Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial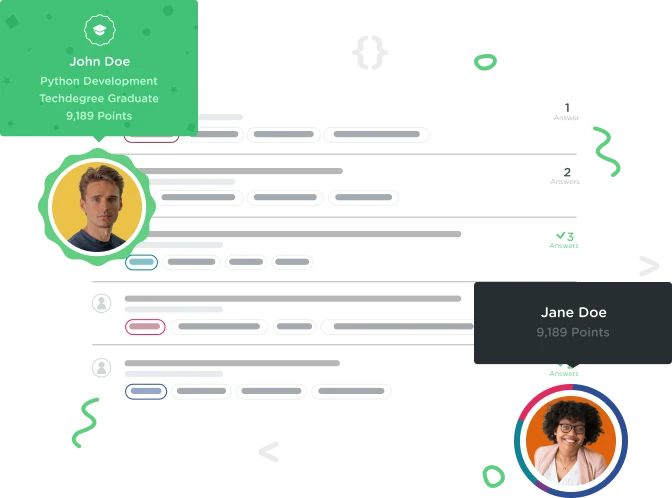
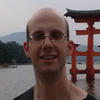
Andrés Angelini
Courses Plus Student 22,744 PointsMy solution to removing vowels: "Vowel Eater"
Not a question. I just thought I would share my solution. Hope it helps and any input would be much appreciated.
"""
VOWEL EATER: A very simply mini console game where the user is
asked to input a list of words and in return receives a new list with
the same words but without the vowels.
"""
import sys
# Return a list of words without vowels.
def eat_vowels(words):
leftovers = []
if words != ['']:
for word in words:
leftover = ""
for letter in word:
if letter not in "aeiou":
leftover += letter
# Uppercase the first letter of each word.
leftover = (leftover[0].upper() + leftover[1:])
leftovers.append(leftover)
return leftovers
# Main function.
def play():
while True:
try:
words = input("Hi! I'm hungry and want to eat some delicious wovels. "
"Enter some words separated by a comma to feed me.\n > ").split(', ')
except ValueError:
print("Hey! Those are not even words! Yack!")
else:
eat_vowels(words)
print("Yummy! This is what you got left with:")
print(", ".join(eat_vowels(words)))
# Prompt the user wether to play again or not.
play_again = ""
while play_again != "y" or play_again != "n":
play_again = input("Play again? Y/n ").lower()
if play_again == "n":
sys.exit()
else:
break
# Run the game.
play()
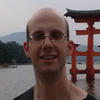
Andrés Angelini
Courses Plus Student 22,744 PointsThanks Emile for taking the time to check the code. You're right!
playing = True
It's just some left over code from my first attempt and that I forgot to remove later. I just edited the code and deleted it. As for the comma not being needed, that's because when you don't use a comma, the program just takes it a phrase, instead of a single word. That is, if you write "treehouse is awesome, shinny, elephant", you will get "Trhs s wsm, Shnny, Lphnt". In principle, this is not ideal because is not what the user expects, as you pointed out, but because one of the points of this is exercise is to use split(), I simply didn't bother. I guess that a better solution would be to use regex in conjunction with replace().
By the way, now that I tried it locally, I realize that it doesn't treat the input() as a string, unless I explicitly enter the words using commas. Could it be an issue of different python versions (between workspaces and the one installed on my system: 2.7.12)? I tried to wrap what comes back from input() with str() but to no avail.
Regards
Jason Bourne
2,301 PointsJason Bourne
2,301 PointsGreat code! One small detail,
playing = True
is redundant.Also, you don't need to ask the user to use a comma to separate words. It works just as well with or without them!
:)