Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial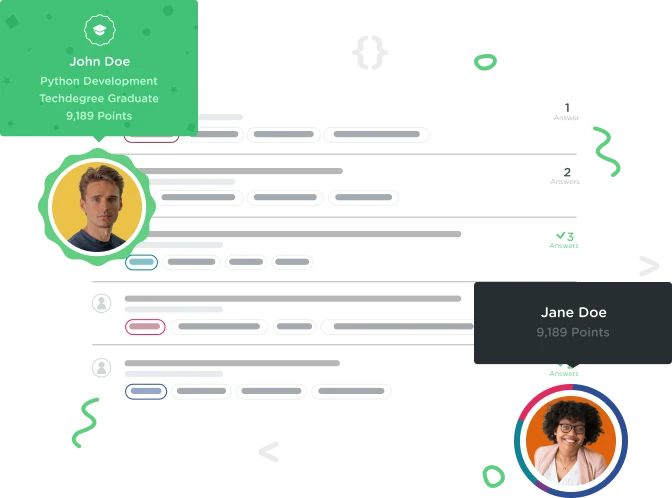
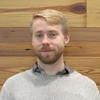
Cody Selman
7,643 PointsMy Solution to "The Build an Object Challenge Part 2."
Hello Everyone,
Here is a snapshot of my solution: https://w.trhou.se/sk86jqse0x
Here are the important JavaScript bits:
function print(message) {
document.write(message);
}
function capitalizeFirstLetter(string) {
return string.charAt(0).toUpperCase() + string.slice(1);
}
function createStudentsHtml (arr) {
for ( var i = 0; i < arr.length; i++ ) {
var currentObject = arr[i]
for ( var prop in currentObject ) {
if ( prop === 'name' ) {
html += '<h2>';
html += capitalizeFirstLetter(prop) + ': ' + currentObject[prop] + '<br>';
html += '</h2>';
} else {
html += '<p>';
html += capitalizeFirstLetter(prop) + ': ' + currentObject[prop] + '<br>';
html += '</p>';
}
}
html += '<br>';
}
}
createStudentsHtml(students);
print(html);
Let me know what you think, or if you have any suggestions or questions about my solution, please.
4 Answers
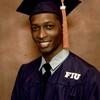
Dane Parchment
Treehouse Moderator 11,077 PointsLet me start by saying congrats on completing a challenge with your own solution, it shows that you are learning and that you are using the tools that you have picked up throughout the course.
Now as for a critique on your solution....it could be better. Now that doesn't mean that your solution is wrong, in fact it may very well work for the job at hand.
Time Complexity
As a software engineer Time Complexity is a very important concept that I need to take into consideration in my day job, and here, I am seeing bad time complexity for no discernible gain.
Basically my code smell for this is the nested loop, unless you are breaking the list/object into smaller pieces on each iteration you are running at a time complexity of O(n)
where n is the number of elements in the list. Normally this is ok, but the problem is you have another loop nested within in, which will multiply the time required to run by another factor. In this case the inner loop loops through each of the object which gives us a time complexity of O(m)
. Unfortunately because this is nested we get a total time complexity of O(n x m)
. With smaller input values this isn't bad, but in web development the student array could have 50 thousand students in it (typical college numbers for example) and students would notice a noticeable delay in there web page loading.
So in terms of time complexity you could have this reduced down to O(n)
.
Space Complexity
In terms of space complexity (how much memory your program uses over the course of its execution), I would say you are alright, it could be better, but it won't kill the RAM of your browser or computer (probably won't even make a dent.
Execution
In terms of how you wrote the program, I would say that while it is written in a very easy to understand manner there are a couple of issues here that I want to go over.
- The inner for loop. Basically this structure doesn't really need to be here. I want to to read through your code and think about what it is you are doing. Here you are going through an array of object, so the outer loop is necessary to access all of the student objects. However, what is that inner loop actually doing? You are looping through each object's properties...but do you actually need to?
- That if statement is also not really necessary. Like the inner for loop, I want you to consider whether you actually need to use it, and what you are currently using it for. Here you are using it to check if the current property you are looping through is the
name
property and if so, specify the type of html tags it gets. But again, thinking about the solution do you actually need to do that?
Fixing the issues
So I have left you with a few questions and now I will sort of answer them.
- For the inner loop, wouldn't it be easier if you just accessed the properties through dot notation instead? Like so:
object.property
. From there you could just use concatenation to add the properties' value to a string.... - The inner if statement is also not really necessary if we implement the above fix for removing the inner for loop.
Using that logic I think you can come up with a more optimized version of the solution!
If you are interested in learning more about time complexity, space complexity, and algorithms/computer science topics in general. Check Out Team Tree Houses' own video series on Algorithms.

Roger Hwang
3,851 PointsHello,
How did you create a code snippet like that on here?
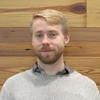
Cody Selman
7,643 PointsHello Roger,
You can style code as I have done above by placing three backticks (`) before and after the code. The initial three backticks can be followed by the name of the language for better formatting. For example, this:
'''JavaScript
var foo = 'bar';
'''
will turn into this after replacing the single-quotes with backticks:
var foo = 'bar';
Treehouse links this site for Markdown Reference, but there's also typically a Markdown Cheatsheet pop-up you can reference below any forum post input box. I hope this helps!
Best regards,

Roger Hwang
3,851 PointsThanks Cody. Did google this question and found out there too. Thanks!
Cody Selman
7,643 PointsCody Selman
7,643 PointsHello Dane,
Thank you very much for your feedback! I didn't consider how the nested loops would impact performance. I'll be sure to check out the Algorithms videos you've recommended.
Best regards,
Morgan Walstrom
4,683 PointsMorgan Walstrom
4,683 PointsWow, excellent feedback! :clap