Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial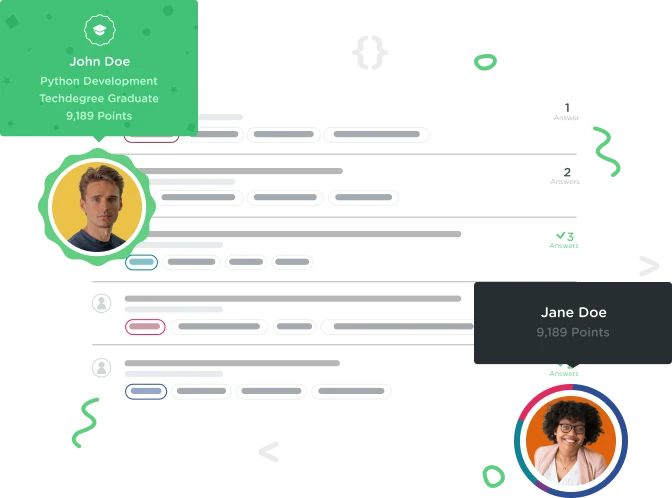
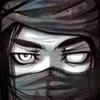
David Mendiola
Full Stack JavaScript Techdegree Student 16,579 PointsMy solution to student record search. How is it?
Instead of hard coding the property names for output decided to use the for in to get the property names and value pairs. Any critique greatly appreciated probably can optimize this more.
var message = '';
var student;
var response = '';
var result = [];
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
do {
response = prompt("Search a name of the student of type 'quit' to exit.");
//Searches students
for (var i = 0; i < students.length; i += 1) {
student = students[i];
if (response == student.name) {
listProperties(student);
response = "quit";
}
}
} while (response !== "quit");
//Outputs search results.
function listProperties(obj){
for(var name in obj) {
message += "<p>" + name + ": " + obj[name] + "</p>";
}
}
print(message);
3 Answers
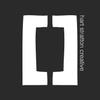
Angelica Hart Lindh
19,465 PointsHi David,
I liked your approach to implement a more dynamic and robust method of printing the results from the Array of Students. I have taken some time to refactor it and convert some of the code to use more concise ES2015 syntax I thought you would like to check it out.
const outputDiv = document.getElementById('output');
const print = message => outputDiv.innerHTML = message;
let response = '';
do {
response = prompt("Search a name of the student of type 'quit' to exit.");
//Searches students
students.forEach( student => {
if (response.toLowerCase() === student.name.toLowerCase()) {
let message = '';
Object.keys(student).forEach( item => {
message += `<p>${item}: ${student[item]}\</p>`
});
print(message);
response = 'quit';
}
});
} while (response !== 'quit');
Example on jsfiddle
Have a read through and shoot any questions you have. You can also find out about the new features of ES2015 with this course from Treehouse Introducing ES2015
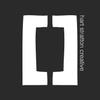
Angelica Hart Lindh
19,465 PointsNo problem, ES2015 really introduces some syntax improvements to javascript code.
The backticks/grave accents in the code represent a Template Literal and the ${expression}
is where to place an expression. e.g:
// OLD STRING CONCATENATION
var pet = 'dog';
var name = 'Archer';
var stringExample = 'I have a ' + pet + ' named ' + name + '.';
// IS THE SAME AS
const pet = 'dog';
const name = 'Archer';
const stringExample = `I have a ${pet} named ${name}.`;
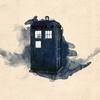
Paul Henry
2,125 PointsThis is great... I just have one question. How can this be altered to return identically named students, such as two differently Jody's?
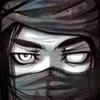
David Mendiola
Full Stack JavaScript Techdegree Student 16,579 PointsI actually have the loop break at the wrong place I should have put it outside the for loop.
var message = '',
student,
response = '';
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
do {
response = prompt("Search a name of the student of type 'quit' to exit.");
for (var i = 0; i < students.length; i++) {
student = students[i];
if (response.toLowerCase() === student.name.toString().toLowerCase()) {
listProperties(student);
}
}
if (message.length > 0){
response = "q";
}
} while (response !== "q");
function listProperties(obj){
for(var name in obj) {
message += "<p>" + name + ": " + obj[name] + "</p>";
}
}
print(message);
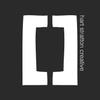
Angelica Hart Lindh
19,465 PointsHi Paul,
Good question! I personally would go about it like this:
//code above omitted
do {
// Using Array.filter() to return true or false for the search parameter against a student name
// Then use Array.map() to return a new array of filtered students
const filteredArray = students
.filter( student => response.toLowerCase() === student.name.toLowerCase() )
.map( student => student );
filteredArray.forEach( student => {
let message = '';
Object.keys(student).forEach( item => {
message += `<p>${item}: ${student[item]}\</p>`
});
print(message);
response = 'quit';
});
} while (response !== 'quit');
// Rest of the code omitted for brevity
Hope that makes sense to you. All the Javascript array methods
David Mendiola
Full Stack JavaScript Techdegree Student 16,579 PointsDavid Mendiola
Full Stack JavaScript Techdegree Student 16,579 PointsThanks for this it was really a treat to learn, especially shorthanding the print function. I was able to discern most of the new code.
This line however I understand but what does the "$" represent for the line below.
message += `<p>${item}: ${student[item]}\</p>`
Thanks again!