "none";
toggleList.textContent = "Show List";
}
});
//add event listener to listen to when button is clicked
DescriptionButton.addEventListener("click", () => {
DescriptionP.innerHTML = Descriptioninput.value + ":";
Descriptioninput.value = "";
});
//we need to create 3 buttons and append to list item
addItemButton.addEventListener("click", () => {
//return collection which is sim to array
let ul = document.getElementsByTagName("ul")[0];
let li = document.createElement("li");
li.textContent = addItemInput.value;
attachListItemButtons(li);
ul.appendChild(li);
addItemInput.value = "";
//add to dom
let sendUl = li.parentNode;
addVisibility(sendUl);
});
setButtons(list, listUl);